XBee API operation library for mbed
Embed:
(wiki syntax)
Show/hide line numbers
Address.h
00001 #ifndef UK_AC_HERTS_SMARTLAB_XBEE_Address 00002 #define UK_AC_HERTS_SMARTLAB_XBEE_Address 00003 00004 #include "mbed.h" 00005 00006 /// XBee address class for both S1 and S2 hardware 00007 class Address 00008 { 00009 protected: 00010 /// total 10 bytes (IEEE 64 bits + 16 bits networ address) 00011 unsigned char value[10]; 00012 public: 00013 /// Get the ZigBee broadcast address. 00014 static Address * BROADCAST_ZIGBEE; 00015 00016 /// Get the XBee broadcast address. 00017 static Address * BROADCAST_XBEE; 00018 00019 /** 00020 * Create empty address : 0x00000000 0x00000000 0x0000 00021 * this is the default ZigBee Coordinatior 00022 */ 00023 Address(); 00024 00025 /** 00026 * Create address from existing byte aray value : 8 bytes of ieee + 2 bytes network 00027 * @param address64 8 bytes of IEEE address 00028 * @param NET16 value 2 bytes network address 00029 */ 00030 Address(const unsigned char * address64, const unsigned char * NET16); 00031 00032 /** Create address from existing byte array value ( 8 bytes of IEEE address + 2 bytes 16 bit network address) 00033 * @param addr 10 bytes leong : (0-7 bytes for IEEE address, 8-9 bytes for 16 bit network address) 00034 */ 00035 Address(const unsigned char * addr); 00036 00037 /** Create address from number value. 00038 * @param serialNumberHigh first four byts of IEEE address and this can be retrieved by 'SH' command (eg. 0x0013A200) 00039 * @param serialNumberLow last four byts of IEEE address and this can be retrieved by 'SL' command. 00040 * @param networkAddress a dynamic allocated value for XBee module, 0x0000 is reserved for the coordinator, and this can be retrieved by 'MY' command. 00041 */ 00042 Address(long serialNumberHigh, long serialNumberLow, int networkAddress); 00043 00044 /// Get the first 4 bytes of IEEE address 00045 unsigned long getSerialNumberHigh(); 00046 00047 /// Get the last 4 bytes of IEEE address 00048 unsigned long getSerialNumberLow(); 00049 00050 /// Get the 16 bit network address 00051 unsigned int getNetworkAddress(); 00052 00053 /// Set the first 4 bytes of IEEE address 00054 void setSerialNumberHigh(long SerialNumberHigh); 00055 00056 /// Set the last 4 bytes of IEEE address 00057 void setSerialNumberLow(long SerialNumberLow); 00058 00059 /// Set the 16 bit network address 00060 void setNetworkAddress(int NetworkAddress); 00061 00062 /** Convert the device address to 10 bytes array. 00063 * @returns IEEE 64 bit address follow by 16 bit network address 00064 */ 00065 const unsigned char * getAddressValue(); 00066 00067 /// Compare two XBee addresses and check if both point to the same device. 00068 friend bool operator ==(const Address &a,const Address &b); 00069 00070 /// Compare two XBee addresses and check if both point to the same device. 00071 friend bool operator !=(const Address &a,const Address &b); 00072 }; 00073 00074 #endif
Generated on Tue Jul 12 2022 11:17:04 by
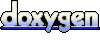