EEPROM in TG-LPC11U35-501
Dependents: mbed_EEPROM USBMSD_step1 USBMSD_step1_5 picossd_step1_2cs
EEPROM.h
00001 //********************** 00002 // EEPROM.h for TG-LPC11U35-501 00003 // 00004 // (C)Copyright 2014 All rights reserved by Y.Onodera 00005 // http://einstlab.web.fc2.com 00006 //********************** 00007 00008 #ifndef __ROMAPI_11U35_H_ 00009 #define __ROMAPI_11U35_H_ 00010 00011 #include "mbed.h" 00012 00013 typedef enum { 00014 CMD_SUCCESS = 0, // Command is executed successfully. 00015 INVALID_COMMAND = 1, // Invalid command. 00016 SRC_ADDR_ERROR = 2, // Source address is not on a word boundary. 00017 DST_ADDR_ERROR = 3, // Destination address is not on a correct boundary. 00018 SRC_ADDR_NOT_MAPPED = 4, // Source address is not mapped in the memory map. 00019 DST_ADDR_NOT_MAPPED = 5, // Destination address is not mapped in the memory map. 00020 COUNT_ERROR = 6, // Byte count is not multiple of 4 or is not a permitted value. 00021 INVALID_SECTOR = 7, // Sector number is invalid. 00022 SECTOR_NOT_BLANK = 8, // Sector is not blank. 00023 SECTOR_NOT_PREPARED_FOR_WRITE_OPERATION = 9, // Command to prepare sector for write operation was not executed. 00024 COMPARE_ERROR =10, // Source and destination data is not same. 00025 BUSY = 11 // flash programming hardware interface is busy. 00026 } IAPstatus; 00027 00028 00029 typedef enum { 00030 Prepare_sector = 50, 00031 Copy_RAM_flash = 51, 00032 Erase_sector = 52, 00033 Blank_check_sector = 53, 00034 Read_Part_ID = 54, 00035 Read_Boot_version = 55, 00036 Compare = 56, 00037 Reinvoke_ISP = 57, 00038 Read_UID = 58, 00039 Erase_page = 59, 00040 EEPROM_Write = 61, 00041 EEPROM_Read = 62 00042 } IAPcommand; 00043 00044 00045 #define IAP_LOCATION (0x1FFF1FF1UL) 00046 typedef void (*IAP)(unsigned int[], unsigned int[]); 00047 00048 00049 class EEPROM { 00050 public: 00051 EEPROM() : iap_entry( reinterpret_cast<IAP>(IAP_LOCATION) ), cclk_kHz( SystemCoreClock / 1000 ) {} 00052 00053 int write( unsigned int EEPROM_addr, char *RAM_addr, unsigned int n ); 00054 int read( unsigned int EEPROM_addr, char *RAM_addr, unsigned int n ); 00055 int put( unsigned int EEPROM_addr, char data ); 00056 char get( unsigned int EEPROM_addr ); 00057 00058 private: 00059 IAP iap_entry; 00060 unsigned int command[5]; 00061 unsigned int status[4]; 00062 char dat[1]; 00063 int cclk_kHz; 00064 }; 00065 00066 00067 #endif /* __ROMAPI_11U35_H_ */
Generated on Tue Jul 26 2022 09:04:46 by
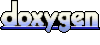