Station API
Embed:
(wiki syntax)
Show/hide line numbers
Ether.h
00001 /* 00002 Copyright (c) 2011, Senio Networks, Inc. 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #include "EthernetNetIf.h" 00024 #include "Utils.h" 00025 00026 /** 00027 * A wrapper class for EthernetNetIf to create an instance from a config file 00028 */ 00029 class Ether { 00030 public: 00031 /** 00032 * creates Ether instance 00033 * 00034 * @param verbose if true display debug info 00035 */ 00036 Ether(bool verbose = false) 00037 : active(false), verbose(verbose) { 00038 } 00039 00040 /** 00041 * creates Ether instance 00042 * 00043 * @param ip IP address 00044 * @param subnet subnet mask 00045 * @param gateway gateway address 00046 * @param dns DNS server address 00047 * @param verbose if true display debug info 00048 */ 00049 Ether(IpAddr ip, IpAddr subnet, IpAddr gateway, IpAddr dns, bool verbose = false) 00050 : eth(ip, subnet, gateway, dns), active(false), verbose(verbose) { 00051 } 00052 00053 /** 00054 * creates Ether instance 00055 * 00056 * @param ip IP address 00057 * @param subnet subnet mask 00058 * @param gateway gateway address 00059 * @param dns DNS server address 00060 * @param verbose if true display debug info 00061 */ 00062 static Ether create(IpAddr ip, IpAddr subnet, IpAddr gateway, IpAddr dns, bool verbose = false) { 00063 return Ether(ip, subnet, gateway, dns, verbose); 00064 } 00065 00066 /** 00067 * creates Ether instance 00068 * 00069 * @param filename configuration file containing IP address, subnet mask, gateway and DNS address 00070 * @param verbose if true display debug info 00071 */ 00072 static Ether create(char *filename = 0, bool verbose = false) { 00073 if (filename) { 00074 char path[32]; 00075 LocalFileSystem local("local"); 00076 sprintf(path, "/local/%s", filename); 00077 if (FILE *fp = fopen(path, "r")) { 00078 char b1, b2, b3, b4; 00079 IpAddr ip = Utils::fgetValues(fp, "ip-address:%hhu.%hhu.%hhu.%hhu", &b1, &b2, &b3, &b4) ? IpAddr(b1, b2, b3, b4) : IpAddr(); 00080 IpAddr subnet = Utils::fgetValues(fp, "subnet-mask:%hhu.%hhu.%hhu.%hhu", &b1, &b2, &b3, &b4) ? IpAddr(b1, b2, b3, b4) : IpAddr(); 00081 IpAddr gateway = Utils::fgetValues(fp, "gateway-address:%hhu.%hhu.%hhu.%hhu", &b1, &b2, &b3, &b4) ? IpAddr(b1, b2, b3, b4) : IpAddr(); 00082 IpAddr dns = Utils::fgetValues(fp, "dns-address:%hhu.%hhu.%hhu.%hhu", &b1, &b2, &b3, &b4) ? IpAddr(b1, b2, b3, b4) : IpAddr(); 00083 fclose(fp); 00084 if (verbose) { 00085 printf("ip-address:%hhu.%hhu.%hhu.%hhu\n", ip[0], ip[1], ip[2], ip[3]); 00086 printf("subnet-mask:%hhu.%hhu.%hhu.%hhu\n", subnet[0], subnet[1], subnet[2], subnet[3]); 00087 printf("gateway-address:%hhu.%hhu.%hhu.%hhu\n", gateway[0], gateway[1], gateway[2], gateway[3]); 00088 printf("dns-address:%hhu.%hhu.%hhu.%hhu\n", dns[0], dns[1], dns[2], dns[3]); 00089 } 00090 00091 return Ether(ip, subnet, gateway, dns, verbose); 00092 } 00093 } 00094 00095 return Ether(); 00096 } 00097 00098 /** 00099 * sets up Ethernet interface 00100 */ 00101 void setup() { 00102 EthernetErr err = eth.setup(); 00103 active = err == ETH_OK; 00104 if (verbose) { 00105 printf("EthernetNetIf.setup() = %d\n", err); 00106 } 00107 } 00108 00109 /** 00110 * returns Ethernet status 00111 * 00112 * @returns true if setup succeeded 00113 */ 00114 bool isActive() { 00115 return active; 00116 } 00117 00118 /** 00119 * returns its IP address in bytes 00120 * 00121 * @returns IP address in byte array 00122 */ 00123 char *getIpAddress() { 00124 static char ip[] = {eth.getIp()[0], eth.getIp()[1], eth.getIp()[2], eth.getIp()[3]}; 00125 return ip; 00126 } 00127 00128 private: 00129 EthernetNetIf eth; 00130 bool active; 00131 bool verbose; 00132 };
Generated on Wed Jul 20 2022 21:57:16 by
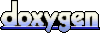