
stm32h7 udp server with adc
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "main.h" 00003 #include "cmsis_os.h" 00004 #include "communication.h" 00005 #include "ADE7912.h" 00006 #include "stdlib.h" 00007 #include "EthernetInterface.h" 00008 #include "LWIPStack.h" 00009 #include "dma.h" 00010 #include "spi.h" 00011 #include "gpio.h" 00012 00013 EthernetInterface net; 00014 00015 DigitalOut led1(LED1); 00016 Thread thread; 00017 int out_buffer[2]; 00018 00019 uint32_t bufFR = 0xAA; 00020 uint8_t str4byte[3]; 00021 00022 00023 void UDP_thread() 00024 { 00025 printf("UDP Socket example\n"); 00026 net.set_network(SocketAddress("192.168.0.10"), SocketAddress("255.255.255.0"), SocketAddress("192.168.0.1")); 00027 if (0 != net.connect()) { 00028 printf("Error connecting\n"); 00029 } 00030 00031 UDPSocket sock; 00032 sock.open(&net); 00033 sock.bind(55151); 00034 SocketAddress receive("192.168.0.1", 55151); 00035 00036 for(;;) { 00037 // out_buffer = {rand(), rand()}; 00038 // sock.recvfrom(&receive, &out_buffer, sizeof(out_buffer)); 00039 // printf(out_buffer); 00040 if (0 > sock.sendto(receive, str4byte, sizeof(str4byte))) { 00041 printf("Error sending data\n"); 00042 } 00043 printf("Update %d, %d, %d \n", str4byte[0], str4byte[1], str4byte[2]); 00044 ThisThread::sleep_for(2000); 00045 } 00046 } 00047 00048 00049 void ADC_thread(){ 00050 struct ADE7912_Inst *ade = New_ADE7912(&hspi1); 00051 struct ADE7912_Phase_Settings a_phase; 00052 a_phase.CS_pin = PHASE_A_CS_Pin; 00053 a_phase.CS_port = PHASE_A_CS_GPIO_Port; 00054 ADE7912_PhaseInit(ade, &a_phase, PHASE_A); 00055 ADE7912_EnablePhase(ade, PHASE_A); 00056 //SCB_DisableDCache(); 00057 00058 uint32_t tickCount = osKernelSysTick(); 00059 /* Infinite loop */ 00060 for(;;) 00061 { 00062 ADE7912_UpdateData(ade); 00063 00064 bufFR = (int32_t)(ADE7912_GetCurrent(ade, PHASE_A) * 1000); 00065 sprintf( (char *)str4byte, "%03d", (short)bufFR ); 00066 00067 // TransmitUARTPackage(&huart3, str_cur+1, 12); 00068 // TransmitUARTPackage(&huart3, str4byte, 3); 00069 // TransmitUARTPackage(&huart3, str_vol+1, 12); 00070 00071 bufFR = (ADE7912_GetVoltage(ade, PHASE_A) * 1000); 00072 sprintf( (char *)str4byte, "%03d", (short)bufFR ); 00073 00074 // TransmitUARTPackage(&huart3, str4byte, 3); 00075 // TransmitUARTPackage(&huart3, str_temp+1, 9); 00076 00077 bufFR = (ADE7912_GetTemp(ade, PHASE_A) * 1); 00078 sprintf( (char *)str4byte, "%03d", (short)bufFR ); 00079 00080 // TransmitUARTPackage(&huart3, str4byte, 3); 00081 // TransmitUARTPackage(&huart3, str_space+1, 2); 00082 00083 ThisThread::sleep_for(1000); 00084 00085 } 00086 } 00087 00088 int main() 00089 { 00090 MX_GPIO_Init(); 00091 MX_DMA_Init(); 00092 MX_SPI1_Init(); 00093 00094 thread.start(UDP_thread); 00095 00096 thread.start(ADC_thread); 00097 00098 //while (true) { 00099 // led1 = !led1; 00100 // ThisThread::sleep_for(500); 00101 // } 00102 }
Generated on Tue Jul 12 2022 15:50:58 by
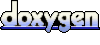