A ferroelectric random access memory or F-RAM is nonvolatile and performs reads and writes similar to a RAM.
FM24V10.h
00001 #ifndef UK_AC_HERTS_SMARTLAB_FM24V10 00002 #define UK_AC_HERTS_SMARTLAB_FM24V10 00003 00004 #include "mbed.h" 00005 00006 class FM24V10 00007 { 00008 public : 00009 /* 00010 *The speed grades (standard mode: 100 kbit/s, full speed: 400 kbit/s, fast mode: 1 mbit/s, high speed: 3,2 Mbit/s) are maximum ratings. Compliant hardware guaranties that it can handle transmission speed up to the maximum clock rate specified by the mode. 00011 */ 00012 enum SPEED_MODE { 00013 STANDARD,FULL,FAST,HIGH 00014 }; 00015 00016 FM24V10(PinName sda, PinName scl, bool A1, bool A2, SPEED_MODE speed = FULL); 00017 00018 void WriteShort(int position, int value, bool Hs_mode = false); 00019 00020 void WriteShort(int position, int * value, int size, bool Hs_mode = false); 00021 00022 void ReadShort(int position, int * value, int size, bool Hs_mode = false); 00023 00024 int ReadShort(int position, bool Hs_mode = false); 00025 00026 private : 00027 static const int HS_COMMAND = 0x09; 00028 00029 static const int FREQUENCY_STANDARD = 100000; 00030 static const int FREQUENCY_FULL = 400000; 00031 static const int FREQUENCY_FAST = 1000000; 00032 static const int FREQUENCY_HIGH = 3200000; 00033 00034 I2C _i2c_bus; 00035 int _speed; 00036 int _addr; 00037 00038 int result; 00039 00040 void Init(bool A1, bool A2); 00041 00042 void GetSlaveAddress(int position); 00043 00044 void StartHS(); 00045 00046 void StopHS(); 00047 }; 00048 00049 #endif
Generated on Fri Jul 15 2022 05:24:32 by
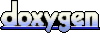