XBee and XBee-PRO ZigBee RF modules provide cost-effective wireless connectivity to electronic devices. They are interoperable with other ZigBee PRO feature set devices, including devices from other vendors.
APIFrame.cpp
00001 #include "APIFrame.h" 00002 00003 APIFrame::APIFrame(unsigned int payloadLength) 00004 :BufferedArray(payloadLength, 10) 00005 {} 00006 00007 APIFrame::APIFrame(APIFrame * frame) 00008 :BufferedArray(frame) 00009 { 00010 if (frame != NULL) { 00011 this->checkSum = frame->checkSum; 00012 this->isVerify = frame->isVerify; 00013 } 00014 } 00015 00016 unsigned char APIFrame::getFrameType() 00017 { 00018 return data[0]; 00019 } 00020 00021 void APIFrame::setFrameType(unsigned char identifier) 00022 { 00023 data[0] = identifier; 00024 } 00025 00026 void APIFrame::allocate(unsigned long length) 00027 { 00028 BufferedArray::allocate(length); 00029 isVerify = false; 00030 } 00031 00032 void APIFrame::rewind() 00033 { 00034 BufferedArray::rewind(); 00035 isVerify = false; 00036 } 00037 00038 bool APIFrame::convert(APIFrame * frame) 00039 { 00040 if (frame == NULL) 00041 return false; 00042 00043 this->data = frame->data; 00044 this->index = frame->index; 00045 this->max = frame->max; 00046 this->checkSum = frame->checkSum; 00047 this->isVerify = frame->isVerify; 00048 return true; 00049 } 00050 00051 void APIFrame::set(unsigned char value) 00052 { 00053 BufferedArray::set(value); 00054 isVerify = false; 00055 } 00056 00057 void APIFrame::sets(const unsigned char * value, unsigned long offset, unsigned long length) 00058 { 00059 BufferedArray::sets(value, offset, length); 00060 isVerify = false; 00061 } 00062 00063 void APIFrame::set(unsigned long position, unsigned char value) 00064 { 00065 BufferedArray::set(position, value); 00066 isVerify = false; 00067 } 00068 00069 void APIFrame::sets(unsigned long position, const unsigned char * value, unsigned long offset, unsigned long length) 00070 { 00071 BufferedArray::sets(position, value, offset, length); 00072 isVerify = false; 00073 } 00074 00075 char APIFrame::getCheckSum() 00076 { 00077 return checkSum; 00078 } 00079 00080 void APIFrame::setCheckSum(unsigned char value) 00081 { 00082 checkSum = value; 00083 } 00084 00085 bool APIFrame::verifyChecksum() 00086 { 00087 if (isVerify) 00088 return true; 00089 00090 char temp = 0x00; 00091 for (int i = 0; i < index; i++) 00092 temp += data[i]; 00093 if (temp + checkSum == 0xFF) 00094 isVerify = true; 00095 else 00096 isVerify = false; 00097 00098 return isVerify; 00099 } 00100 00101 void APIFrame::calculateChecksum() 00102 { 00103 if (isVerify) 00104 return; 00105 00106 char CS = 0x00; 00107 for (int i = 0; i < index; i++) 00108 CS += data[i]; 00109 checkSum = 0xFF - CS; 00110 isVerify = true; 00111 }
Generated on Tue Jul 12 2022 18:56:10 by
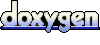