
ECE 4180 Final
Dependencies: mbed wave_player mbed-rtos C12832_lcd 4DGL-uLCD-SE LCD_fonts SDFileSystem
main.cpp
00001 #include "mbed.h" 00002 #include "SDFileSystem.h" 00003 #include "wave_player.h" 00004 #include "uLCD_4DGL.h" 00005 //setup some color objects in flash using const's 00006 00007 #include "rtos.h" 00008 #include "Small_6.h" 00009 #include "Small_7.h" 00010 #include "Arial_9.h" 00011 #include "stdio.h" 00012 #include "C12832_lcd.h" 00013 00014 //class for 3 PWM color values for RGBLED 00015 class LEDColor 00016 { 00017 public: 00018 LEDColor(float r, float g, float b); 00019 float red; 00020 float green; 00021 float blue; 00022 }; 00023 LEDColor:: LEDColor(float r, float g, float b) 00024 : red(r), green(g), blue(b) 00025 { 00026 } 00027 //Operator overload to adjust brightness with no color change 00028 LEDColor operator * (const LEDColor& x, const float& b) 00029 { 00030 return LEDColor(x.red*b,x.green*b,x.blue*b); 00031 } 00032 //Operator overload to add colors 00033 LEDColor operator + (const LEDColor& x, const LEDColor& y) 00034 { 00035 return LEDColor(x.red+y.red,x.green+y.green,x.blue+y.blue); 00036 } 00037 00038 //Class to control an RGB LED using three PWM pins 00039 class RGBLed 00040 { 00041 public: 00042 RGBLed(PinName redpin, PinName greenpin, PinName bluepin); 00043 void write(float red,float green, float blue); 00044 void write(LEDColor c); 00045 RGBLed operator = (LEDColor c) { 00046 write(c); 00047 return *this; 00048 }; 00049 private: 00050 PwmOut _redpin; 00051 PwmOut _greenpin; 00052 PwmOut _bluepin; 00053 }; 00054 00055 RGBLed::RGBLed (PinName redpin, PinName greenpin, PinName bluepin) 00056 : _redpin(redpin), _greenpin(greenpin), _bluepin(bluepin) 00057 { 00058 //50Hz PWM clock default a bit too low, go to 2000Hz (less flicker) 00059 _redpin.period(0.0005); 00060 } 00061 00062 void RGBLed::write(float red,float green, float blue) 00063 { 00064 _redpin = red; 00065 _greenpin = green; 00066 _bluepin = blue; 00067 } 00068 void RGBLed::write(LEDColor c) 00069 { 00070 _redpin = c.red; 00071 _greenpin = c.green; 00072 _bluepin = c.blue; 00073 } 00074 00075 //classes could be moved to include file 00076 00077 //Setup RGB led using PWM pins and class 00078 RGBLed myRGBled(p23,p22,p21); //RGB PWM pins 00079 const LEDColor red(1.0,0.0,0.0); 00080 const LEDColor green(0.0,0.2,0.0); 00081 //brighter green LED is scaled down to same as red and 00082 //blue LED outputs on Sparkfun RGBLED 00083 const LEDColor blue(0.0,0.0,1.0); 00084 const LEDColor yellow(1.0,0.2,0.0); 00085 const LEDColor white(1.0,0.2,1.0); 00086 const LEDColor black(0.0,0.0,0.0); 00087 char bred=0; 00088 char bgreen=0; 00089 char bblue=0; 00090 00091 volatile bool songselect = false; 00092 volatile bool homescreen = true; 00093 uLCD_4DGL uLCD(p28,p27,p30); 00094 SDFileSystem sd(p5, p6, p7, p8, "sd"); //SD card 00095 DigitalOut myled(LED1); 00096 DigitalIn pb1(p20); 00097 DigitalIn pb2(p19); 00098 AnalogOut DACout(p18); 00099 wave_player waver(&DACout); 00100 00101 // mutex to make the lcd lib thread safe 00102 Mutex lcd_mutex; 00103 int songnum = 1; 00104 AnalogIn joy_pot(p16); 00105 // Thread 1 00106 // print homescreen to LCD 00107 void thread1(void const *args) 00108 { 00109 00110 while(true) { // thread loop 00111 00112 if (homescreen){ 00113 lcd_mutex.lock(); 00114 uLCD.cls(); 00115 uLCD.text_height(1.9); 00116 uLCD.text_width(1.9); 00117 uLCD.color(WHITE); 00118 uLCD.locate(0,0); 00119 uLCD.printf("Pick a song"); 00120 uLCD.text_height(1.9); 00121 uLCD.text_width(1.9); 00122 uLCD.locate(1,2); 00123 uLCD.printf("Song1"); 00124 uLCD.locate(1,4); 00125 uLCD.printf("Song2"); 00126 uLCD.locate(1,6); 00127 uLCD.printf("Song3"); 00128 uLCD.locate(1,8); 00129 uLCD.printf("Song4"); 00130 uLCD.rectangle(5, songnum*16-2, 100, songnum*16+8 ,GREEN); 00131 lcd_mutex.unlock(); 00132 00133 } 00134 Thread::wait(200); 00135 } 00136 } 00137 00138 //for song selection during homescreen 00139 void thread2(void const *args) 00140 { 00141 00142 while(1){ 00143 if (homescreen){ 00144 if ((joy_pot <= (1.4/3.3)) && songnum>1) { 00145 songnum--; 00146 } 00147 else if ((joy_pot >= (1.8/3.3)) && songnum<4){ 00148 songnum++; 00149 } 00150 } 00151 Thread::wait(250); 00152 00153 } 00154 } 00155 00156 // Thread 3 00157 //pb1 is to select song 00158 //pb2 is to return to homescreen 00159 void thread3(void const *args) 00160 { 00161 pb1.mode(PullUp); 00162 pb2.mode(PullUp); 00163 while(true) { // thread loop 00164 if (!pb2) 00165 { 00166 homescreen = true; 00167 songselect = false; 00168 } 00169 if (!pb1) 00170 { 00171 songselect = true; 00172 } 00173 Thread::wait(100); // value of pot1 / 100 00174 } 00175 } 00176 00177 int main() 00178 { 00179 //timestamp in terms of seconds 00180 int the_middle[62][5] = {2, 0, 1, 0, 0, 00181 3, 0, 1, 0, 0, 00182 4, 0, 1, 0, 0, 00183 5, 0, 1, 0, 0, 00184 6, 0, 0, 1, 0, 00185 7, 0, 0, 1, 0, 00186 8, 0, 0, 1, 0, 00187 9, 0, 0, 1, 0, 00188 10, 0, 0, 1, 0, 00189 11, 0, 0, 0, 1, 00190 12, 0, 0, 0, 1, 00191 13, 0, 0, 0, 1, 00192 15, 0, 0, 1, 1, 00193 16, 0, 0, 1, 1, 00194 17, 0, 0, 1, 1, 00195 18, 0, 1, 1, 0, 00196 19, 0, 1, 1, 0, 00197 20, 0, 1, 1, 0, 00198 21, 0, 1, 1, 0, 00199 22, 0, 1, 1, 0, 00200 23, 0, 1, 1, 0, 00201 24, 0, 1, 1, 0, 00202 25, 0, 1, 1, 0, 00203 27, 0, 1, 0, 0, 00204 28, 0, 1, 0, 0, 00205 29, 0, 1, 0, 0, 00206 30, 0, 1, 0, 0, 00207 31, 0, 0, 1, 0, 00208 32, 0, 0, 1, 0, 00209 33, 0, 0, 1, 0, 00210 34, 0, 0, 1, 0, 00211 35, 0, 0, 1, 0, 00212 36, 0, 0, 1, 0, 00213 37, 0, 0, 0, 1, 00214 38, 0, 0, 0, 1, 00215 39, 0, 0, 0, 1, 00216 40, 0, 0, 0, 1, 00217 42, 0, 1, 1, 0, 00218 43, 0, 0, 1, 1, 00219 44, 0, 1, 1, 0, 00220 45, 0, 0, 1, 1, 00221 46, 0, 1, 1, 0, 00222 47, 0, 0, 1, 1, 00223 48, 0, 1, 1, 0, 00224 49, 0, 0, 1, 1, 00225 51, 0, 1, 1, 1, 00226 52, 0, 1, 1, 1, 00227 53, 0, 1, 1, 1, 00228 54, 0, 1, 1, 1, 00229 56, 0, 1, 0, 0, 00230 57, 0, 1, 0, 0, 00231 58, 0, 1, 0, 0, 00232 59, 0, 1, 0, 0, 00233 61, 0, 0, 1, 0, 00234 62, 0, 1, 1, 1, 00235 63, 0, 1, 1, 1, 00236 64, 0, 1, 1, 1, 00237 66, 0, 1, 1, 0, 00238 67, 0, 0, 1, 1, 00239 68, 0, 1, 1, 0, 00240 69, 0, 0, 1, 1, 00241 70, 0, 1, 1, 0}; 00242 00243 int stacys_mom[70][5] = {1, 0, 1, 0, 0, 00244 2, 0, 1, 0, 0, 00245 3, 0, 1, 1, 0, 00246 4, 0, 1, 0, 0, 00247 5, 0, 1, 0, 0, 00248 6, 0, 1, 1, 0, 00249 8, 0, 0, 1, 0, 00250 9, 0, 0, 0, 1, 00251 10, 0, 1, 0, 0, 00252 11, 0, 0, 1, 0, 00253 12, 0, 1, 1, 0, 00254 13, 0, 1, 1, 0, 00255 15, 0, 0, 1, 1, 00256 16, 0, 0, 1, 1, 00257 17, 0, 0, 1, 1, 00258 18, 0, 0, 1, 1, 00259 20, 0, 1, 0, 0, 00260 21, 0, 0, 1, 0, 00261 22, 0, 0, 0, 1, 00262 24, 0, 0, 1, 1, 00263 25, 0, 0, 1, 1, 00264 26, 0, 0, 1, 0, 00265 27, 0, 0, 1, 0, 00266 28, 0, 0, 0, 1, 00267 29, 0, 1, 0, 0, 00268 31, 0, 1, 1, 1, 00269 32, 0, 1, 0, 1, 00270 33, 0, 1, 0, 1, 00271 34, 0, 1, 0, 1, 00272 35, 0, 1, 0, 1, 00273 36, 0, 1, 0, 1, 00274 37, 0, 1, 0, 1, 00275 39, 0, 0, 1, 0, 00276 40, 0, 0, 1, 0, 00277 41, 0, 0, 1, 0, 00278 42, 0, 0, 1, 0, 00279 43, 0, 0, 0, 1, 00280 44, 0, 0, 0, 1, 00281 45, 0, 0, 1, 0, 00282 46, 0, 0, 1, 0, 00283 47, 0, 0, 1, 1, 00284 48, 0, 0, 1, 1, 00285 49, 0, 0, 1, 1, 00286 51, 0, 1, 0, 0, 00287 52, 0, 0, 1, 0, 00288 53, 0, 0, 0, 1, 00289 54, 0, 0, 1, 1, 00290 55, 0, 0, 1, 1, 00291 56, 0, 0, 1, 0, 00292 57, 0, 0, 1, 0, 00293 58, 0, 0, 1, 0, 00294 59, 0, 0, 1, 0, 00295 60, 0, 0, 1, 0, 00296 61, 0, 0, 1, 0, 00297 62, 0, 0, 1, 0, 00298 64, 0, 1, 0, 0, 00299 65, 0, 1, 0, 0, 00300 66, 0, 1, 0, 0, 00301 67, 0, 1, 0, 0, 00302 68, 0, 1, 0, 0, 00303 69, 0, 1, 0, 0, 00304 70, 0, 1, 0, 0, 00305 73, 0, 0, 1, 1, 00306 74, 0, 0, 1, 1, 00307 75, 0, 1, 0, 0, 00308 76, 0, 0, 1, 0, 00309 77, 0, 0, 0, 1, 00310 78, 0, 1, 0, 0, 00311 79, 0, 0, 1, 0, 00312 80, 0, 0, 0, 1}; 00313 00314 int sins[60][5] = {2, 0, 1, 0, 0, 00315 3, 0, 0, 1, 0, 00316 4, 0, 1, 0, 0, 00317 5, 0, 0, 1, 0, 00318 6, 0, 0, 0, 1, 00319 7, 0, 1, 0, 0, 00320 8, 0, 0, 0, 1, 00321 9, 0, 1, 0, 0, 00322 10, 0, 0, 0, 1, 00323 11, 0, 1, 1, 1, 00324 13, 0, 1, 0, 0, 00325 14, 0, 0, 1, 0, 00326 15, 0, 1, 0, 0, 00327 16, 0, 0, 1, 0, 00328 17, 0, 0, 1, 0, 00329 18, 0, 0, 1, 0, 00330 19, 0, 0, 1, 0, 00331 20, 0, 0, 1, 0, 00332 22, 0, 0, 0, 1, 00333 23, 0, 0, 1, 1, 00334 24, 0, 0, 0, 1, 00335 25, 0, 0, 1, 1, 00336 26, 0, 0, 0, 1, 00337 27, 0, 0, 1, 1, 00338 28, 0, 1, 0, 0, 00339 29, 0, 1, 1, 0, 00340 30, 0, 1, 0, 0, 00341 31, 0, 1, 1, 0, 00342 33, 0, 1, 0, 0, 00343 34, 0, 1, 0, 0, 00344 35, 0, 1, 0, 0, 00345 36, 0, 0, 1, 1, 00346 37, 0, 0, 1, 1, 00347 38, 0, 0, 1, 1, 00348 39, 0, 0, 1, 1, 00349 40, 0, 0, 1, 1, 00350 42, 0, 1, 1, 0, 00351 43, 0, 1, 1, 0, 00352 44, 0, 0, 1, 0, 00353 45, 0, 0, 1, 1, 00354 46, 0, 0, 1, 1, 00355 47, 0, 0, 1, 1, 00356 48, 0, 0, 1, 1, 00357 49, 0, 0, 1, 1, 00358 50, 0, 0, 1, 0, 00359 51, 0, 1, 1, 0, 00360 52, 0, 1, 1, 0, 00361 53, 0, 1, 1, 0, 00362 54, 0, 1, 1, 0, 00363 55, 0, 1, 1, 0, 00364 56, 0, 1, 0, 0, 00365 57, 0, 0, 1, 0, 00366 58, 0, 1, 0, 0, 00367 59, 0, 0, 1, 0, 00368 60, 0, 1, 0, 0, 00369 62, 0, 1, 1, 0, 00370 63, 0, 1, 1, 0, 00371 64, 0, 1, 1, 0, 00372 65, 0, 1, 1, 0, 00373 66, 0, 1, 1, 0}; 00374 00375 int fireflies[58][5] = { 00376 1, 0, 1, 0, 0, 00377 2, 0, 0, 1, 0, 00378 3, 0, 1, 0, 0, 00379 4, 0, 0, 1, 0, 00380 5, 0, 1, 0, 0, 00381 6, 0, 0, 1, 0, 00382 7, 0, 1, 0, 0, 00383 8, 0, 0, 1, 0, 00384 9, 0, 1, 0, 0, 00385 10, 0, 0, 1, 0, 00386 11, 0, 0, 0, 1, 00387 12, 0, 0, 1, 0, 00388 13, 0, 1, 0, 0, 00389 14, 0, 0, 1, 0, 00390 15, 0, 0, 0, 1, 00391 16, 0, 1, 1, 0, 00392 17, 0, 1, 1, 0, 00393 18, 0, 0, 1, 1, 00394 19, 0, 0, 1, 1, 00395 21, 0, 0, 1, 0, 00396 22, 0, 0, 1, 0, 00397 23, 0, 0, 1, 0, 00398 24, 0, 0, 1, 0, 00399 25, 0, 1, 0, 0, 00400 26, 0, 1, 0, 0, 00401 27, 0, 0, 1, 0, 00402 28, 0, 0, 1, 0, 00403 29, 0, 0, 0, 1, 00404 30, 0, 0, 0, 1, 00405 32, 0, 1, 0, 0, 00406 33, 0, 1, 0, 0, 00407 34, 0, 0, 1, 0, 00408 35, 0, 0, 1, 0, 00409 36, 0, 0, 0, 1, 00410 37, 0, 0, 0, 1, 00411 38, 0, 0, 1, 0, 00412 39, 0, 0, 1, 0, 00413 40, 0, 1, 0, 0, 00414 41, 0, 1, 0, 0, 00415 43, 0, 1, 1, 1, 00416 44, 0, 1, 1, 1, 00417 45, 0, 1, 1, 1, 00418 46, 0, 1, 1, 1, 00419 47, 0, 1, 1, 0, 00420 48, 0, 1, 1, 0, 00421 49, 0, 0, 1, 1, 00422 50, 0, 0, 1, 1, 00423 51, 0, 0, 1, 1, 00424 53, 0, 1, 1, 0, 00425 54, 0, 1, 1, 0, 00426 55, 0, 0, 1, 1, 00427 56, 0, 1, 1, 0, 00428 57, 0, 0, 1, 1, 00429 59, 0, 1, 0, 0, 00430 60, 0, 0, 1, 0, 00431 61, 0, 0, 0, 1, 00432 62, 0, 0, 1, 0, 00433 63, 0, 1, 0, 0}; 00434 //doesnt work yet, nee,d to ask hamblen 00435 uLCD.media_init(); 00436 uLCD.set_sector_address(0x001D, 0x4C01); 00437 uLCD.display_image(0,0); 00438 // t1.start(thread1); 00439 // t2.start(thread2); 00440 // t3.start(thread3); 00441 Thread t1(thread1); 00442 Thread t2(thread2); 00443 Thread t3(thread3); 00444 //startup sound. Commented out for testing without SD card 00445 //FILE *wave_file; 00446 //wave_file=fopen("/sd/cheer.wav","r"); 00447 //waver.play(wave_file); 00448 //fclose(wave_file); 00449 while(1) 00450 { 00451 00452 if (songselect){ 00453 myled = 0; 00454 homescreen = false; 00455 lcd_mutex.lock(); 00456 uLCD.cls(); 00457 uLCD.printf("You selected song %2d",songnum); 00458 lcd_mutex.unlock(); 00459 //add case statement based on songnum or something 00460 //code for playing song from sd 00461 //FILE *wave_file; 00462 //wave_file=fopen("/sd/sample1.wav","r"); 00463 //waver.play(wave_file); 00464 //fclose(wave_file); 00465 //end 00466 } 00467 Thread::wait(100); 00468 } 00469 } 00470 00471
Generated on Wed Aug 24 2022 18:01:02 by
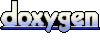