Station API
Embed:
(wiki syntax)
Show/hide line numbers
SuperTweetClient.h
00001 /* 00002 Copyright (c) 2011, Senio Networks, Inc. 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #ifndef SUPERTWEET_H 00024 #define SUPERTWEET_H 00025 00026 #include "SimpleSocket.h" 00027 #include "Utils.h" 00028 00029 /** 00030 * Client API for accessing SuperTweet gateway 00031 */ 00032 class SuperTweetClient { 00033 public: 00034 /** 00035 * creates a SuperTweetClient object 00036 * 00037 * @param user user account 00038 * @param password password (in plain text) 00039 * @param messageTemplate message template for the tweet message 00040 * @param verbose if true display debug info 00041 */ 00042 SuperTweetClient(char *user, char *password, char *messageTemplate, bool verbose = false) 00043 : verbose(verbose) { 00044 char buf[64]; 00045 sprintf(buf, "%s:%s", user, password); 00046 Utils::encodeBase64(buf, credential); 00047 strcpy(this->messageTemplate, messageTemplate); 00048 } 00049 00050 /** 00051 * creates a SuperTweetCient object from a config file 00052 * 00053 * @param filename name of the configuration file 00054 * @param verbose if true display debug info 00055 */ 00056 static SuperTweetClient create(char *filename, bool verbose = false) { 00057 char user[16], password[32], messageTemplate[420]; 00058 00059 if (filename) { 00060 char path[32]; 00061 LocalFileSystem local("local"); 00062 sprintf(path, "/local/%s", filename); 00063 if (FILE *fp = fopen(path, "r")) { 00064 Utils::fgetValues(fp, "user:%s", user); 00065 Utils::fgetValues(fp, "password:%s", password); 00066 Utils::fgetValues(fp, "message:%[^\r\n]", messageTemplate); 00067 fclose(fp); 00068 00069 if (verbose) { 00070 printf("user = %s\n", user); 00071 printf("password = %s\n", password); 00072 printf("messageTemplate = %s\n", messageTemplate); 00073 } 00074 } 00075 } 00076 00077 return SuperTweetClient(user, password, messageTemplate, verbose); 00078 } 00079 00080 /** 00081 * tweets message 00082 * 00083 * @param format template format string, if empty string specified, messageTemplate specified in the constructor is to be used 00084 * @param verbose if true display debug info 00085 */ 00086 bool tweet(char *format, ...) { 00087 va_list argv; 00088 va_start(argv, format); 00089 00090 bool result = false; 00091 00092 if (format == 0 || format[0] == 0) 00093 format = &messageTemplate[0]; 00094 00095 ClientSocket client("api.supertweet.net", 80); 00096 if (client) { 00097 char message[420], message2[420 * 3]; 00098 vsprintf(message, format, argv); 00099 int length = Utils::encodeFormUrl(message, message2); 00100 00101 const char *request = "POST /1/statuses/update.xml HTTP/1.1\r\n" 00102 "Host: api.supertweet.net\r\n" 00103 "Authorization: Basic %s\r\n" 00104 "Content-Length: %d\r\n" 00105 "Content-Type: application/x-www-form-urlencoded\r\n" 00106 "\r\n" 00107 "status="; 00108 client.printf(request, credential, length + 7); 00109 client.write(message2, length); 00110 00111 if (verbose) { 00112 printf(request, credential, length + 7); 00113 printf("%s", message2); 00114 } 00115 00116 while (client) { 00117 if (client.available()) { 00118 while (client.available()) { 00119 char response[128] = {}; 00120 client.read(response, sizeof(response) - 1); 00121 if (!result && strncmp(response, "HTTP/1.1 200", 12) == 0) 00122 result = true; 00123 if (verbose) 00124 printf("%s", response); 00125 } 00126 client.close(); 00127 } 00128 } 00129 } 00130 return result; 00131 } 00132 00133 private: 00134 bool verbose; 00135 char credential[64]; 00136 char messageTemplate[420]; 00137 }; 00138 00139 #endif
Generated on Wed Jul 20 2022 21:57:16 by
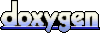