Station API
Embed:
(wiki syntax)
Show/hide line numbers
NTPClient.h
00001 /* 00002 Copyright (c) 2011, Senio Networks, Inc. 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #include "SimpleSocket.h" 00024 #include "Utils.h" 00025 00026 /** 00027 * NTP Client interface to adjust RTC 00028 */ 00029 class NTPClient { 00030 public: 00031 /** 00032 * creates an NTPClient object 00033 * 00034 * @param server NTP server host name 00035 * @param timeout max wait time for connection 00036 */ 00037 NTPClient(char *server = "pool.ntp.org", float timeout = 3.0) : timeout(timeout) { 00038 strncpy(this->server, server, sizeof(this->server)); 00039 } 00040 00041 /** 00042 * creates an NTPClient object from a config file 00043 * 00044 * @param filename name for the configuration file containing server host name and timeout 00045 * @param verbose if true display debug info 00046 */ 00047 static NTPClient create(char *filename, bool verbose = false) { 00048 char server[32] = "pool.ntp.org"; 00049 float timeout = 3.0; 00050 00051 if (filename) { 00052 char path[32]; 00053 LocalFileSystem local("local"); 00054 sprintf(path, "/local/%s", filename); 00055 if (FILE *fp = fopen(path, "r")) { 00056 Utils::fgetValues(fp, "ntp-server:%s", server); 00057 Utils::fgetValues(fp, "ntp-timeout:%f", &timeout); 00058 fclose(fp); 00059 if (verbose) { 00060 printf("ntp-server:%s\n", server); 00061 printf("ntp-timeout:%2.1f\n", timeout); 00062 } 00063 } 00064 } 00065 00066 return NTPClient(server, timeout); 00067 } 00068 00069 /** 00070 * adjusts RTC 00071 * 00072 * @returns true if succeeded 00073 */ 00074 bool setTime() { 00075 Timer timer; 00076 timer.start(); 00077 while (timer.read() < timeout) { 00078 DatagramSocket datagram; 00079 char buf[48] = {0x23}; // 00100011 LI(0), Version(4), Mode(3: Client) 00080 datagram.write(buf, sizeof(buf)); 00081 datagram.send(server, 123); 00082 00083 if (datagram.receive(0, timeout) > 0) { 00084 if (datagram.read(buf, sizeof(buf))) { 00085 unsigned long seconds = 0; 00086 for (int i = 40; i <= 43; i++) 00087 seconds = (seconds << 8) | buf[i]; 00088 set_time(time_t(seconds - 2208988800ULL)); 00089 return true; 00090 } 00091 } 00092 } 00093 00094 return false; 00095 } 00096 00097 private: 00098 char server[32]; 00099 float timeout; 00100 };
Generated on Wed Jul 20 2022 21:57:16 by
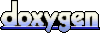