XBee API operation library for mbed
Embed:
(wiki syntax)
Show/hide line numbers
ZigBeePins.h
00001 #ifndef UK_AC_HERTS_SMARTLAB_XBEE_ZigBeePins 00002 #define UK_AC_HERTS_SMARTLAB_XBEE_ZigBeePins 00003 00004 #include "Pin.h" 00005 00006 /** 00007 * Pin configuration information for XBee S2 modules. 00008 */ 00009 class ZigBeePins 00010 { 00011 public: 00012 00013 /** VCC - - Power supply. 00014 * Poor power supply can lead to poor radio performance especially if the supply voltage is not kept within tolerance or is excessively noisy. To help reduce noise a 1uF and 8.2pF capacitor are recommended to be placed as near to pin1 on the PCB as possible. If using a switching regulator for your power supply, switching frequencies above 500 kHz are preferred. Power supply ripple should be limited to a maximum 50 mV peak to peak. 00015 */ 00016 static Pin * P1_VCC; 00017 00018 /// DOUT Output Output UART Data Out. 00019 static Pin * P2_DOUT; 00020 00021 /// DIN / CONFIG(active low) Input Input UART Data In 00022 static Pin * P3_DIN_CONFIG; 00023 00024 /// DIO12 Both Disabled Digital I/O 12. 00025 static Pin * P4_DIO12; 00026 00027 /** 00028 * RESET Both Open-Collector with pull-up 00029 * Module Reset (reset pulse must be at least 200 ns). 00030 */ 00031 static Pin * P5_RESET; 00032 00033 /// RSSI PWM / DIO10 Both Output RX Signal Strength Indicator / Digital IO. 00034 static Pin * P6_RSSI_PWM_DIO10; 00035 00036 /// DIO11 Both Input Digital I/O 11. 00037 static Pin * P7_PWM_DIO11; 00038 00039 /// [reserved] - Disabled Do not connect. 00040 static Pin * P8_RESERVED; 00041 00042 /// DTR(avtive low) / SLEEP_RQ/ DIO8 Both Input Pin Sleep Control Line or Digital IO 8. 00043 static Pin * P9_DTR_SLEEP_DIO8; 00044 00045 /// GND - - Ground. 00046 static Pin * P10_GND; 00047 00048 /// DIO4 Both Disabled Digital I/O 4. 00049 static Pin * P11_DIO4; 00050 00051 /// CTS(active low) / DIO7 Both Output Clear-to-Send Flow Control or Digital I/O 7. CTS, if enabled, is an output. 00052 static Pin * P12_CTS_DIO7; 00053 00054 /// ON / SLEEP(active low) Output Output Module Status Indicator or Digital I/O 9. 00055 static Pin * P13_ON_SLEEP; 00056 00057 /// VREF Input - Not used for EM250. Used for programmable secondary processor. For compatibility with other XBee modules, we recommend connecting this pin voltage reference if Analog sampling is desired. Otherwise, connect to GND. 00058 static Pin * P14_VREF; 00059 00060 /// Associate / DIO5 Both Output Associated Indicator, Digital I/O 5. 00061 static Pin * P15_ASSOCIATE_DIO5; 00062 00063 /// RTS(active low) / DIO6 Both Input Request-to-Send Flow Control, Digital I/O 6. RTS, if enabled, is an input. 00064 static Pin * P16_RTS_DIO6; 00065 00066 /// AD3 / DIO3 Both Disabled Analog Input 3 or Digital I/O 3. 00067 static Pin * P17_AD3_DIO3; 00068 00069 /// AD2 / DIO2 Both Disabled Analog Input 2 or Digital I/O 2. 00070 static Pin * P18_AD2_DIO2; 00071 00072 /// AD1 / DIO1 Both Disabled Analog Input 1 or Digital I/O 1. 00073 static Pin * P19_AD1_DIO1; 00074 00075 /// AD0 / DIO0 / Commissioning Button Both Disabled Analog Input 0, Digital IO 0, or Commissioning Button. 00076 static Pin * P20_AD0_DIO0_COMMISSIONONG_BUTTON; 00077 }; 00078 00079 #endif
Generated on Tue Jul 12 2022 11:17:05 by
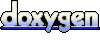