
Trackball based on the NXP LPC11U24 and the ADNS-9500
Dependencies: ADNS9500 USBDevice mbed 25LCxxx_SPI
main.h
00001 /* 00002 * loststone is free sofware: you can redistribute it and/or modify 00003 * it under the terms of the GNU General Public License 3 as published by 00004 * the Free Software Foundation. 00005 * 00006 * loststone is distributed in the hope that it will be useful, 00007 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00008 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00009 * GNU General Public License for more details. 00010 * 00011 * You should have received a copy of the GNU General Public License 00012 * along with loststone. If not, see <http://www.gnu.org/licenses/gpl.txt>. 00013 * 00014 * Copyright (c) 2012-2013 Chris Majoros(chris@majoros.us), GNU3 00015 */ 00016 00017 #include "mbed.h" 00018 #include "USBHID.h" 00019 #include "USBMouse.h" 00020 #include "Ser25lcxxx.h" 00021 00022 #include <stdint.h> 00023 00024 #define ADNS9500_SROM_91 00025 #define ADNS9500_CRCHI (0xBE) 00026 #define ADNS9500_CRCLO (0xEF) 00027 #define ADNS9500_ID (0x56) 00028 #define ADNS6010_FIRMWARE_CRC (0xBEEF) 00029 #define ADNS9500_FIRMWARE_LEN 3070 00030 00031 #include "adns9500.hpp" 00032 00033 00034 #define UINT16(ub, lb) (uint16_t)(((ub & 0xff) << 8) | (lb & 0xff)) 00035 #define INT16(ub, lb) (int16_t)(((ub & 0xff) << 8) | (lb & 0xff)) 00036 00037 #define SETTINGS_BASE 0x00 00038 #define PROFILE_BASE 0xff 00039 #define PROFILE_LEN 0x13 00040 00041 #define MBED 00042 00043 typedef void (*fn)(void); 00044 00045 enum cli_actions { 00046 SET = 0x01, 00047 GET = 0x02, 00048 LOAD_DATA = 0x03, 00049 GET_DATA = 0x04, 00050 CLEAR = 0x05, 00051 INIT = 0x06 00052 }; 00053 00054 enum cli_replies { 00055 retval = 0x01, 00056 message = 0x02 00057 }; 00058 00059 enum buttons { 00060 BUTTON_LEFT = 0x00, //explisitly showing we start at zero 00061 BUTTON_MIDDLE, 00062 BUTTON_RIGHT, 00063 BUTTON_FORWORD, 00064 BUTTON_BACK, 00065 BUTTON_Z, 00066 BUTTON_HIGH_RES, 00067 }; 00068 enum settings { 00069 CPI_X = 0x00, //explisitly showing we start at zero 00070 CPI_Y, 00071 CPI_X_MULITIPLYER, 00072 CPI_Y_MULITIPLYER, 00073 CPI_MAX, // Not currently used. 00074 CPI_MIN, // Not currently used. 00075 CPI_STEP, // Not currently used. 00076 CPI_Z, 00077 CPI_H, 00078 CPI_HR_X, 00079 CPI_HR_Y, 00080 00081 BTN_A, 00082 BTN_B, 00083 BTN_C, 00084 BTN_D, 00085 BTN_E, 00086 BTN_F, 00087 BTN_G, 00088 00089 LED_ACTION, // Not currently used 00090 00091 VID, 00092 PID, 00093 RELEASE, 00094 00095 PROFILE_DEFAULT, 00096 PROFILE_CURRENT, 00097 00098 00099 00100 ADNS_CRC, 00101 ADNS_ID, 00102 ADNS_FW_LEN, 00103 ADNS_FW_OFFSET, 00104 }; 00105 00106 00107 00108 00109 #ifdef MBED 00110 DigitalIn run_mode(p36); 00111 00112 DigitalIn motion_in(p14); 00113 00114 DigitalOut activity(p35); 00115 00116 InterruptIn debug(p29); 00117 00118 InterruptIn btn_a(p21); 00119 InterruptIn btn_b(p22); 00120 InterruptIn btn_c(p23); 00121 InterruptIn btn_d(p24); 00122 InterruptIn btn_e(p25); 00123 InterruptIn btn_f(p26); 00124 InterruptIn btn_g(p27); 00125 00126 InterruptIn prfl_a(p20); 00127 InterruptIn prfl_b(p19); 00128 InterruptIn prfl_c(p18); 00129 InterruptIn prfl_d(p17); 00130 InterruptIn prfl_e(p16); 00131 #elif 00132 DigitalIn run_mode(P1_29); 00133 00134 DigitalIn motion_in(P0_22); 00135 00136 DigitalOut activity(P1_28); 00137 00138 InterruptIn btn_a(P0_18); 00139 InterruptIn btn_b(P0_16); 00140 InterruptIn btn_c(P0_17); 00141 InterruptIn btn_d(P0_23); 00142 InterruptIn btn_e(P1_15); 00143 InterruptIn btn_f(P1_??); 00144 InterruptIn btn_g(P1_??); 00145 00146 InterruptIn prfl_a(P0_4); 00147 InterruptIn prfl_b(P0_5); 00148 InterruptIn prfl_c(P0_21); 00149 InterruptIn prfl_d(P1_23); 00150 InterruptIn prfl_e(P1_24); 00151 #endif 00152 00153 // We are global for the callbacks 00154 USBMouse *mouse; 00155 00156 bool motion_triggered = true; 00157 bool z_axis_active = false; 00158 bool high_rez_active = false; 00159 bool profile_load = true; // Always inishally load the profile even if it might be the same. 00160 bool set_res_hr = false; 00161 bool set_res_z = false; 00162 bool set_res_default = false; 00163 //uint32_t rest_counter; 00164 00165 uint16_t s[28] = { 00166 540, // CPI_X 00167 540, // CPI_Y 00168 40, // CPI_X_MULITIPLYER 00169 40, // CPI_Y_MULITIPLYER 00170 5040, // CPI_MAX 00171 0, // CPI_MIN 00172 90, // CPI_STEP 00173 0, // CPI_Z 00174 0, // CPI_H 00175 360, // CPI_HR_X 00176 360, // CPI_HR_Y 00177 BUTTON_LEFT, 00178 BUTTON_MIDDLE, 00179 BUTTON_RIGHT, 00180 BUTTON_Z, 00181 BUTTON_HIGH_RES, 00182 BUTTON_FORWORD, 00183 BUTTON_BACK, 00184 0x00, // LED_ACTION 00185 0x192f, // VID (No default, must be set) 00186 0x0000, // PID (No default, must be set) 00187 0x00, // PROFILE_DEFAULT 00188 0x00, // PROFILE_CURRENT 00189 0x0000, // RELEASE 00190 0xffff, // ADNS_CRC (No default, must be set) 00191 0xffff, // ADNS_ID (No default, must be set) 00192 0xffff, // ADNS_FW_LEN (No default, must be set) 00193 0xea60 // ADNS_FW_OFFSET 00194 }; 00195 00196 00197 00198 void track( Ser25LCxxx *eeprom ); 00199 void program( Ser25LCxxx *eeprom ); 00200 00201 void motionCallback( void ); 00202 00203 void btn_hr_press( void ); 00204 void btn_hr_release( void ); 00205 00206 void btn_z_press( void ); 00207 void btn_z_release( void ); 00208 00209 void btn_l_press(void); 00210 void btn_l_release( void ); 00211 00212 void btn_m_press( void ); 00213 void btn_m_release( void ); 00214 00215 void btn_r_press( void ); 00216 void btn_r_release( void ); 00217 00218 void btn_f_press( void ); 00219 void btn_f_release( void ); 00220 00221 void btn_b_press( void ); 00222 void btn_b_release( void ); 00223 00224 void prfl_a_set( void ); 00225 void prfl_b_set( void ); 00226 void prfl_c_set( void ); 00227 void prfl_d_set( void ); 00228 void prfl_e_set( void ); 00229 00230 void prfl_stub( void ); 00231 void debug_out(void); 00232 00233 int set_setting( Ser25LCxxx *eeprom, uint16_t attrib, uint16_t val, uint16_t base_address ); 00234 uint16_t get_setting( Ser25LCxxx *eeprom, uint16_t attrib, uint16_t base_address ); 00235 void clear_setting( Ser25LCxxx *eeprom, uint16_t attrib, uint16_t base_address ); 00236 00237 void load_data( Ser25LCxxx *eeprom, uint16_t base, uint16_t len, const uint8_t* data ); 00238 00239 uint8_t* get_data( Ser25LCxxx *eeprom, uint16_t base, uint16_t len );
Generated on Thu Jul 14 2022 10:56:43 by
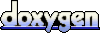