
Clock
Dependencies: DS1307 Grove_Serial_LCD mbed
main.cpp
00001 #include "mbed.h" 00002 #include "SerialLCD.h" 00003 #include "ds1307.h" 00004 00005 SerialLCD lcd(P1_13, P1_14); // Grove Serial LCD is connected to UART Tx and Rx pins 00006 DS1307 rtc(P0_5, P0_4); // Grove RTC is connected to I2C SDA(P0_5) and SCL(P0_4) 00007 00008 int main() { 00009 const char *week[] = {"Sun", "Mon", "Tue", "Wed", "Thu", "Fri", "Sat"}; 00010 int sec, min, hour, day, date, month, year; 00011 char strBuffer[16]; 00012 00013 lcd.begin(); 00014 lcd.print("Clock"); 00015 rtc.start_clock(); 00016 rtc.gettime(&sec, &min, &hour, &day, &date, &month, &year); 00017 if (0 == year) { 00018 rtc.settime(0, 0, 0, 4, 1, 1, 2014 % 100); // Jan 1st, 2014, Wed, 00:00:00 00019 } 00020 00021 while(1) { 00022 rtc.gettime(&sec, &min, &hour, &day, &date, &month, &year); 00023 snprintf(strBuffer, sizeof(strBuffer), "%d-%d-%d %s", 2000 + year, month, date, week[day]); 00024 lcd.setCursor(0, 0); 00025 lcd.print(strBuffer); 00026 snprintf(strBuffer, sizeof(strBuffer), "%d:%d:%d", hour, min, sec); 00027 lcd.setCursor(0, 1); 00028 lcd.print(strBuffer); 00029 wait(0.5); 00030 } 00031 } 00032 00033
Generated on Fri Jul 22 2022 21:22:33 by
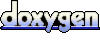