
SCP1000 Logger example
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "SCP1000.h" 00003 00004 DigitalIn sw(p9); 00005 DigitalOut leds[4] = {LED1, LED2, LED3, LED4}; 00006 SCP1000 scp1000(p5, p6, p7, p8); 00007 LocalFileSystem local("local"); 00008 void printMeasurements(char *title, int n); 00009 00010 int main() { 00011 char buf[32]; 00012 00013 for (int i = 0; !sw; i = (i + 1) % 8) { 00014 leds[i < 4 ? i : 7 - i] = 1; 00015 wait(0.05); 00016 leds[i < 4 ? i : 7 - i] = 0; 00017 } 00018 00019 FILE *fp = fopen("/local/scp1000.txt", "a"); 00020 if (fp == NULL) exit(-1); 00021 00022 time_t seconds = time(NULL); 00023 strftime(buf, sizeof(buf), "%x %X", localtime(&seconds)); 00024 fprintf(fp, "Time: %s\n", buf); 00025 fprintf(fp, "SCP1000 ASIC revision number = %d\n", scp1000.revision()); 00026 bool result = scp1000.performSelfTest(); 00027 fprintf(fp, "SCP1000 self test: %s\n", result ? "PASSED" : "FAILED"); 00028 fprintf(fp, "SCP1000 reset\n"); 00029 scp1000.reset(); 00030 fclose(fp); 00031 00032 scp1000.setOperationMode(SCP1000::HIGH_SPEED_MODE); 00033 printMeasurements("SCP1000 High Speed Mode - 9Hz", 3600 * 9); 00034 00035 scp1000.setOperationMode(SCP1000::HIGH_RESOLUTION_MODE); 00036 printMeasurements("SCP1000 High Resolution Mode - 1.8Hz", 3600 * 9 / 5); 00037 00038 scp1000.setOperationMode(SCP1000::ULTRA_LOW_POWER_MODE); 00039 printMeasurements("SCP1000 Ultra Low Power Mode - 1Hz", 3600 * 10); 00040 } 00041 00042 void printMeasurements(char *title, int n) { 00043 FILE *fp = fopen("/local/scp1000.txt", "a"); 00044 fprintf(fp, "%s\n", title); 00045 00046 for (int i = 0; !sw && i < n; i++) { 00047 while (!scp1000.isReady()) 00048 ; 00049 time_t seconds = time(NULL); 00050 char buf[16]; 00051 strftime(buf, sizeof(buf), "%X", localtime(&seconds)); 00052 fprintf(fp, "%s %3.2f, %3.3f\n", buf, scp1000.readTemperature(), scp1000.readPressure()); 00053 leds[i % 4] = (i % 8) < 4; 00054 if (i % 100 == 99) { 00055 fclose(fp); 00056 fp = fopen("/local/scp1000.txt", "a"); 00057 } 00058 } 00059 00060 fclose(fp); 00061 wait(1.0); 00062 }
Generated on Mon Jul 18 2022 11:35:28 by
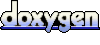