Station API
Embed:
(wiki syntax)
Show/hide line numbers
Utils.h
00001 /* 00002 Copyright (c) 2011, Senio Networks, Inc. 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #ifndef UTILS_H 00024 #define UTILS_H 00025 00026 #include "mbed.h" 00027 #include <ctype.h> 00028 #include <stdarg.h> 00029 00030 /** 00031 * Utility functions 00032 */ 00033 class Utils { 00034 public: 00035 /** 00036 * encodes string in percent encoding 00037 * 00038 * @param s source string 00039 * @param t encoded string 00040 */ 00041 static int encodeFormUrl(char *s, char *t) { 00042 char *head = t; 00043 for (char c; (c = *s) != 0; s++) 00044 switch (c) { 00045 case '\r': 00046 break; 00047 case ' ' : 00048 *t++ = '+'; 00049 break; 00050 default: 00051 t += sprintf(t, isalnum(c) ? "%c" : (c == '\n') ? "\r%c" : "%%%02X", c); 00052 } 00053 *t = '\0'; 00054 return t - head; 00055 } 00056 00057 /** 00058 * encodes data to BASE64 string 00059 * 00060 * @param ibuf input buffer 00061 * @param length length of the input data 00062 * @param obuf output buffer 00063 */ 00064 static void encodeBase64(char ibuf[], int length, char *obuf) { 00065 const char BASE64[] = 00066 "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/"; 00067 int i, j; 00068 for (i = j = 0; j < length; j += 3, i += 4) { 00069 long a = ibuf[j] << 16 | 00070 (j + 1 < length ? ibuf[j + 1] << 8 : 0) | 00071 (j + 2 < length ? ibuf[j + 2] : 0); 00072 for (int k = 3; k >= 0; k--, a >>= 6) 00073 obuf[i + k] = (j + k - 1) < length ? BASE64[a & 63] : '='; 00074 } 00075 obuf[i] = '\0'; 00076 } 00077 00078 /** 00079 * encodes string to BASE64 string 00080 * @param ibuf input string buffer 00081 * @param obuf output buffer 00082 */ 00083 static void encodeBase64(char *ibuf, char *obuf) { 00084 encodeBase64(ibuf, strlen(ibuf), obuf); 00085 } 00086 00087 /** 00088 * gets values from a file according to the specified pattern format 00089 * 00090 * @param fp pointer to the input FILE 00091 * @param pattern scanf format string, followed by variables to store the retrieved data 00092 */ 00093 static bool fgetValues(FILE *fp, const char *pattern, ...) { 00094 va_list argv; 00095 va_start(argv, pattern); 00096 00097 rewind(fp); 00098 while (!feof(fp)) { 00099 char buf[128]; 00100 fgets(buf, sizeof(buf) - 1, fp); 00101 int ret = vsscanf(buf, pattern, argv); 00102 if (ret > 0) { 00103 return true; 00104 } 00105 } 00106 return false; 00107 } 00108 }; 00109 #endif
Generated on Wed Jul 20 2022 21:57:16 by
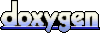