Own fork of MbedSmartRest
Dependents: MbedSmartRestMain MbedSmartRestMain
Fork of MbedSmartRest by
AbstractSmartRest.h
00001 #ifndef ABSTRACTSMARTREST_H 00002 #define ABSTRACTSMARTREST_H 00003 00004 #include "config.h" 00005 #include <stddef.h> 00006 #include <stdint.h> 00007 #include "DataGenerator.h" 00008 #include "ParsedRecord.h" 00009 #ifdef SMARTREST_TRANSACTIONAL 00010 #include "Aggregator.h" 00011 #endif 00012 00013 /** Return value indicating that no error occurred. */ 00014 #define SMARTREST_SUCCESS 0 00015 /** Return value indicating that the connection has been closed during 00016 * data transmission. */ 00017 #define SMARTREST_CONNECTION_FAILED 1 00018 /** Return value indicating an internal state error. */ 00019 #define SMARTREST_INTERNAL_ERROR 2 00020 /** Return value indicating a transmission timeout. */ 00021 #define SMARTREST_TIMEOUT_ERROR 3 00022 /** Return value indicating an end of response indicated by the 00023 * Content-Length header. */ 00024 #define SMARTREST_END_OF_RESPONSE 4 00025 /** Return value indicating that the connection has been closed. */ 00026 #define SMARTREST_CONNECTION_CLOSED 5 00027 00028 /** 00029 * Abstract SmartRest facade class. 00030 * This class provides methods to send a request and receive a response 00031 * from the server. 00032 * 00033 * Example: 00034 * @code 00035 * // given a concrete SmartRest implementation and a template 00036 * SmartRest client; 00037 * StaticData template; 00038 * 00039 * // bootstrap 00040 * if (client.bootstrap(template) != SMARTREST_SUCCESS) { 00041 * // error handling 00042 * return; 00043 * } 00044 * 00045 * if (client.send(StaticData("100,Hello")) != SMARTREST_SUCCESS) { 00046 * // error handling 00047 * } 00048 * 00049 * uint8_t ret; 00050 * while ((ret = client.receive(record)) == SMARTREST_SUCCESS) { 00051 * // work with data 00052 * } 00053 * 00054 * // error handling 00055 * 00056 * // call after every request. 00057 * client.stop(); 00058 * @encode 00059 */ 00060 class AbstractSmartRest 00061 { 00062 public: 00063 virtual ~AbstractSmartRest() { }; 00064 00065 #ifdef SMARTREST_TRANSACTIONAL 00066 /** 00067 * Performs a SmartRest request without receiving any data from the 00068 * server. 00069 * @param generator the generator which will generate the data to be 00070 * sent as a template. 00071 * @param overrideIdentifier a device identifier which gets sent instead 00072 * of the identifier specified in the 00073 * constructor. If an empty string is 00074 * specified, no identifier is sent at all. 00075 * @return a non-zero value if and only if an error occured 00076 */ 00077 virtual uint8_t request(const DataGenerator&, const char* = NULL) = 0; 00078 00079 /** 00080 * Performs a SmartRest request. 00081 * @param generator the generator which will generate the data to be 00082 * sent as a template. 00083 * @param aggregator the aggregator where all received records will 00084 * be stored. The aggregator must be managed, 00085 * otherwise an error will be returned. 00086 * @param overrideIdentifier a device identifier which gets sent instead 00087 * of the identifier specified in the 00088 * constructor. If an empty string is 00089 * specified, no identifier is sent at all. 00090 * @return a non-zero value if and only if an error occured 00091 */ 00092 virtual uint8_t request(const DataGenerator&, Aggregator&, const char* = NULL) = 0; 00093 #endif 00094 00095 /* 00096 * Initiates the SmartRest bootstrap process. 00097 * When successful, the template identifier will be replaced by the 00098 * global managed object ID in future requests. 00099 * @param generator the generator which will generate the data to be 00100 * sent as a template. 00101 * @return a non-zero value if and only if an error occured 00102 */ 00103 virtual uint8_t bootstrap(const DataGenerator&) = 0; 00104 00105 /** 00106 * Sends a smart request. 00107 * @param generator the generator which will generate the data to be 00108 * sent. 00109 * @param overrideIdentifier a device identifier which gets sent instead 00110 * of the identifier specified in the 00111 * constructor. If an empty string is 00112 * specified, no identifier is sent at all. 00113 * @return a non-zero value if and only if an error occured 00114 */ 00115 virtual uint8_t send(const DataGenerator&, const char* = NULL) = 0; 00116 00117 /** 00118 * Same functionality and return value as the above, except the connection 00119 * is closed immediately after sending, do NOT wait for response. 00120 */ 00121 virtual uint8_t sendAndClose(const DataGenerator&, const char* = NULL) = 0; 00122 00123 /** 00124 * Starts a SmartRest stream. 00125 * @param uri the stream endpoint URI 00126 * @param record the record which will be sent to the endpoint 00127 * @param overrideIdentifier a device identifier which gets sent instead 00128 * of the identifier specified in the 00129 * constructor. If an empty string is 00130 * specified, no identifier is sent at all. 00131 * @return a non-zero value if and only if an error occured 00132 */ 00133 virtual uint8_t stream(const char*, const Record&, const char* = NULL) = 0; 00134 00135 /** 00136 * Tries to receive a parsed response row. 00137 * When the function succeeds, but the row pointer is NULL, there are 00138 * no more rows to be read. 00139 * @param record an instance to where the parsed row is written 00140 * @return a non-zero value if and only if an error occured 00141 */ 00142 virtual uint8_t receive(ParsedRecord&) = 0; 00143 00144 /* 00145 * Closes the connection. 00146 */ 00147 virtual void stop() = 0; 00148 }; 00149 00150 #endif
Generated on Tue Jul 12 2022 17:00:00 by
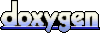