Station API
Embed:
(wiki syntax)
Show/hide line numbers
GMCounter.h
00001 /* 00002 Copyright (c) 2011, Senio Networks, Inc. 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #ifndef GMCOUNTER_H 00024 #define GMCOUNTER_H 00025 00026 #include "mbed.h" 00027 #include "Utils.h" 00028 00029 /** 00030 * class for Geiger Mueller Counter 00031 */ 00032 class GMCounter { 00033 public: 00034 /** 00035 * Constructor 00036 * 00037 * @param gmcPin pin for GMC input 00038 * @param buzzerPin pin for buzzer output 00039 * @param ledPin pin for LED output 00040 * @param cpm2usv conversion ratio of CPM to uSv 00041 * @param buzzerEnabled if true enable buzzer 00042 * @param ledEnabled if true enable LED 00043 */ 00044 GMCounter(PinName gmcPin, PinName buzzerPin, PinName ledPin, float cpm2usv = 1.0F / 120, bool buzzerEnabled = true, bool ledEnabled = true) 00045 : interrupt(gmcPin), buzzer(buzzerPin), led(ledPin), cpm2usv(cpm2usv), buzzerEnabled(buzzerEnabled), ledEnabled(ledEnabled), 00046 counter(0), index(0), index60(0), elapsed(0) { 00047 memset(count, 0, sizeof(count)); 00048 memset(count60, 0, sizeof(count60)); 00049 ticker.attach(this, &GMCounter::tickerHandler, 1); 00050 ticker60.attach(this, &GMCounter::ticker60Handler, 60); 00051 interrupt.rise(this, &GMCounter::interruptHandler); 00052 } 00053 00054 /** 00055 * creates an GMCounter object 00056 * 00057 * @param gmcPin pin for GMC input 00058 * @param buzzerPin pin for buzzer output 00059 * @param ledPin pin for LED output 00060 * @param filename name of the config file 00061 * @param verbose if true display debug info 00062 * 00063 * @returns GMCounter object 00064 */ 00065 static GMCounter create(PinName gmcPin, PinName buzzerPin, PinName ledPin, char *filename, bool verbose = false) { 00066 bool buzzerEnabled = true, ledEnabled = true; 00067 float cpm2usv = 1.0F / 120; 00068 00069 if (filename) { 00070 char path[32]; 00071 LocalFileSystem local("local"); 00072 sprintf(path, "/local/%s", filename); 00073 if (FILE *fp = fopen(path, "r")) { 00074 Utils::fgetValues(fp, "set-buzzer:%d", &buzzerEnabled); 00075 Utils::fgetValues(fp, "set-led:%d", &ledEnabled); 00076 Utils::fgetValues(fp, "cpm-to-usv:%f", &cpm2usv); 00077 fclose(fp); 00078 if (verbose) { 00079 printf("set-buzzer:%d\n", buzzerEnabled); 00080 printf("set-led:%d\n", ledEnabled); 00081 printf("cpm-to-usv:%f\n", cpm2usv); 00082 } 00083 } 00084 } 00085 00086 return GMCounter(gmcPin, buzzerPin, ledPin, cpm2usv, buzzerEnabled, ledEnabled); 00087 } 00088 00089 /** 00090 * gets CPM (Counts Per Minute) value 00091 * 00092 * @returns counts during the last 60 seconds 00093 */ 00094 int getCPM() { 00095 // make sure that no index updated while reading the array entries 00096 // if there was (interrupt happened!), retry reading. 00097 int i, cpm; 00098 do { 00099 i = index; 00100 cpm = count[(i - 1 + 61) % 61] - count[i]; 00101 } while (i != index); 00102 00103 return cpm; 00104 } 00105 00106 /** 00107 * gets average CPM (Counts Per Minute) 00108 * 00109 * @returns average CPM during the last 60 minutes 00110 */ 00111 float getAverageCPM() { 00112 // make sure that no index60 updated while reading the array entries 00113 // if there was (interrupt happened!), retry reading. 00114 int i, total; 00115 do { 00116 i = index60; 00117 total = count60[(i - 1 + 61) % 61] - count60[i]; 00118 } while (i != index60); 00119 00120 return elapsed == 0 ? 0 : elapsed < 60.0 ? total / elapsed : total / 60.0; 00121 } 00122 00123 /** 00124 * Returns radiation 00125 * 00126 * @returns radiation in uSv 00127 */ 00128 float getRadiation() { 00129 return getCPM() * cpm2usv; 00130 } 00131 00132 /** 00133 * Returns average radiation 00134 * 00135 * @returns average radiation during last 60 minutes in uSv 00136 */ 00137 float getAverageRadiation() { 00138 return getAverageCPM() * cpm2usv; 00139 } 00140 00141 /** 00142 * returns buzzer status 00143 * 00144 * @returns buzzer status 00145 */ 00146 bool getBuzzer() { 00147 return buzzerEnabled; 00148 } 00149 00150 /** 00151 * sets buzzer enabled or disabled 00152 */ 00153 void setBuzzer(bool enable = true) { 00154 buzzerEnabled = enable; 00155 } 00156 00157 /** 00158 * returns LED status 00159 * 00160 * @returns LED status 00161 */ 00162 bool getLED() { 00163 return ledEnabled; 00164 } 00165 00166 /** 00167 * sets LED enabled or disabled 00168 */ 00169 void setLED(bool enable = true) { 00170 ledEnabled = enable; 00171 } 00172 00173 /** 00174 * sets CPM to uSv conversion ration 00175 * 00176 * @param cpm2usv conversion ratio uSv/CPM 00177 */ 00178 void setConversionRatio(float cpm2usv) { 00179 this->cpm2usv = cpm2usv; 00180 } 00181 00182 private: 00183 InterruptIn interrupt; 00184 DigitalOut buzzer; 00185 DigitalOut led; 00186 Timeout timeout; 00187 Ticker ticker, ticker60; 00188 float cpm2usv; 00189 bool buzzerEnabled; 00190 bool ledEnabled; 00191 unsigned int counter; 00192 unsigned int count[61]; 00193 unsigned int count60[61]; 00194 int index; 00195 int index60; 00196 float elapsed; 00197 00198 void interruptHandler() { 00199 counter++; 00200 if (buzzerEnabled) buzzer = 1; 00201 if (ledEnabled) led = 1; 00202 timeout.attach(this, &GMCounter::timeoutHandler, 0.03); 00203 } 00204 00205 void tickerHandler() { 00206 count[index] = counter; 00207 index = (index + 1) % 61; 00208 } 00209 00210 void ticker60Handler() { 00211 if (elapsed < 60) elapsed++; 00212 count60[index60] = counter; 00213 index60 = (index60 + 1) % 61; 00214 } 00215 00216 void timeoutHandler() { 00217 if (buzzerEnabled) buzzer = 0; 00218 if (ledEnabled) led = 0; 00219 } 00220 }; 00221 00222 #endif
Generated on Wed Jul 20 2022 21:57:16 by
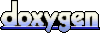