XBee and XBee-PRO ZigBee RF modules provide cost-effective wireless connectivity to electronic devices. They are interoperable with other ZigBee PRO feature set devices, including devices from other vendors.
Tx64TransmitOptions.cpp
00001 #include "Tx64TransmitOptions.h" 00002 00003 Tx64TransmitOptions::Tx64TransmitOptions() { } 00004 00005 Tx64TransmitOptions::Tx64TransmitOptions(unsigned char value) 00006 : OptionsBase(value) 00007 { } 00008 00009 Tx64TransmitOptions::Tx64TransmitOptions(bool disable_retries_and_route_repair, bool donot_repeat_packet, bool send_packet_with_broadcast_PanID, bool invoke_traceroute, bool purge_packet_if_delayed_due_to_duty_cycle) 00010 { 00011 value = 0x00; 00012 if (disable_retries_and_route_repair) 00013 value |= 0x01; 00014 if (donot_repeat_packet) 00015 value |= 0x02; 00016 if (send_packet_with_broadcast_PanID) 00017 value |= 0x04; 00018 if (invoke_traceroute) 00019 value |= 0x08; 00020 if (purge_packet_if_delayed_due_to_duty_cycle) 00021 value |= 0x10; 00022 } 00023 00024 Tx64TransmitOptions * Tx64TransmitOptions::DonotRepeatPacket = new Tx64TransmitOptions(0x02); 00025 00026 Tx64TransmitOptions * Tx64TransmitOptions::SendPacketWithBroadcastPanID = new Tx64TransmitOptions(0x04); 00027 00028 Tx64TransmitOptions * Tx64TransmitOptions::InvokeTraceroute = new Tx64TransmitOptions(0x08); 00029 00030 Tx64TransmitOptions * Tx64TransmitOptions::PurgePacketWhenDelayed = new Tx64TransmitOptions(0x10); 00031 00032 bool Tx64TransmitOptions::getDonotRepeatPacket() 00033 { 00034 if ((value & 0x02) == 0x02) 00035 return true; 00036 else return false; 00037 } 00038 00039 void Tx64TransmitOptions::setDonotRepeatPacket(bool status) 00040 { 00041 if (status) 00042 value |= 0x02; 00043 else 00044 value &= 0xFD; 00045 } 00046 00047 bool Tx64TransmitOptions::getSendPacketWithBroadcastPanID() 00048 { 00049 if ((value & 0x04) == 0x04) 00050 return true; 00051 else return false; 00052 } 00053 00054 void Tx64TransmitOptions::setSendPacketWithBroadcastPanID(bool status) 00055 { 00056 if (status) 00057 value |= 0x04; 00058 else 00059 value &= 0xFB; 00060 } 00061 00062 bool Tx64TransmitOptions::getInvokeTraceroute() 00063 { 00064 if ((value & 0x08) == 0x08) 00065 return true; 00066 else return false; 00067 } 00068 00069 void Tx64TransmitOptions::setInvokeTraceroute(bool status) 00070 { 00071 if (status) 00072 value |= 0x08; 00073 else 00074 value &= 0xF7; 00075 } 00076 00077 bool Tx64TransmitOptions::getPurgePacketWhenDelayed() 00078 { 00079 if ((value & 0x10) == 0x10) 00080 return true; 00081 else return false; 00082 } 00083 00084 void Tx64TransmitOptions::setPurgePacketWhenDelayed(bool status) 00085 { 00086 if (status) 00087 value |= 0x10; 00088 else 00089 value &= 0xEF; 00090 }
Generated on Tue Jul 12 2022 18:56:10 by
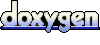