
SimpleSocket 1.0 examples
Dependencies: EthernetNetIf SimpleSocket 1.0 mbed
multicast.cpp
00001 #include "EthernetNetIf.h" 00002 #include "SimpleSocket.h" 00003 00004 void multicast() { 00005 EthernetNetIf eth; 00006 eth.setup(); 00007 00008 Host multicast(IpAddr(239, 192, 1, 100), 50000); 00009 DatagramSocket datagram(multicast); 00010 00011 while (true) { 00012 Host host; 00013 if (datagram.receive(&host, 1 + (rand() % 5) / 3.0) > 0) { 00014 int value; 00015 datagram.scanf("%d", &value); 00016 IpAddr ip = host.getIp(); 00017 printf("received from %d.%d.%d.%d:%d %d\n", ip[0], ip[1], ip[2], ip[3], host.getPort(), value); 00018 } else { 00019 char* message = "12345!"; 00020 datagram.printf(message); 00021 datagram.send(multicast); 00022 printf("sent: %s\n", message); 00023 wait(1); 00024 } 00025 } 00026 }
Generated on Wed Jul 13 2022 16:35:12 by
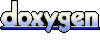