Station API
Embed:
(wiki syntax)
Show/hide line numbers
PachubeClient.h
00001 /* 00002 Copyright (c) 2011, Senio Networks, Inc. 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #ifndef PACHUBE_CLIENT_H 00024 #define PACHUBE_CLIENT_H 00025 00026 #include "SimpleSocket.h" 00027 #include "Utils.h" 00028 00029 /** 00030 * Client API for accessing Pachube 00031 */ 00032 class PachubeClient { 00033 public: 00034 /** 00035 * creates an PachubeClient object 00036 * 00037 * @param feedid Feed ID 00038 * @param apikey API Key 00039 * @param verbose if true display debug info 00040 */ 00041 PachubeClient(int feedid, char *apikey, bool verbose = false) 00042 : feedid(feedid), length(0), verbose(verbose) { 00043 strcpy(this->apikey, apikey); 00044 } 00045 00046 /** 00047 * creates an PachubeClient object 00048 * 00049 * @param filename name of the configuration file 00050 * @param verbose if true display debug info 00051 */ 00052 static PachubeClient create(char *filename, bool verbose = false) { 00053 int feedid = 0; 00054 char apikey[64] = {}; 00055 00056 if (filename) { 00057 char path[32]; 00058 LocalFileSystem local("local"); 00059 sprintf(path, "/local/%s", filename); 00060 if (FILE *fp = fopen(path, "r")) { 00061 Utils::fgetValues(fp, "feed-id:%d", &feedid); 00062 Utils::fgetValues(fp, "api-key:%s", apikey); 00063 fclose(fp); 00064 if (verbose) { 00065 printf("feed-id = %d\n", feedid); 00066 printf("api-key = %s\n", apikey); 00067 } 00068 } 00069 } 00070 00071 return PachubeClient(feedid, apikey, verbose); 00072 } 00073 00074 /** 00075 * adds data to the Pachube datastream 00076 * 00077 * @param id Datastream ID 00078 * @param value value to add 00079 */ 00080 void add(int id, float value) { 00081 length += sprintf(&data[length], "%d,%f\n", id, value); 00082 } 00083 00084 /** 00085 * adds data to the Pachube datastream 00086 * 00087 * @param id Datastream ID 00088 * @param value value to add 00089 */ 00090 void add(char *id, float value) { 00091 length += sprintf(&data[length], "%s,%f\n", id, value); 00092 } 00093 00094 /** 00095 * updates datastreams 00096 * 00097 * @returns true if succeeded 00098 */ 00099 bool update() { 00100 bool result = false; 00101 00102 ClientSocket client("api.pachube.com", 80); 00103 if (client) { 00104 const char *request = "PUT /v2/feeds/%d.csv HTTP/1.1\r\n" 00105 "Host: api.pachube.com\r\n" 00106 "X-PachubeApiKey: %s\r\n" 00107 "Content-Type: text/csv\r\n" 00108 "Content-Length: %d\r\n" 00109 "Connection: close\r\n\r\n"; 00110 00111 client.printf(request, feedid, apikey, length); 00112 client.write(data, length); 00113 if (verbose) { 00114 printf(request, feedid, apikey, length); 00115 printf("%s", data); 00116 } 00117 00118 while (client) { 00119 if (client.available()) { 00120 while (client.available()) { 00121 char response[128] = {}; 00122 client.read(response, sizeof(response) - 1); 00123 if (!result && strncmp(response, "HTTP/1.1 200", 12) == 0) 00124 result = true; 00125 if (verbose) 00126 printf("%s", response); 00127 } 00128 client.close(); 00129 } 00130 } 00131 } 00132 length = 0; 00133 return result; 00134 } 00135 00136 private: 00137 int feedid; 00138 int length; 00139 bool verbose; 00140 char apikey[64]; 00141 char data[64]; 00142 }; 00143 00144 #endif
Generated on Wed Jul 20 2022 21:57:16 by
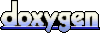