
Automatic Input USB Keyboard triggered by CapsLock.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "USBKeyboard.h" 00003 00004 //LED1: NUM_LOCK, LED2: CAPS_LOCK, LED3: SCROLL_LOCK 00005 BusOut leds(LED1, LED2, LED3); 00006 USBKeyboard keyboard; 00007 00008 int main(void) { 00009 uint8_t caps; // status of CapsLock 00010 00011 while (!keyboard.configured()) { // wait until keyboard is configured 00012 } 00013 00014 while (1) { 00015 leds = keyboard.lockStatus(); 00016 caps = keyboard.lockStatus() & 0x2; 00017 00018 // wait until CapsLock is pressed 00019 while ((keyboard.lockStatus() & 0x2) == caps) { 00020 leds = keyboard.lockStatus(); 00021 } 00022 00023 if (!caps) { 00024 keyboard.keyCode(KEY_CAPS_LOCK); // lowercase input 00025 } 00026 00027 // Automatic input 00028 keyboard.keyCode('r', 0x08); // win + r 00029 wait(0.1); 00030 keyboard.puts("iexplore http://seeedstudio.com\n\n"); 00031 } 00032 }
Generated on Mon Jul 25 2022 04:13:19 by
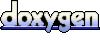