The Library of SNTP Client for W5500 SNTP is short for Simple Network Time Protocol.
Dependents: SNTP_Ethernet_W5500 SNTP_Ethernet_W5500 SNTP_SHT15_WIZwizki-W7500 Nucleo_SNTP_Ethernet_W5500 ... more
SNTPClient.h
00001 /** 00002 * @author Raphael Kwon 00003 * 00004 * @section LICENSE 00005 * 00006 * Copyright (c) 2014 WIZnet Co., Ltd. 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy 00009 * of this software and associated documentation files (the "Software"), to deal 00010 * in the Software without restriction, including without limitation the rights 00011 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00012 * copies of the Software, and to permit persons to whom the Software is 00013 * furnished to do so, subject to the following conditions: 00014 * 00015 * The above copyright notice and this permission notice shall be included in 00016 * all copies or substantial portions of the Software. 00017 * 00018 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00019 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00020 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00021 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00022 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00023 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00024 * THE SOFTWARE. 00025 * 00026 * @section DESCRIPTION 00027 * Simple Network Time Protocol Client 00028 * 00029 */ 00030 00031 #ifndef SNTPCLIENT_H 00032 #define SNTPCLIENT_H 00033 00034 #include "mbed.h" 00035 #include "UDPSocket.h" 00036 00037 /* 00038 * @brief Define it for Debug & Monitor DNS processing. 00039 * @note 00040 */ 00041 //#define _SNTP_DEBUG_ 00042 00043 #define MAX_SNTP_BUF_SIZE sizeof(ntpformat) ///< maximum size of DNS buffer. */ 00044 #define ntp_port 123 //ntp server port number 00045 #define SECS_PERDAY 86400UL // seconds in a day = 60*60*24 00046 #define UTC_ADJ_HRS 9 // SEOUL : GMT+9 00047 #define EPOCH 1900 // NTP start year 00048 00049 /* for ntpclient */ 00050 typedef signed char s_char; 00051 typedef unsigned long long tstamp; 00052 typedef unsigned int tdist; 00053 00054 typedef struct _ntpformat 00055 { 00056 uint8_t dstaddr[4]; /* destination (local) address */ 00057 char version; /* version number */ 00058 char leap; /* leap indicator */ 00059 char mode; /* mode */ 00060 char stratum; /* stratum */ 00061 char poll; /* poll interval */ 00062 s_char precision; /* precision */ 00063 tdist rootdelay; /* root delay */ 00064 tdist rootdisp; /* root dispersion */ 00065 char refid; /* reference ID */ 00066 tstamp reftime; /* reference time */ 00067 tstamp org; /* origin timestamp */ 00068 tstamp rec; /* receive timestamp */ 00069 tstamp xmt; /* transmit timestamp */ 00070 } ntpformat; 00071 00072 typedef struct _datetime 00073 { 00074 uint16_t yy; 00075 uint8_t mo; 00076 uint8_t dd; 00077 uint8_t hh; 00078 uint8_t mm; 00079 uint8_t ss; 00080 } datetime; 00081 00082 /** SNTPClient client Class. 00083 * 00084 * Example (ethernet network): 00085 * @code 00086 * #include "mbed.h" 00087 * #include "EthernetInterface.h" 00088 * #include "SNTPClient.h" 00089 * 00090 * int main() { 00091 * EthernetInterface eth; 00092 * eth.init(); //Use DHCP 00093 * eth.connect(); 00094 * printf("IP Address is %s\n\r", eth.getIPAddress()); 00095 * 00096 * SNTPClient ws("ws://sockets.mbed.org:443/ws/demo/rw"); 00097 * ws.connect(); 00098 * 00099 * while (1) { 00100 * int res = ws.send("SNTPClient Hello World!"); 00101 * 00102 * if (ws.read(recv)) { 00103 * printf("rcv: %s\r\n", recv); 00104 * } 00105 * 00106 * wait(0.1); 00107 * } 00108 * } 00109 * @endcode 00110 */ 00111 00112 00113 class SNTPClient 00114 { 00115 public: 00116 /** 00117 * Constructor 00118 * 00119 * @param url The SNTPClient host 00120 */ 00121 SNTPClient(char * url, uint8_t time_zone); 00122 00123 /** 00124 * Connect to the SNTPClient url 00125 * 00126 *@return true if the connection is established, false otherwise 00127 */ 00128 bool connect(); 00129 00130 /** 00131 * Read a SNTPClient message 00132 * 00133 * @param message pointer to the string to be read (null if drop frame) 00134 * 00135 * @return true if a SNTPClient frame has been read 00136 */ 00137 bool getTime(datetime *time); 00138 00139 /** 00140 * Close the SNTPClient connection 00141 * 00142 * @return true if the connection has been closed, false otherwise 00143 */ 00144 bool close(); 00145 00146 /* 00147 * Accessor: get host from the SNTPClient url 00148 * 00149 * @return host 00150 */ 00151 char* getHost(); 00152 00153 private: 00154 00155 uint16_t port; 00156 char host[32]; 00157 00158 UDPSocket socket; 00159 Endpoint sntp_server; 00160 00161 ntpformat NTPformat; 00162 datetime Nowdatetime; 00163 uint8_t ntpmessage[48]; 00164 uint8_t tz; // Time Zone 00165 00166 void get_seconds_from_ntp_server(uint8_t *buf, uint16_t idx); 00167 tstamp changedatetime_to_seconds(void); 00168 void calcdatetime(tstamp seconds); 00169 }; 00170 00171 #endif
Generated on Sat Jul 16 2022 17:11:49 by
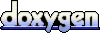