XBee and XBee-PRO ZigBee RF modules provide cost-effective wireless connectivity to electronic devices. They are interoperable with other ZigBee PRO feature set devices, including devices from other vendors.
APIFrame.h
00001 #ifndef UK_AC_HERTS_SMARTLAB_XBEE_APIFrame 00002 #define UK_AC_HERTS_SMARTLAB_XBEE_APIFrame 00003 00004 #include "BufferedArray.h" 00005 00006 /// An API frame is the structured data sent and received through the serial interface of the radio module when it is configured in API or API escaped operating modes. API frames are used to communicate with the module or with other modules in the network. 00007 class APIFrame : public BufferedArray 00008 { 00009 private: 00010 /// Checksum value for the API fram. 00011 unsigned char checkSum; 00012 00013 /// A state to indicate whether this packet's checksum is verified while process. 00014 bool isVerify; 00015 00016 public: 00017 static const unsigned char Tx64_Request =0x00; 00018 static const unsigned char Tx16_Request =0x01; 00019 static const unsigned char AT_Command = 0x08; 00020 static const unsigned char AT_Command_Queue_Parameter_Value = 0x09; 00021 static const unsigned char ZigBee_Transmit_Request = 0x10; 00022 static const unsigned char Explicit_Addressing_ZigBee_Command_Frame = 0x11; 00023 static const unsigned char Remote_Command_Request = 0x17; 00024 static const unsigned char Create_Source_Route = 0x21; 00025 static const unsigned char Register_Joining_Device = 0x24; 00026 static const unsigned char Rx64_Receive_Packet = 0x80; 00027 static const unsigned char Rx16_Receive_Packet = 0x81; 00028 static const unsigned char Rx64_IO_Data_Sample_Rx_Indicator = 0x82; 00029 static const unsigned char Rx16_IO_Data_Sample_Rx_Indicator = 0x83; 00030 static const unsigned char AT_Command_Response = 0x88; 00031 static const unsigned char XBee_Transmit_Status = 0x89; 00032 static const unsigned char Modem_Status = 0x8A; 00033 static const unsigned char ZigBee_Transmit_Status = 0x8B; 00034 static const unsigned char ZigBee_Receive_Packet = 0x90; 00035 static const unsigned char ZigBee_Explicit_Rx_Indicator = 0x91; 00036 static const unsigned char ZigBee_IO_Data_Sample_Rx_Indicator = 0x92; 00037 static const unsigned char XBee_Sensor_Read_Indicato = 0x94; 00038 static const unsigned char Node_Identification_Indicator = 0x95; 00039 static const unsigned char Remote_Command_Response = 0x97; 00040 static const unsigned char Over_the_Air_Firmware_Update_Status = 0xA0; 00041 static const unsigned char Route_Record_Indicator = 0xA1; 00042 static const unsigned char Device_Authenticated_Indicator = 0xA2; 00043 static const unsigned char Many_to_One_Route_Request_Indicator = 0xA3; 00044 00045 static const unsigned char StartDelimiter = 0x7E; 00046 00047 APIFrame(unsigned int payloadLength); 00048 00049 APIFrame(APIFrame * frame); 00050 00051 /** Get the API frame type. 00052 * 00053 * @returns 00054 * Tx64_Request =0x00, 00055 * Tx16_Request =0x01, 00056 * AT_Command = 0x08, 00057 * AT_Command_Queue_Parameter_Value = 0x09, 00058 * ZigBee_Transmit_Request = 0x10, 00059 * Explicit_Addressing_ZigBee_Command_Frame = 0x11, 00060 * Remote_Command_Request = 0x17, 00061 * Create_Source_Route = 0x21, 00062 * Register_Joining_Device = 0x24, 00063 * Rx64_Receive_Packet = 0x80, 00064 * Rx16_Receive_Packet = 0x81, 00065 * Rx64_IO_Data_Sample_Rx_Indicator = 0x82, 00066 * Rx16_IO_Data_Sample_Rx_Indicator = 0x83, 00067 * AT_Command_Response = 0x88, 00068 * XBee_Transmit_Status = 0x89, 00069 * Modem_Status = 0x8A, 00070 * ZigBee_Transmit_Status = 0x8B, 00071 * ZigBee_Receive_Packet = 0x90, 00072 * ZigBee_Explicit_Rx_Indicator = 0x91, 00073 * ZigBee_IO_Data_Sample_Rx_Indicator = 0x92, 00074 * XBee_Sensor_Read_Indicato = 0x94, 00075 * Node_Identification_Indicator = 0x95, 00076 * Remote_Command_Response = 0x97, 00077 * Over_the_Air_Firmware_Update_Status = 0xA0, 00078 * Route_Record_Indicator = 0xA1, 00079 * Device_Authenticated_Indicator = 0xA2, 00080 * Many_to_One_Route_Request_Indicator = 0xA3, 00081 */ 00082 unsigned char getFrameType(); 00083 00084 void setFrameType(unsigned char identifier); 00085 00086 void allocate(unsigned long length); 00087 00088 void rewind(); 00089 00090 bool convert(APIFrame * frame); 00091 00092 /** Write 8-bit data into current posiston and increase by 1. 00093 * @param value sigle byte 00094 */ 00095 void set(unsigned char value); 00096 00097 /** Write array of data into current posiston, and increase by the lenght. 00098 * @param value array of byte 00099 * @param offset start point of the data 00100 * @param length length to write 00101 */ 00102 void sets(const unsigned char * value, unsigned long offset, unsigned long length); 00103 00104 /** Write 8-bit data into specific posiston and deos not affect the current position. 00105 * @param position where to write 00106 * @param value sigle byte 00107 */ 00108 void set(unsigned long position, unsigned char value); 00109 00110 /** Write array of data into specific posiston and deos not affect the current position. 00111 * @param position where to write 00112 * @param value array of byte 00113 * @param offset start point of the data 00114 * @param length length to write 00115 */ 00116 void sets(unsigned long position, const unsigned char * value, unsigned long offset, unsigned long length); 00117 00118 /** Get checksum. 00119 * @returns the checksum value 00120 */ 00121 char getCheckSum(); 00122 00123 /** Set checksum. 00124 * @param value checksum value 00125 */ 00126 void setCheckSum(unsigned char value); 00127 00128 /** Check is the API frame's checksum is verified. 00129 * @returns true checksum match, 00130 * false checksum not match 00131 */ 00132 bool verifyChecksum(); 00133 00134 /// Calculate the checksum value. 00135 void calculateChecksum(); 00136 }; 00137 00138 #endif
Generated on Tue Jul 12 2022 18:56:10 by
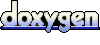