XBee API operation library for mbed
Embed:
(wiki syntax)
Show/hide line numbers
XBeePins.h
00001 #ifndef UK_AC_HERTS_SMARTLAB_XBEE_XBeePins 00002 #define UK_AC_HERTS_SMARTLAB_XBEE_XBeePins 00003 00004 #include "Pin.h" 00005 00006 /** 00007 * Pin configuration information for XBee S1 modules. 00008 */ 00009 class XBeePins 00010 { 00011 public: 00012 /** Power supply. 00013 * Poor power supply can lead to poor radio performance, especially if the supply voltage is not kept within tolerance or is excessively noisy. To help reduce noise, we recommend placing a 1.0 μF and 8.2 pF capacitor as near as possible to pin 1 on the XBee. If using a switching regulator for the power supply, switching frequencies above 500 kHz are preferred. Power supply ripple should be limited to a maximum 100 mV peak to peak. 00014 */ 00015 static Pin * P1_VCC; 00016 00017 /// DOUT, Output, UART data out. 00018 static Pin * P2_DOUT; 00019 00020 /// DIN / CONFIG(active low), Input, UART data In. 00021 static Pin * P3_DIN_CONFIG; 00022 00023 /// DO8, Either, Digital output 8, Function is not supported at the time of this release(18/05/2015). 00024 static Pin * P4_DO8; 00025 00026 /// RESET(active low), Input, Module reset (reset pulse must be at least 200 ns). 00027 static Pin * P5_RESET; 00028 00029 /// PWM0 / RSSI, Either, PWM output 0 / RX signal strength indicator. 00030 static Pin * P6_RSSI_PWM0; 00031 00032 /// PWM1, Either, PWM output 1. 00033 static Pin * P7_PWM1; 00034 00035 /// Reserved, Do not connect. 00036 static Pin * P8_RESERVED; 00037 00038 /// DTR(active low) / SLEEP_RQ/ DI8, Either, Pin sleep control line or digital input 8. 00039 static Pin * P9_DTR_SLEEP_DIO8; 00040 00041 /// Ground. 00042 static Pin * P10_GND; 00043 00044 /// AD4 / DIO4, Either, Analog input 4 or digital I/O 4. 00045 static Pin * P11_AD4_DIO4; 00046 00047 /// CTS(active low) / DIO7 Either Clear-to-send flow control or digital I/O 7. 00048 static Pin * P12_CTS_DIO7; 00049 00050 /// ON / SLEEP(active low), Output, Module status indicator 00051 static Pin * P13_ON_SLEEP; 00052 00053 /// VREF, Input, Voltage reference for A/D inputs. 00054 static Pin * P14_VREF; 00055 00056 /// Associate / AD5 / DIO5, Either, Associated indicator, analog input 5 or digital I/O 5. 00057 static Pin * P15_ASSOCIATE_AD5_DIO5; 00058 00059 /// RTS(active low) / DIO6, Either, Request-to-send flow control, or digital I/O 6. 00060 static Pin * P16_RTS_AD6_DIO6; 00061 00062 /// AD3 / DIO3 Either Analog input 3 or digital I/O 3. 00063 static Pin * P17_AD3_DIO3; 00064 00065 /// AD2 / DIO2 Either Analog input 2 or digital I/O 2. 00066 static Pin * P18_AD2_DIO2; 00067 00068 /// AD1 / DIO1 Either Analog input 1 or digital I/O 1. 00069 static Pin * P19_AD1_DIO1; 00070 00071 /// AD0 / DIO0 Either Analog input 0, digital I/O 0. 00072 static Pin * P20_AD0_DIO0; 00073 }; 00074 00075 #endif
Generated on Tue Jul 12 2022 11:17:05 by
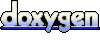