With calibration routine and driving method
Fork of Stepper_Motor_X27168 by
StepperMotor_X27168.h
00001 /** Stepper Motor control library 00002 * 00003 * @class StepperMotor_X27168 00004 * @author Aditya Garg, Nisarg Himani 00005 * @version 1.0 (Oct-19-2015) 00006 * 00007 * This is the driver for Automotive Gauge Stepper Motor X27168 00008 * 00009 * ----------------------IMPORTANT-------------------- 00010 * The API assumes motor is postioned at zero as there 00011 * is no way to detect or control that in software 00012 * --------------------------------------------------- 00013 */ 00014 00015 #ifndef MBED_STEPPER_MOTOR_X27168 00016 #define MBED_STEPPER_MOTOR_X27168 00017 00018 #include "mbed.h" 00019 00020 #define MAX_POS 629 // Maximum Steps Possible (not degrees) 00021 #define DEFAULT_SPEED 500 // Default Speed in Steps per second 00022 00023 /** Stepper Motor control class 00024 * 00025 * Example: 00026 * @code 00027 * #include "mbed.h" 00028 * #include "StepperMotor_X27168.h" 00029 * 00030 * StepperMotor_X27168 smotor(p25, p26, p23, p22); 00031 * 00032 * int main() { 00033 * 00034 * smotor.step_position(180); 00035 * wait(0.5); 00036 * 00037 * smotor.step_position(100); 00038 * wait(0.5); 00039 * 00040 * smotor.angle_position(270); 00041 * wait(0.5); 00042 * 00043 * smotor.step_position(0); 00044 * wait(0.5); 00045 * } 00046 * @endcode 00047 */ 00048 00049 class StepperMotor_X27168 { 00050 00051 public: 00052 00053 /** Constants for motor rotate control */ 00054 typedef enum { 00055 COIL_A_FOR = 0, // Forward Polarity in H-Bright Port A 00056 COIL_B_FOR = 1, // Forward Polarity in H-Bright Port B 00057 COIL_A_REV = 2, // Reverse Polarity in H-Bright Port A 00058 COIL_B_REV = 3, // Reverse Polarity in H-Bright Port B 00059 STOP_MOTOR = 4, // Turn Off Both Coils 00060 } Polarity; 00061 00062 /** Create a stepper motor object connected to specified DigitalOut pins 00063 * 00064 * @param A_f DigitalOut pin for Forward Control of H-Brigde Port A (AIN1) 00065 * @param A_r DigitalOut pin for Reverse Control of H-Brigde Port A (AIN2) 00066 * @param B_f DigitalOut pin for Forward Control of H-Brigde Port B (BIN1) 00067 * @param B_r DigitalOut pin for Reverse Control of H-Brigde Port B (BIN2) 00068 */ 00069 StepperMotor_X27168(PinName A_f, PinName A_r, PinName B_f, PinName B_r); 00070 00071 /** Create a stepper motor object connected to specified DigitalOut pins 00072 * starting at specified position 00073 * 00074 * @param A_f DigitalOut pin for Forward Control of H-Brigde Port A (AIN1) 00075 * @param A_r DigitalOut pin for Reverse Control of H-Brigde Port A (AIN2) 00076 * @param B_f DigitalOut pin for Forward Control of H-Brigde Port B (BIN1) 00077 * @param B_r DigitalOut pin for Reverse Control of H-Brigde Port B (BIN2) 00078 * @param init_step_position Rotate of given initial step position 00079 */ 00080 StepperMotor_X27168(PinName A_f, PinName A_r, PinName B_f, PinName B_r, int init_step_position); 00081 00082 /** Set the motor speed (i.e. number of steps per second) 00083 * Motor will malfuntion is speed is faster than the 00084 * the maximum capability if the motor. 00085 * 00086 * @param s steps per second : lower number makes the turn slower (default = 1000) 00087 */ 00088 void set_speed(float s); 00089 00090 /** Get the motor speed (i.e. number of steps per second) 00091 * 00092 * @return steps per second 00093 */ 00094 int get_speed(); 00095 00096 /** Set the maximum steps the motor should take (not degrees) 00097 * 00098 * @param p maximum_steps :(ignored if parameter is greater than 629, the physical limit of the motor) 00099 */ 00100 void set_max_position(int p); 00101 00102 /** Get the motor maximum position (int steps not degress) 00103 * 00104 * @return maximum position 00105 */ 00106 int get_max_position(); 00107 00108 /** Turn the motor one step (1/2 Degree) 00109 * 00110 * @param dir 0 CLOCKWISE/FORWARD 00111 * 1 ANTI-CLOCKWISE/REVERSE 00112 * 2 STOP 00113 * 00114 * @return current_position of the motor 00115 */ 00116 int step(int dir); 00117 00118 /** Turn the motor to a specific step 00119 * 00120 * @param pos desired position in steps (0-max_position) 00121 */ 00122 void step_position(int pos); 00123 00124 /** Turn the motor to a specific degree angle with a resolution of 0.5 degrees 00125 * 00126 * @param angle desired angle (0-(max_positon/2)) 00127 */ 00128 void angle_position(float angle); 00129 00130 /** Initialize the motor by rotating CW for full range and CCW for full range 00131 */ 00132 void init(); 00133 00134 private: 00135 BusOut motor_control; // 4-bit Bus Controlling the H-Brigde 00136 int max_position; // Software Limit to motor rotation 00137 int speed; // Speed of Rotation 00138 int cur_position; // Current Position of Motor (0-max_position) 00139 Polarity cur_state; // Current State of H-Brige Controls 00140 }; 00141 #endif
Generated on Thu Jul 14 2022 23:34:00 by
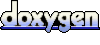