Infrared Rays library
Embed:
(wiki syntax)
Show/hide line numbers
IR.h
00001 //********************** 00002 // IR.h for mbed 00003 // 00004 // (C)Copyright 2014-2015 All rights reserved by Y.Onodera 00005 // http://einstlab.web.fc2.com 00006 //********************** 00007 00008 #ifndef IR_H_ 00009 #define IR_H_ 00010 00011 #include "mbed.h" 00012 #define IR_LIMITS 64 // bytes buffer = IR_LIMITS x8 bits 00013 #define AEHA 19 00014 #define NEC 22 00015 #define SONY 23 00016 00017 class IR{ 00018 public: 00019 IR (PinName irin, PinName irin); 00020 void init(); 00021 00022 unsigned char countHigh(); 00023 unsigned char countLow(); 00024 void getIR2(); 00025 void getIR(); 00026 void outON(char n, char t); 00027 void outOFF(char n, char t); 00028 void setIR(); 00029 00030 unsigned char buf[IR_LIMITS]; // bytes buffer 00031 unsigned char mode; // 1:NEC, 2:AEHA, 3:SONY 00032 unsigned short bits; // 32768 bits capable 00033 00034 protected: 00035 00036 DigitalIn _irin; 00037 DigitalOut _irout; 00038 00039 00040 }; 00041 00042 00043 #endif /* IR_H_ */
Generated on Fri Jul 15 2022 10:34:51 by
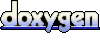