
SimpleSocket 1.0 examples
Dependencies: EthernetNetIf SimpleSocket 1.0 mbed
httpclient.cpp
00001 #include "EthernetNetIf.h" 00002 #include "SimpleSocket.h" 00003 00004 void httpclient() { 00005 EthernetNetIf eth; 00006 eth.setup(); 00007 00008 printf("URL => "); 00009 char url[128]; 00010 scanf("%s", url); 00011 00012 char hostpart[64], host[64], path[128]; 00013 int port = 80; 00014 sscanf(url, "http://%[^/]%s", hostpart, path); 00015 sscanf(hostpart, "%[^:]:%d", host, &port); 00016 00017 ClientSocket socket(host, port); 00018 00019 if (socket.connected()) { 00020 socket.printf("GET %s HTTP/1.0\r\n\r\n", path); 00021 00022 while (socket) { 00023 if (socket.available()) { 00024 char buf[128] = {}; 00025 socket.read(buf, sizeof(buf) - 1); 00026 printf("%s", buf); 00027 } 00028 } 00029 } 00030 }
Generated on Wed Jul 13 2022 16:35:12 by
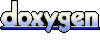