
Own fork of MbedSmartRestMain
Dependencies: C027_Support C12832 LM75B MMA7660 MbedSmartRest mbed-rtos mbed
Fork of MbedSmartRestMain by
ReportThread.h
00001 #ifndef REPORTTHREAD_H 00002 #define REPORTTHREAD_H 00003 #include "Operation.h" 00004 #include "SmartRestConf.h" 00005 #include "SmartRestSocket.h" 00006 #define OPERATION_DICT_SIZE 10 00007 00008 class OperationDict 00009 { 00010 public: 00011 OperationDict(): count(0) {} 00012 const Operation& operator [](unsigned short i) const { return opl[i]; } 00013 Operation *set(long id, OperationState state) { 00014 short i = count-1; 00015 for (; i >= 0; --i) { 00016 if (opl[i].identifier == id) 00017 break; 00018 } 00019 if (i >= 0) { 00020 opl[i].identifier = id; 00021 opl[i].state = state; 00022 return &opl[i]; 00023 } else if (count < OPERATION_DICT_SIZE) { 00024 i = count++; 00025 opl[i].identifier = id; 00026 opl[i].state = state; 00027 return &opl[i]; 00028 } else 00029 return NULL; 00030 } 00031 void clear() { count = 0; } 00032 bool full() const { return count >= OPERATION_DICT_SIZE; } 00033 unsigned short size() const { return count; } 00034 virtual ~OperationDict() {} 00035 private: 00036 unsigned short count; 00037 Operation opl[OPERATION_DICT_SIZE]; 00038 }; 00039 00040 class ReportThread 00041 { 00042 public: 00043 ReportThread(OperationPool& pool) : ipool(pool), dict(), sock(), 00044 thread(ReportThread::threadWrapper, this) { 00045 strncpy(uri, "/s", sizeof(uri)); 00046 } 00047 virtual ~ReportThread() { } 00048 void threadFunc(); 00049 static void threadWrapper(const void *p) { ((ReportThread*)p)->threadFunc(); } 00050 private: 00051 char uri[4]; 00052 OperationPool& ipool; 00053 OperationDict dict; 00054 char buf[SMARTREST_SIZE]; 00055 char buf2[SMARTREST_BODY_SIZE]; 00056 SmartRestSocket sock; 00057 Thread thread; 00058 }; 00059 00060 #endif /* REPORTTHREAD_H */
Generated on Sat Jul 16 2022 07:10:59 by
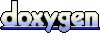