Using 5 sensors(luminance, motion, tempurature, humanity, air_quality) to control
Dependencies: Air_Quality DHT mbed-src
main.cpp
00001 #include "mbed.h" 00002 #include "DHT.h" 00003 #include"Air_Quality.h" 00004 00005 AirQuality airqualitysensor; 00006 int current_quality = -1; 00007 PinName analogPin = A0; 00008 00009 DHT sensor(D3, DHT11); 00010 InterruptIn motion(D2); 00011 AnalogIn luminance(A1); 00012 00013 DigitalOut led_R(LED_RED); 00014 DigitalOut led_G(LED_GREEN); 00015 DigitalOut led_B(LED_BLUE); 00016 00017 int motion_detected = 0; 00018 00019 // Interrupt Handler 00020 void AirQualityInterrupt() 00021 { 00022 AnalogIn sensor(analogPin); 00023 airqualitysensor.last_vol = airqualitysensor.first_vol; 00024 airqualitysensor.first_vol = sensor.read()*1000; 00025 airqualitysensor.timer_index = 1; 00026 } 00027 00028 void irq_handler(void) 00029 { 00030 motion_detected = 1; 00031 } 00032 00033 int main() 00034 { 00035 int error = 0; 00036 float hum = 0.0f; 00037 float cel = 0.0f; 00038 00039 airqualitysensor.init(analogPin, AirQualityInterrupt); 00040 00041 int cnt = 0; 00042 motion.rise(&irq_handler); 00043 00044 while(1) 00045 { 00046 wait(0.1); 00047 error = sensor.readData(); 00048 00049 if (0 == error) { 00050 hum = sensor.ReadHumidity(); 00051 cel = sensor.ReadTemperature(CELCIUS); 00052 printf(" 1. Humidity : %4.2f\r\n\n", hum); 00053 printf(" 2. Temperature in Celcius : %2.2f\r\n\n", cel); 00054 00055 { 00056 if (hum > 70) { // if celcius is higher than 28, LED_RED on. 00057 led_R = 0; // LED_RED on 00058 led_B = 1; // LED_BLUE off 00059 } 00060 else{ 00061 led_R = 1; // LED_RED off 00062 led_B = 0; // LED_BLUE on 00063 } 00064 } 00065 { 00066 if(cel > 28) { // if celcius is higher than 28, LED_RED on. 00067 led_R = 0; // LED_RED on 00068 led_B = 1; // LED_BLUE off 00069 } 00070 else { 00071 led_R = 1; // LED_RED off 00072 led_B = 0; // LED_BLUE on 00073 } 00074 } 00075 } 00076 else { 00077 printf("Error : %d\n", error); 00078 } 00079 00080 if(motion_detected) { 00081 cnt++; 00082 motion_detected = 0; 00083 /*led_R = 1; 00084 led_G = 1; 00085 led_B = 1;*/ 00086 00087 printf("3. Something move%d\r\n\n", cnt); 00088 wait(0.1); 00089 } 00090 00091 if(luminance.read()){ 00092 00093 if(0.1<=luminance.read()&&luminance.read()<=0.3){ 00094 led_R=0; led_G=0; led_B=1;} // yellow LED on 00095 00096 else{ 00097 led_R=1; led_G=1; led_B=1;} // led off 00098 00099 printf("4. Luminance: %f\r\n\n", luminance.read()); 00100 wait(0.1); 00101 } 00102 else { 00103 led_R=0; led_G=0; led_B=0; // white LED on 00104 printf("4. Luminance: %f\r\n\n", luminance.read()); 00105 wait(0.1); 00106 } 00107 //air quality sensor 00108 current_quality=airqualitysensor.slope(); 00109 if (current_quality >= 0) { // if a valid data returned. 00110 if (current_quality == 0){ 00111 printf("5. High pollution! Force signal active\n\r\n"); 00112 // led1 = 0; 00113 // led2 = 1; 00114 } 00115 else if (current_quality == 1){ 00116 printf("5. High pollution!\n\r\n"); 00117 // led1 = 0; 00118 // led2 = 1; 00119 } 00120 else if (current_quality == 2){ 00121 printf("5. Low pollution!\n\n\r"); 00122 // led1 = 0; 00123 // led2 = 1; 00124 } 00125 else if (current_quality == 3){ 00126 printf("5. Fresh air\n\r\n"); 00127 // led1 = 1; 00128 // led2 = 0; 00129 } 00130 } 00131 00132 } 00133 } 00134 /* 00135 AnalogIn luminance(A0); 00136 InterruptIn motion(D2); 00137 00138 DigitalOut RED(LED1, 1); 00139 DigitalOut GREEN(LED2, 1); 00140 DigitalOut BLUE(LED3, 1); 00141 00142 int motion_detected = 0; 00143 00144 void irq_handler(void) 00145 { 00146 motion_detected = 1; 00147 } 00148 00149 int main() 00150 { 00151 int cnt = 0; 00152 motion.rise(&irq_handler); 00153 00154 while (true) { 00155 00156 //grove motion sensor 00157 if(motion_detected) { 00158 cnt++; 00159 motion_detected = 0; 00160 RED = 0; 00161 GREEN = 1; 00162 BLUE = 1; 00163 wait(1); 00164 printf("Something move%d\r\n", cnt); 00165 } 00166 00167 //luminance sensor 00168 if(luminance.read()){ 00169 00170 if(0.1<=luminance.read()&&luminance.read()<=0.3){ 00171 RED=0; GREEN=0; BLUE=1;} // yellow LED on 00172 00173 else{ 00174 RED=1; GREEN=1; BLUE=1;} // led off 00175 00176 printf("Luminance: %f\r\n", luminance.read()); 00177 wait(0.5f); 00178 } 00179 else { 00180 RED=0; GREEN=0; BLUE=0; // white LED on 00181 printf("Luminance: %f\r\n", luminance.read()); 00182 wait(0.5f); 00183 } 00184 00185 } 00186 }*/ 00187 /* 00188 InterruptIn motion(D2); 00189 00190 DigitalOut RED(LED1, 1); 00191 DigitalOut GREEN(LED2, 1); 00192 DigitalOut BLUE(LED3, 1); 00193 00194 int motion_detected = 0; 00195 00196 void irq_handler(void) 00197 { 00198 motion_detected = 1; 00199 } 00200 00201 int main() 00202 { 00203 int cnt = 0; 00204 motion.rise(&irq_handler); 00205 00206 while (true) { 00207 00208 //grove motion sensor 00209 if(motion_detected) { 00210 cnt++; 00211 motion_detected = 0; 00212 RED = 0; 00213 GREEN = 1; 00214 BLUE = 1; 00215 wait(1); 00216 printf("Something move%d\r\n", cnt); 00217 } 00218 00219 } 00220 }*/
Generated on Thu Jul 14 2022 17:16:59 by
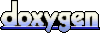