
Test Mysql
Dependencies: mbed MySQLClient EthernetNetIf
MySQLClientExample.cpp
00001 #include "mbed.h" 00002 #include "EthernetNetIf.h" 00003 #include "MySQLClient.h" 00004 00005 #define SQL_SERVER "192.168.100.204" 00006 #define SQL_USER "mysql" 00007 #define SQL_PASSWORD "mysql" 00008 #define SQL_DB "petey" 00009 00010 EthernetNetIf eth; 00011 MySQLClient sql; 00012 00013 MySQLResult sqlLastResult; 00014 void onMySQLResult(MySQLResult r) 00015 { 00016 sqlLastResult = r; 00017 } 00018 00019 int main() 00020 { 00021 printf("Start\n"); 00022 00023 printf("Setting up...\n"); 00024 EthernetErr ethErr = eth.setup(); 00025 if(ethErr) 00026 { 00027 printf("Error %d in setup.\n", ethErr); 00028 return -1; 00029 } 00030 printf("Setup OK\n"); 00031 00032 Host host(IpAddr(), 3306, SQL_SERVER); 00033 00034 //Connect 00035 sqlLastResult = sql.open(host, SQL_USER, SQL_PASSWORD, SQL_DB, onMySQLResult); 00036 while(sqlLastResult == MYSQL_PROCESSING) 00037 { 00038 Net::poll(); 00039 } 00040 if(sqlLastResult != MYSQL_OK) 00041 { 00042 printf("Error %d during connection\n", sqlLastResult); 00043 } 00044 00045 //SQL Command 00046 //Make command 00047 char cmd[128] = {0}; 00048 const char* msg="1"; 00049 const char* msg2="69.78"; 00050 const char* msg3="70.90"; 00051 const char* msg4="TRIP-A"; 00052 sprintf(cmd, "INSERT INTO location (idlocation, lati, long, tripid) VALUES('%s')", msg,msg2,msg3,msg4); 00053 00054 //INSERT INTO DB 00055 string cmdStr = string(cmd); 00056 sqlLastResult = sql.sql(cmdStr); 00057 while(sqlLastResult == MYSQL_PROCESSING) 00058 { 00059 Net::poll(); 00060 } 00061 if(sqlLastResult != MYSQL_OK) 00062 { 00063 printf("Error %d during SQL Command\n", sqlLastResult); 00064 } 00065 00066 sql.exit(); 00067 00068 while(1) 00069 { 00070 00071 } 00072 00073 return 0; 00074 }
Generated on Tue Jul 19 2022 07:30:18 by
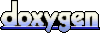