XBee and XBee-PRO ZigBee RF modules provide cost-effective wireless connectivity to electronic devices. They are interoperable with other ZigBee PRO feature set devices, including devices from other vendors.
ExplicitAddress.cpp
00001 #include "ExplicitAddress.h" 00002 00003 ExplicitAddress::ExplicitAddress() 00004 { } 00005 00006 ExplicitAddress::ExplicitAddress (const unsigned char * address, const unsigned char * explicitAddress ) 00007 : Address(address) 00008 { 00009 memcpy(explicitValue, explicitAddress, 6); 00010 } 00011 00012 ExplicitAddress::ExplicitAddress(long SerialNumberHigh, long SerialNumberLow, int NetworkAddress, int SourceEndpoint, int DestinationEndpoint, int ClusterID, int ProfileID) 00013 : Address(SerialNumberHigh, SerialNumberLow, NetworkAddress) 00014 { 00015 explicitValue[0] = SourceEndpoint; 00016 explicitValue[1] = DestinationEndpoint; 00017 explicitValue[2] = ClusterID >> 8; 00018 explicitValue[3] = ClusterID; 00019 explicitValue[4] = ProfileID >> 8; 00020 explicitValue[5] = ProfileID; 00021 } 00022 00023 unsigned char * ExplicitAddress::getExplicitValue() 00024 { 00025 return explicitValue; 00026 } 00027 00028 unsigned char ExplicitAddress::getSourceEndpoint() 00029 { 00030 return explicitValue[0]; 00031 } 00032 00033 void ExplicitAddress::setSourceEndpoint(unsigned char SourceEndpoint) 00034 { 00035 explicitValue[0] = SourceEndpoint; 00036 } 00037 00038 unsigned char ExplicitAddress::getDestinationEndpoint() 00039 { 00040 return explicitValue[1]; 00041 } 00042 00043 void ExplicitAddress::setDestinationEndpoint(unsigned char DestinationEndpoint) 00044 { 00045 explicitValue[1] = DestinationEndpoint; 00046 } 00047 00048 unsigned int ExplicitAddress::getClusterID() 00049 { 00050 return (explicitValue[2] << 8) | explicitValue[3]; 00051 } 00052 00053 void ExplicitAddress::setClusterID(unsigned int ClusterID) 00054 { 00055 explicitValue[2] = ClusterID >> 8; 00056 explicitValue[3] = ClusterID; 00057 } 00058 00059 unsigned int ExplicitAddress::getProfileID() 00060 { 00061 return (explicitValue[4] << 8) | explicitValue[5]; 00062 } 00063 00064 void ExplicitAddress::setProfileID(unsigned int ProfileID) 00065 { 00066 explicitValue[4] = ProfileID >> 8; 00067 explicitValue[5] = ProfileID; 00068 }
Generated on Tue Jul 12 2022 18:56:10 by
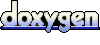