
RTnoV3 is a program which enables your device to communicate with RT-middleware world This is an example of RTno, which have double type dataport. Actually, in RTno, double is float (IEEE FLOAT32). To know RT-middleware, visit: http://www.openrtm.org To know more about RTno, visit: http://ysuga.net/robot_e/rtm_e/rtc_e/1065?lang=en
Dependencies: EthernetNetIf mbed RTnoV3
main.cpp
00001 #include "mbed.h" 00002 00003 00004 00005 /** 00006 * RTno_Template.pde 00007 * RTno is RT-middleware and arduino. 00008 * 00009 * Using RTno, arduino device can communicate any RT-components 00010 * through the RTno-proxy component which is launched in PC. 00011 * Connect arduino with USB, and program with RTno library. 00012 * You do not have to define any protocols to establish communication 00013 * between arduino and PC. 00014 * 00015 * Using RTno, you must not define the function "setup" and "loop". 00016 * Those functions are automatically defined in the RTno libarary. 00017 * You, developers, must define following functions: 00018 * int onInitialize(void); 00019 * int onActivated(void); 00020 * int onDeactivated(void); 00021 * int onExecute(void); 00022 * int onError(void); 00023 * int onReset(void); 00024 * These functions are spontaneously called by the RTno-proxy 00025 * RT-component which is launched in the PC. 00026 * @author Yuki Suga 00027 * This code is written/distributed for public-domain. 00028 */ 00029 00030 #include <RTno.h> 00031 00032 /** 00033 * This function is called at first. 00034 * conf._default.baudrate: baudrate of serial communication 00035 * exec_cxt.periodic.type: reserved but not used. 00036 */ 00037 void rtcconf(config_str& conf, exec_cxt_str& exec_cxt) { 00038 // If you want to use Serial Connection, configure below: 00039 conf._default.connection_type = ConnectionTypeSerialUSB; // USBTX & USBRX (In Windows, Driver must be updated.) 00040 //conf._default.connection_type = ConnectionTypeSerial1; // pin9=tx, pin10=rx 00041 //conf._default.connection_type = ConnectionTypeSerial2; // pin13=tx, pin14=rx 00042 //conf._default.connection_type = ConnectionTypeSerial3; // pin28=tx, pin27=rx 00043 conf._default.baudrate = 57600; // This value is required when you select ConnectionTypeSerial* 00044 00045 // If you want to use EthernetTCP, configure below: 00046 //conf._default.connection_type = ConnectionTypeEtherTcp; 00047 //conf._default.port = 23; 00048 //conf._default.mac_address = MACaddr(0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED); 00049 //conf._default.ip_address = IPaddr(192,168,42,100); 00050 //conf._default.subnet_mask = IPaddr(255,255,255,0); 00051 //conf._default.default_gateway = IPaddr(192,168,42,254); 00052 // exec_cxt.periodic.type = ProxySynchronousExecutionContext; 00053 exec_cxt.periodic.type = Timer1ExecutionContext; // onExecute is called by Timer1. Period must be specified by 'rate' option. 00054 exec_cxt.periodic.rate = 100; // [Hz] This option is indispensable when type is Timer*ExecutionContext. 00055 } 00056 00057 00058 /** 00059 * Declaration Division: 00060 * 00061 * DataPort and Data Buffer should be placed here. 00062 * 00063 * available data types are as follows: 00064 * TimedLong 00065 * TimedDouble 00066 * TimedFloat 00067 * TimedLongSeq 00068 * TimedDoubleSeq 00069 * TimedFloatSeq 00070 * 00071 * Please refer following comments. If you need to use some ports, 00072 * uncomment the line you want to declare. 00073 **/ 00074 //TimedLong in0; 00075 //InPort<TimedLong> in0In("in0", in0); 00076 //TimedLongSeq in0; 00077 //InPort<TimedLongSeq> in0In("in0", in0); 00078 00079 //TimedLong out0; 00080 //OutPort<TimedLong> out0Out("out0", out0); 00081 TimedDoubleSeq out0; 00082 OutPort<TimedDoubleSeq> out0Out("out0", out0); 00083 00084 00085 AnalogIn adcIn0(p15); 00086 AnalogIn adcIn1(p16); 00087 AnalogIn adcIn2(p17); 00088 AnalogIn adcIn3(p18); 00089 AnalogIn adcIn4(p19); 00090 AnalogIn adcIn5(p20); 00091 00092 ////////////////////////////////////////// 00093 // on_initialize 00094 // 00095 // This function is called in the initialization 00096 // sequence. The sequence is triggered by the 00097 // PC. When the RTnoRTC is launched in the PC, 00098 // then, this function is remotely called 00099 // through the USB cable. 00100 // In on_initialize, usually DataPorts are added. 00101 // 00102 ////////////////////////////////////////// 00103 int RTno::onInitialize() { 00104 /* Data Ports are added in this section. 00105 * addInPort(in1In); 00106 * 00107 * addOutPort(out1Out); 00108 */ 00109 addOutPort(out0Out); 00110 00111 // Some initialization (like port direction setting) 00112 // int LED = 13; 00113 return RTC_OK; 00114 } 00115 00116 //////////////////////////////////////////// 00117 // on_activated 00118 // This function is called when the RTnoRTC 00119 // is activated. When the activation, the RTnoRTC 00120 // sends message to call this function remotely. 00121 // If this function is failed (return value 00122 // is RTC_ERROR), RTno will enter ERROR condition. 00123 //////////////////////////////////////////// 00124 int RTno::onActivated() { 00125 // Write here initialization code. 00126 return RTC_OK; 00127 } 00128 00129 ///////////////////////////////////////////// 00130 // on_deactivated 00131 // This function is called when the RTnoRTC 00132 // is deactivated. 00133 ///////////////////////////////////////////// 00134 int RTno::onDeactivated() 00135 { 00136 // Write here finalization code. 00137 return RTC_OK; 00138 } 00139 00140 ////////////////////////////////////////////// 00141 // This function is repeatedly called when the 00142 // RTno is in the ACTIVE condition. 00143 // If this function is failed (return value is 00144 // RTC_ERROR), RTno immediately enter into the 00145 // ERROR condition.r 00146 ////////////////////////////////////////////// 00147 int RTno::onExecute() { 00148 /** 00149 * Usage of InPort with premitive type. 00150 */ 00151 /* 00152 if(in0In.isNew()) { 00153 in0In.read(); 00154 led = in0.data; 00155 } 00156 */ 00157 00158 00159 /** 00160 * Usage of InPort with sequence type 00161 */ 00162 /* 00163 if(in0In.isNew(&in1In)) { 00164 in0In.read(); 00165 for(int i = 0;i < in0.data.length;i++) { 00166 long data_buffer = in0.data[i]; 00167 } 00168 } 00169 */ 00170 00171 /** 00172 * Usage of OutPort with primitive type. 00173 out0.data = 3.14159; 00174 out0Out.write(); 00175 */ 00176 00177 /** 00178 * Usage of OutPort with sequence type. 00179 */ 00180 out0.data.length(6); 00181 out0.data[0] = (float)(adcIn0.read_u16() >> 4) * 3.0 / 1024; 00182 out0.data[1] = (float)(adcIn1.read_u16() >> 4) * 3.0 / 1024; 00183 out0.data[2] = (float)(adcIn2.read_u16() >> 4) * 3.0 / 1024; 00184 out0.data[3] = (float)(adcIn3.read_u16() >> 4) * 3.0 / 1024; 00185 out0.data[4] = (float)(adcIn4.read_u16() >> 4) * 3.0 / 1024; 00186 out0.data[5] = (float)(adcIn5.read_u16() >> 4) * 3.0 / 1024; 00187 out0Out.write(); 00188 00189 00190 return RTC_OK; 00191 } 00192 00193 00194 ////////////////////////////////////// 00195 // on_error 00196 // This function is repeatedly called when 00197 // the RTno is in the ERROR condition. 00198 // The ERROR condition can be recovered, 00199 // when the RTno is reset. 00200 /////////////////////////////////////// 00201 int RTno::onError() 00202 { 00203 return RTC_OK; 00204 } 00205 00206 //////////////////////////////////////// 00207 // This function is called when 00208 // the RTno is reset. If on_reset is 00209 // succeeded, the RTno will enter into 00210 // the INACTIVE condition. If failed 00211 // (return value is RTC_ERROR), RTno 00212 // will stay in ERROR condition.ec 00213 /////////////////////////////////////// 00214 int RTno::onReset() 00215 { 00216 return RTC_OK; 00217 }
Generated on Thu Jul 14 2022 21:04:44 by
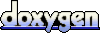