
SimpleSocket 1.0 examples
Dependencies: EthernetNetIf SimpleSocket 1.0 mbed
ntpclient2.cpp
00001 #include "EthernetNetIf.h" 00002 #include "SimpleSocket.h" 00003 00004 typedef unsigned long long Time64; 00005 00006 Time64 toTime64(char buf[]) { 00007 Time64 time64 = 0; 00008 for (int i = 0; i < 8; i++) { 00009 time64 = (time64 << 8) | buf[i]; 00010 } 00011 return time64; 00012 } 00013 00014 Time64 toTime64(int usecs) { 00015 return Time64(double(usecs) * (1ULL << 32) / 1000000); 00016 } 00017 00018 void printTime64(char *title, Time64 time64) { 00019 unsigned long seconds = (unsigned long) (time64 >> 32); 00020 unsigned long subsecs = (unsigned long) (time64 & 0xFFFFFFFFULL); 00021 char buf[16]; 00022 00023 time_t jstime = time_t(seconds - 2208988800UL) + 9 * 3600; 00024 strftime(buf, sizeof(buf), "%m/%d %X", localtime(&jstime)); 00025 printf("%s: %s", title, buf); 00026 sprintf(buf, "%f\n", (double) subsecs / (1ULL << 32)); 00027 printf("%s", &buf[1]); 00028 } 00029 00030 void ntpclient2() { 00031 EthernetNetIf eth; 00032 eth.setup(); 00033 00034 char *NTP_SERVER = "pool.ntp.org"; 00035 char buf[48] = {0x23};// 00100011 LI(0), Version(4), Mode(3: Client) 00036 Timer timer; 00037 Time64 adjustedTime = 0; 00038 00039 for (int count = 0; count < 5; count++) { 00040 buf[0] = 0x23; 00041 DatagramSocket datagram; 00042 datagram.write(buf, sizeof(buf)); 00043 timer.reset(); 00044 datagram.send(NTP_SERVER, 123); 00045 timer.start(); 00046 if (datagram.receive() > 0) { 00047 int turnaround = timer.read_us(); 00048 if (datagram.read(buf, sizeof(buf))) { 00049 Time64 receivedTime = toTime64(&buf[32]); 00050 Time64 transferTime = toTime64(&buf[40]); 00051 adjustedTime = toTime64(turnaround / 2) + receivedTime / 2 + transferTime / 2; 00052 timer.reset(); 00053 timer.start(); 00054 printTime64("transfer", transferTime); 00055 printTime64("adjusted", adjustedTime); 00056 printf("\n"); 00057 } 00058 } else { 00059 wait(5); 00060 } 00061 } 00062 00063 float subsecs = (double) (adjustedTime & 0xFFFFFFFFULL) / (1ULL << 32); 00064 wait(1 - subsecs); 00065 set_time((size_t) ((adjustedTime >> 32) - 2208988800UL) + 1); 00066 00067 time_t jstime = time(NULL) + 9 * 3600; 00068 strftime(buf, sizeof(buf), "%m/%d %X", localtime(&jstime)); 00069 printf("RTC delta = %6d, %s\n\n", timer.read_us(), buf); 00070 00071 for (int count = 0; count < 20; count++) { 00072 buf[0] = 0x23; 00073 DatagramSocket datagram; 00074 datagram.write(buf, sizeof(buf)); 00075 timer.reset(); 00076 datagram.send(NTP_SERVER, 123); 00077 timer.start(); 00078 if (datagram.receive() > 0) { 00079 int turnaround = timer.read_us(); 00080 if (datagram.read(buf, sizeof(buf))) { 00081 Time64 receivedTime = toTime64(&buf[32]); 00082 Time64 transferTime = toTime64(&buf[40]); 00083 adjustedTime = toTime64(turnaround / 2) + receivedTime / 2 + transferTime / 2; 00084 printTime64("adjusted", adjustedTime); 00085 time_t jstime = time(NULL) + 9 * 3600; 00086 strftime(buf, sizeof(buf), "%m/%d %X", localtime(&jstime)); 00087 printf(" RTC: %s\n\n", buf); 00088 } 00089 } else { 00090 wait(5); 00091 } 00092 } 00093 00094 }
Generated on Wed Jul 13 2022 16:35:12 by
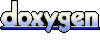