XBee API operation library for mbed
Embed:
(wiki syntax)
Show/hide line numbers
Pin.h
00001 #ifndef UK_AC_HERTS_SMARTLAB_XBEE_Pin 00002 #define UK_AC_HERTS_SMARTLAB_XBEE_Pin 00003 00004 /// Base class for XBee module pin configuration 00005 class Pin 00006 { 00007 private: 00008 static unsigned char bitfield[2]; 00009 00010 unsigned char num; 00011 00012 char com[2]; 00013 00014 unsigned char IODet[2]; 00015 00016 public: 00017 /** 00018 * Create a pin with its number, using this constructor means this pin has no IO functions. 00019 * @param number the number of the pin (0 - 20) 00020 */ 00021 Pin(unsigned char number); 00022 00023 /** 00024 * Create a pin with its number, using this constructor means this pin has IO functions. 00025 * @param number the number of the pin (0 - 20) 00026 * @param command the configure command for the pin (eg. 'D0') 00027 * @param changeDetection IO change dection bitmask configuration. 00028 */ 00029 Pin(unsigned char number, const char * command, unsigned int changeDetection); 00030 00031 // Get the pin number 00032 unsigned char getNumber(); 00033 00034 /// Get the configuration command 00035 const char * getCommand(); 00036 00037 /// Get the IO change detection bitmask for this pin 00038 const unsigned char * getIODetection(); 00039 00040 /// Get the IO change detection bitmask for a set of pins. 00041 static const unsigned char * IOChangeDetectionConfiguration(Pin ** pins, unsigned char size); 00042 00043 /// Compare two pin and check if both are the same. 00044 friend bool operator ==(const Pin &a,const Pin &b); 00045 00046 /// Compare two pin and check if both are the same. 00047 friend bool operator !=(const Pin &a,const Pin &b); 00048 }; 00049 00050 #endif
Generated on Tue Jul 12 2022 11:17:04 by
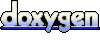