Station API
Embed:
(wiki syntax)
Show/hide line numbers
Station.h
00001 /* 00002 Copyright (c) 2011, Senio Networks, Inc. 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #ifndef STATION_H 00024 #define STATION_H 00025 00026 #include "SimpleSocket.h" 00027 #include <map> 00028 #include <string> 00029 00030 /** 00031 * A simple command/response network interface for mbed 00032 */ 00033 class Station { 00034 public: 00035 /** 00036 * creates a Station object 00037 * 00038 * @param port port number 00039 */ 00040 Station(int port) : server(port) {} 00041 00042 ~Station() {} 00043 00044 /** 00045 * adds a command handler 00046 * 00047 * @param command name of the command 00048 * @param handler pointer to the handler of the command 00049 */ 00050 void addHandler(string command, void (*handler)(void)) { 00051 handlerMap.insert(pair<string, FunctionPointer>(command, FunctionPointer(handler))); 00052 } 00053 00054 /** 00055 * adds a command handler 00056 * 00057 * @param command name of the command 00058 * @param tptr pointer to the object to call the handler on 00059 * @param handler pointer to the handler of the command 00060 */ 00061 template <typename T> void addHandler(string command, T* tptr, void (T::*handler)(void)) { 00062 handlerMap.insert(pair<string, FunctionPointer>(command, FunctionPointer(tptr, handler))); 00063 } 00064 00065 /** 00066 * sets the buffer for communication between caller and callee 00067 * 00068 * @param buf pointer to the communication buffer 00069 * @param length length of the buffer 00070 */ 00071 void setBuffer(char *buf, int length) { 00072 this->buf = buf; 00073 this->length = length; 00074 } 00075 00076 /** 00077 * Check for any client and handle its request. This function is expected to be called repeatedly in main loop. 00078 */ 00079 void handleClient() { 00080 ClientSocket client = server.accept(0); 00081 while (client) { 00082 if (client.available()) { 00083 memset(buf, '\0', length); 00084 int len = client.read(buf, this->length - 1); 00085 char name[16] = {}; 00086 sscanf(buf, "%s", name); 00087 callHandler(string(name)); 00088 if (buf[0]) { 00089 client.write(buf, strlen(buf)); 00090 } 00091 client.close(); 00092 } 00093 } 00094 } 00095 00096 private: 00097 ServerSocket server; 00098 map<string, FunctionPointer> handlerMap; 00099 char *buf; 00100 int length; 00101 00102 void callHandler(string name) { 00103 for (map<string, FunctionPointer>::iterator it = handlerMap.begin(); it != handlerMap.end(); it++) { 00104 if (name.compare(it->first) == 0) { 00105 (it->second).call(); 00106 break; 00107 } 00108 } 00109 } 00110 }; 00111 00112 #endif
Generated on Wed Jul 20 2022 21:57:16 by
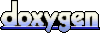