Murata RF modules are designed to simplify wireless development and certification by minimizing the amount of RF expertise you need to wirelessly enable a wide range of applications.
SoftAPConfig.cpp
00001 #include "SoftAPConfig.h" 00002 00003 using namespace SmartLabMuRata; 00004 00005 SoftAPConfig::SoftAPConfig(const State state, const char * SSID, const SecurityMode securityMode, const char * securityKey) 00006 : WIFINetwork(SSID, securityMode, securityKey) 00007 { 00008 SetOnOffState(state); 00009 } 00010 00011 SoftAPConfig::State SoftAPConfig::GetOnOffStatus() 00012 { 00013 return state; 00014 } 00015 00016 char SoftAPConfig::GetPersistency() 00017 { 00018 return persistency ? 0x01 : 0x00; 00019 } 00020 00021 SoftAPConfig * SoftAPConfig::SetOnOffState(const State onOff) 00022 { 00023 state = onOff; 00024 return this; 00025 } 00026 00027 SoftAPConfig * SoftAPConfig::SetPersistency(const bool persistency) 00028 { 00029 this->persistency = persistency; 00030 return this; 00031 } 00032 00033 SoftAPConfig * SoftAPConfig::SetSecurityKey(const char * SecurityKey) 00034 { 00035 WIFINetwork::SetSecurityKey(SecurityKey); 00036 return this; 00037 } 00038 00039 SoftAPConfig * SoftAPConfig::SetBSSID(const char * BSSID) 00040 { 00041 WIFINetwork::SetBSSID(BSSID); 00042 return this; 00043 } 00044 00045 SoftAPConfig * SoftAPConfig::SetSSID(const char * SSID) 00046 { 00047 WIFINetwork::SetSSID(SSID); 00048 return this; 00049 } 00050 00051 /// <summary> 00052 /// WIFI_SECURITY_OPEN 00053 /// WIFI_SECURITY_WPA_TKIP_PSK 00054 /// WIFI_SECURITY_WPA2_AES_PSK 00055 /// WIFI_SECURITY_WPA2_MIXED_PSK 00056 /// supported 00057 /// </summary> 00058 /// <param name="securityMode"></param> 00059 /// <returns></returns> 00060 SoftAPConfig * SoftAPConfig::SetSecurityMode(const SecurityMode securityMode) 00061 { 00062 WIFINetwork::SetSecurityMode(securityMode); 00063 return this; 00064 } 00065 00066 SoftAPConfig * SoftAPConfig::SetChannel(const char channel) 00067 { 00068 WIFINetwork::SetChannel(channel); 00069 return this; 00070 }
Generated on Fri Jul 15 2022 01:02:11 by
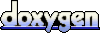