MMA7361L interface
Dependents: MMA7361L_Example ARLISS2012_Hidaka
MMA7361L.h
00001 #ifndef MMA7361L_H 00002 #define MMA7361L_H 00003 00004 #include "mbed.h" 00005 00006 /** 00007 * MMA7361L accelerometer 00008 */ 00009 class MMA7361L { 00010 public: 00011 /** 00012 * Creates an MMA7361L accelerometer interface, connected to the specified pins 00013 * 00014 * @param xoutPin xout pin 00015 * @param youtPin yout pin 00016 * @param zoutPin zout pin 00017 * @param zeroGDetectPin 0G detect pin 00018 * @param gSelectPin select 1.5G/6G pin 00019 * @param nSleepPin ~sleep pin 00020 * 00021 */ 00022 MMA7361L(PinName xoutPin, PinName youtPin, PinName zoutPin, PinName zeroGDetectPin = NC, PinName gSelectPin = NC, PinName sleepPin = NC); 00023 00024 /** 00025 * Scale enumeration declaration 00026 */ 00027 enum Scale { 00028 00029 /** 00030 * 1.5G mode 00031 */ 00032 SCALE_1_5G, 00033 00034 /** 00035 * 6G mode 00036 */ 00037 SCALE_6G 00038 }; 00039 00040 /** 00041 * Gets the current total acceleration 00042 * 00043 * @param forceRead true to read the device, false to use cache whenever appropriate 00044 * @returns total acceleration in g 00045 */ 00046 float getAccel(bool forceRead = false); 00047 00048 /** 00049 * Gets the current acceleration along the X axis 00050 * 00051 * @param forceRead true to read the device, false to use cache whenever appropriate 00052 * @returns acceleration along the X axis 00053 */ 00054 float getAccelX(bool forceRead = false); 00055 00056 /** 00057 * Gets the current acceleration along the Y axis 00058 * 00059 * @param forceRead true to read the device, false to use cache whenever appropriate 00060 * @returns acceleration along the Y axis 00061 */ 00062 float getAccelY(bool forceRead = false); 00063 00064 /** 00065 * Gets the current acceleration along the Z axis 00066 * 00067 * @param forceRead true to read the device, false to use cache whenever appropriate 00068 * @returns acceleration along the Z axis 00069 */ 00070 float getAccelZ(bool forceRead = false); 00071 00072 /** 00073 * Computes the inclination of the X axis 00074 * 00075 * @param forceRead true to read the device, false to use cache whenever appropriate 00076 * @returns the inclination of the X axis 00077 */ 00078 float getTiltX(bool forceRead = false); 00079 00080 /** 00081 * Computes the inclination of the Y axis 00082 * 00083 * @param forceRead true to read the device, false to use cache whenever appropriate 00084 * @returns the inclination of the Y axis 00085 */ 00086 float getTiltY(bool forceRead = false); 00087 00088 /** 00089 * Computes the inclination of the Z axis 00090 * 00091 * @param forceRead true to read the device, false to use cache whenever appropriate 00092 * @returns the inclination of the Z axis 00093 */ 00094 float getTiltZ(bool forceRead = false); 00095 00096 /** 00097 * Specifies the scale to use 00098 * 00099 * @param scale SCALE_1_5G (for 1.5G) or SCALE_6G (for 6G) 00100 */ 00101 void setScale(Scale scale); 00102 00103 /** 00104 * Sets sleep mode 00105 * 00106 * @param on true to activate sleep mode, false to resume normal operation 00107 */ 00108 void setSleep(bool on); 00109 00110 /** 00111 * Tests whether 0G is detected 00112 * 00113 * @returns true if 0G is detected, false otherwise 00114 */ 00115 bool zeroGDetected(); 00116 00117 /** 00118 * Sets fucntion to be called when 0G is detected 00119 * 00120 * @param func pointer to a function called when 0G is detected, 0 to reset as none 00121 */ 00122 void setZeroGDetectListener(void (*func)(void)); 00123 00124 /** 00125 * Sets member fucntion to be called when 0G is detected 00126 * 00127 * @param t pointer to the object to call the member function on 00128 * @param func pointer to the member function to be called when 0G is detected, 0 to reset as none 00129 */ 00130 template<typename T> 00131 void setZeroGDetectListener(T* t, void (T::*func)(void)); 00132 00133 /** 00134 * Prints instance info for debugging 00135 */ 00136 void printInfo(); 00137 00138 /** 00139 * Sets calibration info 00140 * 00141 * @param scale scale for calibration 00142 * @param minX min X value when the X axis is vertical and stable under 1g 00143 * @param maxX max X value when the X axis is vertical and stable under 1g 00144 * @param minY min Y value when the Y axis is vertical and stable under 1g 00145 * @param maxY max Y value when the Y axis is vertical and stable under 1g 00146 * @param minZ min Z value when the Z axis is vertical and stable under 1g 00147 * @param maxZ max Z value when the Z axis is vertical and stable under 1g 00148 */ 00149 00150 void calibrate(Scale scale, float minX, float maxX, float minY, float maxY, float minZ, float maxZ); 00151 00152 private: 00153 enum { 00154 ACCEL = 1 << 0, 00155 ACCEL_X = 1 << 1, 00156 ACCEL_Y = 1 << 2, 00157 ACCEL_Z = 1 << 3, 00158 TILT_X = 1 << 4, 00159 TILT_Y = 1 << 5, 00160 TILT_Z = 1 << 6 00161 }; 00162 00163 AnalogIn xout, yout, zout; 00164 InterruptIn zeroGDetect; 00165 DigitalOut gSelect; 00166 DigitalOut nSleep; 00167 bool zeroGDetectEnabled; 00168 bool gSelectEnabled; 00169 bool sleepEnabled; 00170 Scale scale; 00171 float ratio; 00172 float accelX, accelY, accelZ; 00173 int flags; 00174 struct Calibration { 00175 float minX, maxX; 00176 float minY, maxY; 00177 float minZ, maxZ; 00178 } calib[2]; 00179 00180 void prepare(int type, bool forceRead); 00181 float calibrate(float value, int type, Scale scale); 00182 }; 00183 00184 #endif
Generated on Sat Jul 23 2022 18:02:38 by
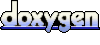