changed low freq. clock source to IRC
Dependents: BLE_ANCS_SDAPI_IRC
Fork of nRF51822 by
nRF51822n.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "nRF51822n.h" 00019 00020 #include "btle/btle.h" 00021 00022 /** 00023 * The singleton which represents the nRF51822 transport for the BLEDevice. 00024 */ 00025 static nRF51822n deviceInstance; 00026 00027 /** 00028 * BLE-API requires an implementation of the following function in order to 00029 * obtain its transport handle. 00030 */ 00031 BLEDeviceInstanceBase * 00032 createBLEDeviceInstance(void) { 00033 return (&deviceInstance); 00034 } 00035 00036 /**************************************************************************/ 00037 /*! 00038 @brief Constructor 00039 */ 00040 /**************************************************************************/ 00041 nRF51822n::nRF51822n(void) 00042 { 00043 } 00044 00045 /**************************************************************************/ 00046 /*! 00047 @brief Destructor 00048 */ 00049 /**************************************************************************/ 00050 nRF51822n::~nRF51822n(void) 00051 { 00052 } 00053 00054 /**************************************************************************/ 00055 /*! 00056 @brief Initialises anything required to start using BLE 00057 00058 @returns ble_error_t 00059 00060 @retval BLE_ERROR_NONE 00061 Everything executed properly 00062 00063 @section EXAMPLE 00064 00065 @code 00066 00067 @endcode 00068 */ 00069 /**************************************************************************/ 00070 ble_error_t nRF51822n::init(void) 00071 { 00072 /* ToDo: Clear memory contents, reset the SD, etc. */ 00073 btle_init(); 00074 00075 return BLE_ERROR_NONE; 00076 } 00077 00078 /**************************************************************************/ 00079 /*! 00080 @brief Resets the BLE HW, removing any existing services and 00081 characteristics 00082 00083 @returns ble_error_t 00084 00085 @retval BLE_ERROR_NONE 00086 Everything executed properly 00087 00088 @section EXAMPLE 00089 00090 @code 00091 00092 @endcode 00093 */ 00094 /**************************************************************************/ 00095 ble_error_t nRF51822n::reset(void) 00096 { 00097 wait(0.5); 00098 00099 /* Wait for the radio to come back up */ 00100 wait(1); 00101 00102 return BLE_ERROR_NONE; 00103 }
Generated on Tue Jul 12 2022 16:36:22 by
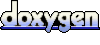