
ソースの整理中ですが、利用はできます。
Dependencies: EthernetInterface HttpServer TextLCD mbed-rpc mbed-rtos mbed Socket lwip-eth lwip-sys lwip
RPCHandler.cpp
00001 /* 00002 Permission is hereby granted, free of charge, to any person obtaining a copy 00003 of this software and associated documentation files (the "Software"), to deal 00004 in the Software without restriction, including without limitation the rights 00005 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00006 copies of the Software, and to permit persons to whom the Software is 00007 furnished to do so, subject to the following conditions: 00008 00009 The above copyright notice and this permission notice shall be included in 00010 all copies or substantial portions of the Software. 00011 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00013 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00014 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00015 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00016 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00018 THE SOFTWARE. 00019 */ 00020 00021 //#define _DEBUG_RPC_HANDLER 00022 00023 #include "RPCHandler.h" 00024 #include "mbed_rpc.h" 00025 00026 #define RPC_DATA_LEN 64 00027 00028 RPCHandler::RPCHandler(const char* rootPath, const char* path, TCPSocketConnection* pTCPSocketConnection) : HTTPRequestHandler(rootPath, path, pTCPSocketConnection) 00029 {} 00030 00031 RPCHandler::~RPCHandler() 00032 { 00033 #ifdef _DEBUG_RPC_HANDLER 00034 printf("++++(RPC Handler)Handler destroyed\r\n"); 00035 #endif 00036 } 00037 00038 void RPCHandler::doGet() 00039 { 00040 #ifdef _DEBUG_RPC_HANDLER 00041 printf("++++(RPC Handler)doGet\r\n"); 00042 #endif 00043 char resp[RPC_DATA_LEN] = {0}; 00044 char req[RPC_DATA_LEN] = {0}; 00045 00046 #ifdef _DEBUG_RPC_HANDLER 00047 printf("++++(RPC Handler)Path : %s\r\n", path().c_str()); 00048 printf("++++(RPC Handler)Root Path : %s\r\n", rootPath().c_str()); 00049 #endif 00050 //Remove path 00051 strncpy(req, path().c_str(), RPC_DATA_LEN-1); 00052 #ifdef _DEBUG_RPC_HANDLER 00053 printf("++++(RPC Handler)RPC req(before) : %s\r\n", req); 00054 #endif 00055 //Remove "%20", "+", "," from req 00056 cleanReq(req); 00057 #ifdef _DEBUG_RPC_HANDLER 00058 printf("++++(RPC Handler)RPC req(after) : %s\r\n", req); 00059 #endif 00060 //Do RPC Call 00061 RPC::call(req, resp); //FIXME: Use bool result 00062 #ifdef _DEBUG_RPC_HANDLER 00063 printf("++++(RPC Handler)Response %s \r\n",resp); 00064 #endif 00065 //Response 00066 setContentLen( strlen(resp) ); 00067 00068 //Make sure that the browser won't cache this request 00069 // respHeaders()["Cache-control"]="no-cache;no-store"; 00070 // respHeaders()["Pragma"]="no-cache"; 00071 // respHeaders()["Expires"]="0"; 00072 addRespHeaders("Cache-control","no-cache;no-store"); 00073 addRespHeaders("Pragma","no-cache"); 00074 addRespHeaders("Expires","0"); 00075 00076 //Write data 00077 // respHeaders()["Connection"] = "close"; 00078 addRespHeaders("Connection", "close"); 00079 writeData(resp, strlen(resp)); 00080 #ifdef _DEBUG_RPC_HANDLER 00081 printf("++++(RPC Handler)Exit RPCHandler::doGet()\r\n"); 00082 #endif 00083 } 00084 00085 void RPCHandler::doPost() 00086 { 00087 00088 } 00089 00090 void RPCHandler::doHead() 00091 { 00092 00093 } 00094 00095 00096 void RPCHandler::onReadable() //Data has been read 00097 { 00098 00099 } 00100 00101 void RPCHandler::onWriteable() //Data has been written & buf is free 00102 { 00103 #ifdef _DEBUG_RPC_HANDLER 00104 printf("++++(RPC Handler)onWriteable event\r\n"); 00105 #endif 00106 // close(); //Data written, we can close the connection 00107 } 00108 00109 void RPCHandler::onClose() //Connection is closing 00110 { 00111 //Nothing to do 00112 } 00113 00114 void RPCHandler::cleanReq(char* data) 00115 { 00116 char* p; 00117 if((p = strstr(data, "+"))!=NULL)memset((void*) p, ' ', 1); 00118 else if((p = strstr(data, ","))!=NULL)memset((void*) p, ' ', 1); 00119 else if((p = strstr(data, "%20"))!=NULL) { 00120 memset((void*) p, ' ', 1); 00121 while(*(p+2)!=NULL) { 00122 p++; 00123 memset((void*) p,*(p+2),1); 00124 } 00125 } 00126 00127 if((p = strstr(data, "+"))!=NULL)memset((void*) p, ' ', 1); 00128 else if((p = strstr(data, ","))!=NULL)memset((void*) p, ' ', 1); 00129 else if((p = strstr(data, "%20"))!=NULL) { 00130 memset((void*) p, ' ', 1); 00131 while(*(p+2)!=NULL) { 00132 p++; 00133 memset((void*) p,*(p+2),1); 00134 } 00135 } 00136 } 00137 00138 00139 00140
Generated on Tue Jul 12 2022 13:42:57 by
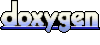