
LV8548 Motor Driver Stepper Motor Dc MOtor
Embed:
(wiki syntax)
Show/hide line numbers
Main.cpp
00001 #include "mbed.h" 00002 #include "LV8548.h" 00003 00004 #define DC_ID "LV8548DC_Ver.2.0.0\n" 00005 #define STEP_ID "LV8548Step_Ver.2.0.0\n" 00006 00007 //#define DEFAULT_BAUDRATE (9600) 00008 #define DEFAULT_BAUDRATE (19200) 00009 //#define DEFAULT_BAUDRATE (115200) 00010 00011 #define USE_DC_MOTOR (false) // true : dc motor false: Stepper 00012 00013 00014 #define _USE_DEBUG_ (1) 00015 enum DCSerialCommandType { 00016 SRMES_GET_ID = 0x03, // 3 00017 SRMES_POLLING_ID = 0x04, // 4 00018 //// For Dc Motor Control //////// 00019 SRMES_CTL_VOLTAGE = 'A', // 'A' 00020 SRMES_ROTATION = 'D', // 'D' 00021 SRMES_PWM_FREQSET = 'g', // 'g' 00022 SRMES_START_FLAG = 'h', // 'h' 00023 //// For Stepper Motor Control //////// 00024 SRMES_STEP_ANGLE = 'i', // 'i' 00025 SRMES_ROTATION_ANGLE = 'j', // 'j' 00026 SRMES_ROTATION_TIME = 'k', // 'k' 00027 SRMES_ROTATION_STEP = 'l', // 'l' 00028 SRMES_ROTATION_STOP = 'n', // 'n' 00029 SRMES_ROTATION_FREE = 'o', // 'o' 00030 }; 00031 //// For Dc Motor 00032 00033 // Segment definition of serial message 00034 typedef struct 00035 { 00036 uint8_t motorNo; 00037 uint8_t duty; 00038 } __attribute__ ((packed)) SrMesDivCtlVoltage; 00039 00040 typedef struct 00041 { 00042 uint8_t motorNo; 00043 DriverPwmOut select; 00044 } __attribute__ ((packed)) SrMesDivStartFlag; 00045 00046 typedef struct 00047 { 00048 uint8_t motorNo; 00049 DriverPwmMode select; 00050 } __attribute__ ((packed)) SrMesDivRotation; 00051 00052 typedef struct 00053 { 00054 uint8_t hz; 00055 } __attribute__ ((packed)) SrMesDivPwmFreqset; 00056 00057 00058 00059 SrMesDivCtlVoltage DcMtrvoltValue ; 00060 SrMesDivRotation DcMtrrotationValue; 00061 SrMesDivStartFlag DcMtrstartFlag; 00062 SrMesDivPwmFreqset DcMtrfreqValue; 00063 00064 /// For Stepper Motor 00065 typedef struct 00066 { 00067 uint16_t angle; 00068 } __attribute__ ((packed)) SrMesDivSetStepAngle; 00069 00070 typedef struct 00071 { 00072 uint16_t frequency; 00073 uint16_t deg; 00074 uint8_t rotation; 00075 uint8_t exp; 00076 } __attribute__ ((packed)) SrMesDivRotationDeg; 00077 00078 typedef struct 00079 { 00080 uint16_t frequency; 00081 uint16_t time; 00082 uint8_t rotation; 00083 uint8_t exp; 00084 } __attribute__ ((packed)) SrMesDivRotationTime; 00085 00086 typedef struct 00087 { 00088 uint16_t frequency; 00089 uint16_t step; 00090 uint8_t rotation; 00091 uint8_t exp; 00092 } __attribute__ ((packed)) SrMesDivRotationStep; 00093 00094 00095 00096 SrMesDivSetStepAngle StepMtrAngleValue; 00097 SrMesDivRotationDeg StepMtrDegValue; 00098 SrMesDivRotationTime StepMtrTimeValue; 00099 SrMesDivRotationStep StepMtrStepValue; 00100 00101 00102 00103 //////// USB CDC SIDE DEBUG UART ///////////// 00104 /* pc の仮想ポートをwindows softで開ける */ 00105 //Serial pc(USBTX, USBRX); // tx, rx 00106 //#if _USE_DEBUG_ 00107 //Serial uart(D1, D0); // tx, rx 00108 //#endif 00109 //////// OUT SIDE UART INPUT ///////////// 00110 #if _USE_DEBUG_ 00111 Serial uart(USBTX, USBRX); // tx, rx 00112 #endif 00113 Serial pc(D1, D0); // tx, rx 00114 00115 DigitalOut myled(LED1); 00116 #if USE_DC_MOTOR 00117 LV8548 MOTORDriver( D3,D5,D10,D11,DCMOTOR); // SELECT DCMOTOR 00118 #else 00119 LV8548 MOTORDriver( D3,D5,D10,D11,STEPERMOTOR); // STEP MOTOR 00120 #endif 00121 00122 DigitalOut TEST(D12); 00123 Ticker Timer500; 00124 Ticker Timer1ms; 00125 uint8_t LedImage; 00126 uint8_t CommandTimer; 00127 00128 #define SBUFMAX 64 00129 volatile uint8_t SerialBuffer[SBUFMAX]; 00130 volatile uint8_t RingBuffer[256]; 00131 volatile uint8_t RingReadpoint; 00132 volatile uint8_t RingWritepoint; 00133 00134 /*******************************************/ 00135 /* AA */ 00136 /* AAAA ii ii */ 00137 /* AA AA sssss cccc */ 00138 /* AAAAAA ss cc iii iii */ 00139 /* AA AA ssss cc ii ii */ 00140 /* AA AA ss cc ii ii */ 00141 /* AA AA sssss cccc iiii iiii */ 00142 /* */ 00143 /*******************************************/ 00144 00145 uint8_t Ascii(uint8_t Data) 00146 { 00147 switch(Data & 0xf) 00148 { 00149 case 0: return('0'); 00150 case 1: return('1'); 00151 case 2: return('2'); 00152 case 3: return('3'); 00153 case 4: return('4'); 00154 case 5: return('5'); 00155 case 6: return('6'); 00156 case 7: return('7'); 00157 case 8: return('8'); 00158 case 9: return('9'); 00159 case 0xA: return('A'); 00160 case 0xB: return('B'); 00161 case 0xC: return('C'); 00162 case 0xD: return('D'); 00163 case 0xE: return('E'); 00164 case 0xF: return('F'); 00165 } 00166 return (0); 00167 } 00168 /***************************************************************************************************************************/ 00169 /* UU UU RRRRR IIII */ 00170 /* UU UU tt RR RR ii II */ 00171 /* UU UU aaaa rrrrr tttttt RR RR eeee cccc vv vv eeee II rrrrr qqqqq */ 00172 /* UU UU aa rr rr tt RRRRR ee ee cc iii vv vv ee ee II rr rr qq qq */ 00173 /* UU UU aaaaa rr tt RRRR eeeeee cc ii vv vv eeeeee II rr qq qq */ 00174 /* UU UU aa aa rr tt RR RR ee cc ii vvvv ee II rr qqqqq */ 00175 /* UUUU aaaaa rr ttt RR RR eeee cccc iiii vv eeee IIII rr qq */ 00176 /* qq */ 00177 /***************************************************************************************************************************/ 00178 void Read_Rx(void) 00179 { 00180 RingWritepoint++; 00181 RingBuffer[RingWritepoint] = pc.getc(); 00182 CommandTimer = 2; // 2(最小1)ms後にコマンド処理 00183 } 00184 /***********************************************************************************************************/ 00185 /* CCCC PPPPP */ 00186 /* CC CC dd PP PP */ 00187 /* CC oooo mm mm mm mm nnnnn aaaa dd PP PP aaaa rrrrr sssss eeee */ 00188 /* CC oo oo mmmmmmm mmmmmmm nn nn aa ddddd PPPPP aa rr rr ss ee ee */ 00189 /* CC oo oo mmmmmmm mmmmmmm nn nn aaaaa dd dd PP aaaaa rr ssss eeeeee */ 00190 /* CC CC oo oo mm m mm mm m mm nn nn aa aa dd dd PP aa aa rr ss ee */ 00191 /* CCCC oooo mm mm mm mm nn nn aaaaa ddddd PP aaaaa rr sssss eeee */ 00192 /* */ 00193 /***********************************************************************************************************/ 00194 void SerialRead(void) 00195 { 00196 int i = 0; 00197 if(CommandTimer ) return; /* 1ms 以上データ無しが安定してから読む */ 00198 00199 00200 SerialBuffer[0] = '\0'; 00201 while( RingWritepoint != RingReadpoint) 00202 { 00203 RingReadpoint++; 00204 SerialBuffer[i] = RingBuffer[RingReadpoint]; 00205 #if _USE_DEBUG_ 00206 uart.putc(Ascii(SerialBuffer[i] >> 4)) ; 00207 uart.putc(Ascii(SerialBuffer[i] >> 0)) ; 00208 uart.putc(0x20); 00209 #endif 00210 i++; 00211 if(i >= (SBUFMAX-1)) 00212 { 00213 #if _USE_DEBUG_ 00214 uart.printf("Max over\n"); 00215 #endif 00216 SerialBuffer[i] = '\0'; 00217 00218 } 00219 continue; 00220 } 00221 00222 switch( SerialBuffer[0] ) 00223 { 00224 case SRMES_GET_ID: // 3 00225 { 00226 #if _USE_DEBUG_ 00227 uart.printf("Send Id\n"); 00228 #endif 00229 #if USE_DC_MOTOR 00230 pc.printf(DC_ID); 00231 #else 00232 pc.printf(STEP_ID); 00233 #endif 00234 } 00235 break; 00236 00237 case SRMES_POLLING_ID: // 4 00238 { 00239 #if _USE_DEBUG_ 00240 uart.printf("Polling\n"); 00241 #endif 00242 } 00243 break; 00244 /***************************************************************************************************/ 00245 /* FFFFFF DDDD MM MM */ 00246 /* FF DD DD MMM MMM tt */ 00247 /* FF oooo rrrrr DD DD cccc MMMMMMM oooo tttttt oooo rrrrr */ 00248 /* FFFF oo oo rr rr DD DD cc MM M MM oo oo tt oo oo rr rr */ 00249 /* FF oo oo rr DD DD cc MM MM oo oo tt oo oo rr */ 00250 /* FF oo oo rr DD DD cc MM MM oo oo tt oo oo rr */ 00251 /* FF oooo rr DDDD cccc MM MM oooo ttt oooo rr */ 00252 /* */ 00253 /***************************************************************************************************/ 00254 case SRMES_CTL_VOLTAGE: // 0x41 00255 { 00256 DcMtrvoltValue = *(SrMesDivCtlVoltage *)&SerialBuffer[1]; 00257 #if _USE_DEBUG_ 00258 uart.printf("Voltage Commnad %2x %2x \n",DcMtrvoltValue.motorNo,DcMtrvoltValue.duty); 00259 #endif 00260 MOTORDriver.SetDcPwmDuty(DcMtrvoltValue.motorNo,(float)DcMtrvoltValue.duty/(float)100); 00261 } 00262 break; 00263 00264 case SRMES_ROTATION: // 0x44 00265 { 00266 DcMtrrotationValue = *(SrMesDivRotation *)&SerialBuffer[1]; 00267 #if _USE_DEBUG_ 00268 uart.printf("Roteationcommand %2x %2x \n",DcMtrrotationValue.motorNo,DcMtrrotationValue.select); 00269 #endif 00270 MOTORDriver.SetDcPwmMode(DcMtrrotationValue.motorNo,DcMtrrotationValue.select); 00271 } 00272 break; 00273 00274 case SRMES_PWM_FREQSET: // 0x67 00275 { 00276 DcMtrfreqValue = *(SrMesDivPwmFreqset *)&SerialBuffer[1]; 00277 #if _USE_DEBUG_ 00278 uart.printf("Freq Hz %2x \n",DcMtrfreqValue.hz); 00279 #endif 00280 switch(DcMtrfreqValue.hz) 00281 { 00282 case 0: MOTORDriver.SetDcPwmFreqency( MOTOR_A, 7813 ); 00283 MOTORDriver.SetDcPwmFreqency( MOTOR_B, 7813 ); 00284 break; 00285 case 1: MOTORDriver.SetDcPwmFreqency( MOTOR_A,977 ); 00286 MOTORDriver.SetDcPwmFreqency( MOTOR_B,977 ); 00287 break; 00288 case 2: MOTORDriver.SetDcPwmFreqency( MOTOR_A,244 ); 00289 MOTORDriver.SetDcPwmFreqency( MOTOR_B,244 ); 00290 break; 00291 case 3: MOTORDriver.SetDcPwmFreqency( MOTOR_A,61 ); 00292 MOTORDriver.SetDcPwmFreqency( MOTOR_B,61 ); 00293 break; 00294 default: break; 00295 } 00296 } 00297 break; 00298 00299 case SRMES_START_FLAG: // 0x68 00300 { 00301 DcMtrstartFlag = *(SrMesDivStartFlag *)&SerialBuffer[1]; 00302 #if _USE_DEBUG_ 00303 uart.printf("StartFlag command %2x %2x \n",DcMtrstartFlag.motorNo,DcMtrstartFlag.select); 00304 #endif 00305 MOTORDriver.SetDcOutPut(DcMtrstartFlag.motorNo,DcMtrstartFlag.select); 00306 } 00307 break; 00308 /*******************************************************************************************************************/ 00309 /* FFFFFF SSSS MM MM */ 00310 /* FF SS SS tt MMM MMM tt */ 00311 /* FF oooo rrrrr SS tttttt eeee ppppp MMMMMMM oooo tttttt oooo rrrrr */ 00312 /* FFFF oo oo rr rr SSSS tt ee ee pp pp MM M MM oo oo tt oo oo rr rr */ 00313 /* FF oo oo rr SS tt eeeeee pp pp MM MM oo oo tt oo oo rr */ 00314 /* FF oo oo rr SS SS tt ee ppppp MM MM oo oo tt oo oo rr */ 00315 /* FF oooo rr SSSS ttt eeee pp MM MM oooo ttt oooo rr */ 00316 /* pp */ 00317 /*******************************************************************************************************************/ 00318 case SRMES_STEP_ANGLE: 00319 { 00320 StepMtrAngleValue = *(SrMesDivSetStepAngle *)&SerialBuffer[1]; 00321 #if _USE_DEBUG_ 00322 uart.printf("Step Angle command %4x Req:% \n",StepMtrAngleValue.angle); 00323 #endif 00324 MOTORDriver.SetStepAngle(StepMtrAngleValue.angle/100); 00325 } 00326 break; 00327 00328 case SRMES_ROTATION_ANGLE: 00329 { 00330 SrMesDivRotationDeg StepMtrDegValue = *(SrMesDivRotationDeg *)&SerialBuffer[1]; 00331 #if _USE_DEBUG_ 00332 uart.printf("Step Deg command \n"); 00333 #endif 00334 MOTORDriver.SetStepDeg(StepMtrDegValue.frequency/10, StepMtrDegValue.deg/100, StepMtrDegValue.rotation, StepMtrDegValue.exp); 00335 } 00336 break; 00337 00338 case SRMES_ROTATION_TIME: 00339 { 00340 StepMtrTimeValue = *(SrMesDivRotationTime *)&SerialBuffer[1]; 00341 #if _USE_DEBUG_ 00342 uart.printf("Step Time command \n"); 00343 #endif 00344 MOTORDriver.SetStepTime(StepMtrTimeValue.frequency/10, StepMtrTimeValue.time/10, StepMtrTimeValue.rotation, StepMtrTimeValue.exp); 00345 } 00346 break; 00347 00348 case SRMES_ROTATION_STEP: 00349 { 00350 #if _USE_DEBUG_ 00351 uart.printf("Step Step command \n"); 00352 #endif 00353 StepMtrStepValue = *(SrMesDivRotationStep *)&SerialBuffer[1]; 00354 MOTORDriver.SetStepStep(StepMtrStepValue.frequency/10, StepMtrStepValue.step/10, StepMtrStepValue.rotation,StepMtrStepValue.exp); 00355 } 00356 break; 00357 00358 case SRMES_ROTATION_STOP: 00359 { 00360 #if _USE_DEBUG_ 00361 uart.printf("Step Stop command \n"); 00362 #endif 00363 MOTORDriver.SetStepStop(); 00364 } 00365 break; 00366 00367 case SRMES_ROTATION_FREE: 00368 { 00369 #if _USE_DEBUG_ 00370 uart.printf("Step Free command \n"); 00371 #endif 00372 00373 MOTORDriver.SetStepFree(); 00374 } 00375 break; 00376 00377 default: 00378 { 00379 #if _USE_DEBUG_ 00380 // uart.printf("unknown command\n"); 00381 #endif 00382 } 00383 break; 00384 } 00385 } 00386 /***************************************************************************/ 00387 /* TTTTTT PPPPP */ 00388 /* TT ii PP PP */ 00389 /* TT mm mm eeee rrrrr PP PP rrrrr oooo cccc */ 00390 /* TT iii mmmmmmm ee ee rr rr PPPPP rr rr oo oo cc */ 00391 /* TT ii mmmmmmm eeeeee rr PP rr oo oo cc */ 00392 /* TT ii mm m mm ee rr PP rr oo oo cc */ 00393 /* TT iiii mm mm eeee rr PP rr oooo cccc */ 00394 /* */ 00395 /***************************************************************************/ 00396 void Eventtimer500(void) 00397 { 00398 LedImage = !LedImage; 00399 } 00400 00401 void Eventtimer1ms(void) 00402 { 00403 if(CommandTimer) CommandTimer --; 00404 } 00405 00406 /***********************************/ 00407 /* MM MM */ 00408 /* MMM MMM ii */ 00409 /* MMMMMMM aaaa nnnnn */ 00410 /* MM M MM aa iii nn nn */ 00411 /* MM MM aaaaa ii nn nn */ 00412 /* MM MM aa aa ii nn nn */ 00413 /* MM MM aaaaa iiii nn nn */ 00414 /* */ 00415 /***********************************/ 00416 int main() 00417 { 00418 RingReadpoint = 0; 00419 RingWritepoint = 0; 00420 CommandTimer = 0; 00421 pc .baud(DEFAULT_BAUDRATE); 00422 #if _USE_DEBUG_ 00423 uart.baud(115200);//DEFAULT_BAUDRATE); 00424 pc.printf("Program Start!\n"); 00425 uart.printf("Program deb Start!\n"); 00426 #endif 00427 Timer500.attach(&Eventtimer500, 0.5); 00428 Timer1ms.attach(&Eventtimer1ms, 0.001); 00429 pc.attach(Read_Rx, Serial::RxIrq); 00430 while(1) { 00431 SerialRead(); 00432 myled = LedImage; // LED is ON 00433 } 00434 00435 }
Generated on Sat Jul 16 2022 21:49:24 by
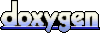