test
Dependencies: EthernetNetIf mbed
Fork of HTTPServer by
main.c
00001 #include "mbed.h" 00002 #include "EthernetNetIf.h" 00003 #include "HTTPServer.h" 00004 00005 00006 EthernetNetIf eth( 00007 IpAddr(192,168,1,8), //IP address 00008 IpAddr(255,255,255,0), //Subnet mask 00009 IpAddr(192,168,1,1), //Gateway 00010 IpAddr(192,168,1,1) //DNS 00011 ); 00012 HTTPServer svr; 00013 00014 DigitalOut led1(LED1); 00015 DigitalOut led2(LED2,"led2"); 00016 LocalFileSystem fs("webfs"); 00017 SPI spi(p5, p6, p7); // mosi, miso, sclk 00018 DigitalOut cs(p8); 00019 float Intempareture,Outtempareture; 00020 00021 int main() { 00022 printf("Setting up...\n"); 00023 EthernetErr ethErr = eth.setup(); 00024 if(ethErr) 00025 { 00026 printf("Error %d in setup.\n", ethErr); 00027 return -1; 00028 } 00029 printf("Setup OK\n"); 00030 00031 FSHandler::mount("/webfs","/"); 00032 svr.addHandler<RPCHandler>("/rpc"); 00033 svr.addHandler<FSHandler>("/"); 00034 //svr.addHandler<SimpleHandler>("/"); //Default handler 00035 svr.bind(80); 00036 00037 printf("Listening...\n"); 00038 00039 Timer tm; 00040 tm.start(); 00041 //Listen indefinitely 00042 00043 // Setup the spi for 8 bit data, high steady state clock, 00044 // second edge capture, with a 1MHz clock rate 00045 spi.format(16,3); 00046 spi.frequency(1000000); 00047 00048 while(true) 00049 { 00050 Net::poll(); 00051 if(tm.read()>.5) 00052 { 00053 led1=!led1; //Show that we are alive 00054 tm.start(); 00055 } 00056 // Select the device by seting chip select low 00057 cs = 0; 00058 00059 // Send 0x8f, the command to read the WHOAMI register 00060 //spi.write(0x0000); 00061 00062 // Send a dummy byte to receive the contents of the WHOAMI register 00063 int outtemp = spi.write(0x0000); 00064 int intemp = spi.write(0x0000); 00065 //printf("outtemp = 0x%X\n", outtemp); 00066 //lcd.locate(0,0); 00067 //lcd.printf("Out:0x%X\n",outtemp); 00068 //printf("intemp = 0x%X\n", intemp); 00069 //lcd.locate(0,1); 00070 //lcd.printf("In :0x%X",intemp); 00071 // Deselect the device 00072 cs = 1; 00073 float disp; 00074 if((outtemp& 0x8000) == 0){ // 0℃以上 00075 disp = (outtemp >> 4) * 0.0625; 00076 } else { // 0℃未満 00077 disp = (((0xffff - outtemp) >> 4) + 1) * -0.0625; 00078 } 00079 Outtempareture=disp; 00080 printf("Out:%4.2f[deg]\n",disp); 00081 00082 if((intemp& 0x8000) == 0){ // 0℃以上 00083 disp = (intemp>> 4) * 0.0625; 00084 } else { // 0℃未満 00085 disp = (((0xffff - intemp) >> 4) + 1) * -0.0625; 00086 } 00087 Intempareture=disp; 00088 printf("In:%4.2f[deg]\n",disp); 00089 //HTML File output 00090 FILE *fp=fopen("/webfs/my.htm","w"); 00091 fprintf(fp,"<html>\r\n"); 00092 fprintf(fp, "<head>\r\n"); 00093 fprintf(fp, "<title>\r\n"); 00094 fprintf(fp, "LED2 ON/OF\r\n"); 00095 fprintf(fp, "</title>\r\n"); 00096 fprintf(fp, "</head>\r\n"); 00097 fprintf(fp, "<body>\r\n"); 00098 /* 00099 fprintf(fp, "<script language=\"javascript\">\n"); 00100 fprintf(fp, "var Button=0; \n"); 00101 fprintf(fp, "function button_push(flug){\n"); 00102 fprintf(fp, "if(Button==0){ Button=1;document.Form.FormButton.value=\"Off\";}\n"); 00103 fprintf(fp, "else{ Button=0; document.Form.FormButton.value=\"On\"; } \n"); 00104 fprintf(fp, "var req=new XMLHttpRequest()\n"); 00105 fprintf(fp, "req.open(\"GET\", \"http://\"+location.host+\"/rpc/led2/write+\"+Button. true); \n"); 00106 fprintf(fp, "req.send(\"\"); \n"); 00107 fprintf(fp, "function tick(){ \n"); 00108 fprintf(fp, "var value=%f;\n",Outtempareture); 00109 fprintf(fp, "document.Form.textbox.value=value;\n"); 00110 fprintf(fp, "var value2=%f;\n",Intempareture); 00111 fprintf(fp, "document.Form.textbox2.value=value2;\n"); 00112 fprintf(fp, "}\n"); 00113 fprintf(fp, "setInterval(\"tick()\",1000);\n"); 00114 fprintf(fp, "</script>\n"); 00115 */ 00116 00117 //fprintf(fp, "<form name=\"Form\" action=\"#\"> \n"); 00118 fprintf(fp, "<font size=5>\n"); 00119 fprintf(fp, "My room Tempareture\n"); 00120 //fprintf(fp, "<input type=\"button\" value=\"On\" name=\"FormButton\" onclick=\"button_push(0)\">\n"); 00121 fprintf(fp, "<br>\n"); 00122 fprintf(fp, "Out temp:\n"); 00123 fprintf(fp, "<font size=8><b>\n"); 00124 fprintf(fp, "%5.2f\n",Outtempareture); 00125 fprintf(fp, "</b></font>\n"); 00126 fprintf(fp, "<br>\n"); 00127 fprintf(fp, "In temp:\n"); 00128 fprintf(fp, "<font size=8><b>\n"); 00129 fprintf(fp, "%5.2f\n",Intempareture); 00130 fprintf(fp, "</b></font>\n"); 00131 fprintf(fp, "<br>\n"); 00132 fprintf(fp, "<hr>\n"); 00133 fprintf(fp, "</font>\n"); 00134 fprintf(fp, " Twitter: <a href=\"https://twitter.com/ynotsu\">@ynotsu</a>"); 00135 fprintf(fp, "</body>\n"); 00136 fprintf(fp, "</html>\n"); 00137 fclose(fp); 00138 00139 //wait(10); 00140 00141 } 00142 00143 return 0; 00144 }
Generated on Tue Jul 12 2022 22:18:59 by
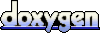