Station API
Embed:
(wiki syntax)
Show/hide line numbers
Location.h
00001 /* 00002 Copyright (c) 2011, Senio Networks, Inc. 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #ifndef LOCATION_H 00024 #define LOCATION_H 00025 00026 #include "Utils.h" 00027 00028 /** 00029 * Location info object 00030 */ 00031 class Location { 00032 public: 00033 /** 00034 * Constructor 00035 * 00036 * @param longitude longitude in degrees 00037 * @param latitude latutude in degrees 00038 * @param elevation elevation in meters 00039 */ 00040 Location(double longitude = 0, double latitude = 0, float elavation = 0) 00041 : longitude(longitude), latitude(latitude), elevation(elevation) {} 00042 00043 /** 00044 * creates a Location object from a config file 00045 * 00046 * @param filename name of the config file 00047 * @param verbose if true display debug info 00048 */ 00049 static Location create(char *filename, bool verbose = false) { 00050 double longitude = 0, latitude = 0; 00051 float elevation = 0; 00052 00053 if (filename) { 00054 char path[32]; 00055 LocalFileSystem local("local"); 00056 sprintf(path, "/local/%s", filename); 00057 if (FILE *fp = fopen(path, "r")) { 00058 Utils::fgetValues(fp, "longitude:%lf", &longitude); 00059 Utils::fgetValues(fp, "latitude:%lf", &latitude); 00060 Utils::fgetValues(fp, "elevation:%f", &elevation); 00061 fclose(fp); 00062 if (verbose) { 00063 printf( "longitude:%lf\n", longitude); 00064 printf( "latitude:%lf\n", latitude); 00065 printf( "elevation:%f\n", elevation); 00066 } 00067 } 00068 } 00069 00070 return Location(longitude, latitude, elevation); 00071 } 00072 00073 /** 00074 * Returns longitude 00075 * 00076 * @returns longitude in degrees 00077 */ 00078 double getLongitude() { 00079 return longitude; 00080 } 00081 00082 /** 00083 * Sets longitude 00084 * 00085 * @param longitude longitude in degrees 00086 */ 00087 void setLongitude(double longitude) { 00088 this->longitude = longitude; 00089 } 00090 00091 /** 00092 * Returns longitude 00093 * 00094 * @returns longitude in degrees 00095 */ double getLatitude() { 00096 return latitude; 00097 } 00098 00099 /** 00100 * Sets latitude 00101 * 00102 * @param latitude latitude in degrees 00103 */ 00104 void setLatitude(double latinude) { 00105 this->latitude = latitude; 00106 } 00107 00108 /** 00109 * Returns elevation 00110 * 00111 * @returns elevation in meters 00112 */ 00113 float getElevation() { 00114 return elevation; 00115 } 00116 00117 /** 00118 * Sets elevation 00119 * 00120 * @param elevation elevation in meters 00121 */ 00122 void setElavation(float elevation) { 00123 this->elevation = elevation; 00124 } 00125 00126 private: 00127 double longitude, latitude; 00128 float elevation; 00129 }; 00130 00131 #endif
Generated on Wed Jul 20 2022 21:57:16 by
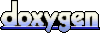