An interrupt-driven interface to 4x4 keypad.
Dependents: FYPFinalProgram FYPFinalizeProgram KEYS Proyect_Patric_electronic_door_MSC_Ok_ESP ... more
Keypad.cpp
00001 #include "Keypad.h" 00002 00003 extern Serial PC; 00004 00005 void Keypad::_cbRow0Rise() 00006 { 00007 _checkIndex(0, _rows[0]); 00008 } 00009 00010 void Keypad::_cbRow1Rise() 00011 { 00012 _checkIndex(1, _rows[1]); 00013 } 00014 00015 void Keypad::_cbRow2Rise() 00016 { 00017 _checkIndex(2, _rows[2]); 00018 } 00019 00020 void Keypad::_cbRow3Rise() 00021 { 00022 _checkIndex(3, _rows[3]); 00023 } 00024 00025 void 00026 Keypad::_setupRiseTrigger 00027 ( 00028 ) 00029 { 00030 if (_rows[0]) { 00031 _rows[0]->rise(this, &Keypad::_cbRow0Rise); 00032 } 00033 00034 if (_rows[1]) { 00035 _rows[1]->rise(this, &Keypad::_cbRow1Rise); 00036 } 00037 00038 if (_rows[2]) { 00039 _rows[2]->rise(this, &Keypad::_cbRow2Rise); 00040 } 00041 00042 if (_rows[3]) { 00043 _rows[3]->rise(this, &Keypad::_cbRow3Rise); 00044 } 00045 } 00046 00047 00048 Keypad::Keypad 00049 (PinName r0 00050 ,PinName r1 00051 ,PinName r2 00052 ,PinName r3 00053 ,PinName c0 00054 ,PinName c1 00055 ,PinName c2 00056 ,PinName c3 00057 ,int debounce_ms 00058 ) 00059 { 00060 PinName rPins[4] = {r0, r1, r2, r3}; 00061 PinName cPins[4] = {c0, c1, c2, c3}; 00062 00063 for (int i = 0; i < 4; i++) { 00064 _rows[i] = NULL; 00065 _cols[i] = NULL; 00066 } 00067 00068 _nRow = 0; 00069 for (int i = 0; i < 4; i++) { 00070 if (rPins[i] != NC) { 00071 _rows[i] = new InterruptIn(rPins[i]); 00072 _nRow++; 00073 } else 00074 break; 00075 } 00076 _setupRiseTrigger(); 00077 00078 _nCol = 0; 00079 for (int i = 0; i < 4; i++) { 00080 if (cPins[i] != NC) { 00081 _cols[i] = new DigitalOut(cPins[i]); 00082 _nCol++; 00083 } else 00084 break; 00085 } 00086 00087 _debounce = debounce_ms; 00088 } 00089 00090 Keypad::~Keypad 00091 () 00092 { 00093 for (int i = 0; i < 4; i++) { 00094 if (_rows[i] != 0) 00095 delete _rows[i]; 00096 } 00097 00098 for (int i = 0; i < 4; i++) { 00099 if (_cols[i] != 0) 00100 delete _cols[i]; 00101 } 00102 } 00103 00104 void 00105 Keypad::start 00106 ( 00107 ) 00108 { 00109 for (int i = 0; i < _nCol; i++) 00110 _cols[i]->write(1); 00111 } 00112 00113 void 00114 Keypad::stop 00115 ( 00116 ) 00117 { 00118 for (int i = 0; i < _nCol; i++) 00119 _cols[i++]->write(0); 00120 } 00121 00122 void 00123 Keypad::attach 00124 (uint32_t (*fptr)(uint32_t index) 00125 ) 00126 { 00127 _callback.attach(fptr); 00128 } 00129 00130 void 00131 Keypad::_checkIndex 00132 (int row 00133 ,InterruptIn *therow 00134 ) 00135 { 00136 #ifdef THREAD_H 00137 Thread::wait(_debounce); 00138 #else 00139 wait_ms(_debounce); 00140 #endif 00141 00142 if (therow->read() == 0) 00143 return; 00144 00145 int c; 00146 for (c = 0; c < _nCol; c++) { 00147 _cols[c]->write(0); // de-energize the column 00148 if (therow->read() == 0) { 00149 break; 00150 } 00151 } 00152 00153 int index = row * _nCol + c; 00154 _callback.call(index); 00155 start(); // Re-energize all columns 00156 } 00157
Generated on Tue Jul 12 2022 19:57:39 by
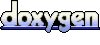