
SimpleSocket 1.0 examples
Dependencies: EthernetNetIf SimpleSocket 1.0 mbed
ntpclient.cpp
00001 #include "EthernetNetIf.h" 00002 #include "SimpleSocket.h" 00003 00004 void ntpclient() { 00005 EthernetNetIf eth; 00006 eth.setup(); 00007 00008 char *NTP_SERVER = "pool.ntp.org"; 00009 printf("ntp server = %s\n", NTP_SERVER); 00010 00011 while (true) { 00012 DatagramSocket datagram; 00013 char buf[48] = {0x23}; // 00100011 LI(0), Version(4), Mode(3: Client) 00014 datagram.write(buf, sizeof(buf)); 00015 datagram.send(NTP_SERVER, 123); 00016 00017 if (datagram.receive() > 0) { 00018 if (datagram.read(buf, sizeof(buf)) > 0) { 00019 unsigned long seconds = 0; 00020 for (int i = 40; i <= 43; i++) 00021 seconds = (seconds << 8) | buf[i]; 00022 set_time(time_t(seconds - 2208988800ULL)); 00023 char timestamp[16]; 00024 time_t jstime = time(NULL) + 9 * 3600; 00025 strftime(timestamp, sizeof(timestamp), "%m/%d %X", localtime(&jstime)); 00026 printf("Time: %s\n", timestamp); 00027 break; 00028 } 00029 } else { 00030 printf("no answer\n"); 00031 wait(1.0); 00032 } 00033 } 00034 }
Generated on Wed Jul 13 2022 16:35:12 by
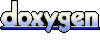