class of Buttons -isPressed -wasPressed -notPressed -LongPress (Timer)
Embed:
(wiki syntax)
Show/hide line numbers
Button.cpp
00001 #include "Button.h" 00002 #include "mbed.h" 00003 00004 00005 /******************************************************************************/ 00006 // constructor of instance button 00007 // - PinName: Name of pin of MBed LPC1768 (e.g. p9, p10,...) 00008 // - PinMode: PullUp, PullDown, PullNone, OpenDrain 00009 // - time: defines the time after the longPress-Flag is set. 00010 // 00011 /******************************************************************************/ 00012 Button::Button(PinName pBtn, PinMode pBtnMode, float time) 00013 { 00014 fTime = time; 00015 pin = new DigitalIn(pBtn, pBtnMode); 00016 00017 if(fTime > 0) 00018 timer = new Timeout; 00019 00020 init(); 00021 00022 } 00023 00024 00025 /******************************************************************************/ 00026 // initialisation 00027 // - bNewState: current state of button 00028 // - bOldState: previous state of button 00029 // - bIsPressed: flag is set if button is pressed 00030 // - bLongPresed: flag is set, after timer is zero (bIsPressed = true) 00031 // - bWasPressed: after button is not pressed anymore 00032 // 00033 /******************************************************************************/ 00034 void Button::init() 00035 { 00036 bNewState = false; 00037 bOldState = false; 00038 00039 bIsPressed = false; 00040 bLongPress = false; 00041 bWasPressed = false; 00042 00043 00044 bBtnNewAction = false; 00045 00046 iNumCycle = 0; 00047 if(fTime > 0) 00048 timer->detach(); 00049 00050 00051 return; 00052 } 00053 00054 00055 /******************************************************************************/ 00056 // query the Button with debouncing 00057 // - bNewState: current state of button 00058 // - bOldState: previous state of button 00059 // - bBtnNewAction: flag, if an action is occur 00060 // - iNumCycle: cycle counter of same state 00061 // - NUNCYCLES: max. value of cycle counter 00062 // - *iNumBtn: pointer to variable, which counts the number of pressed buttons 00063 // 00064 /******************************************************************************/ 00065 void Button::polling(uint8_t *iNumBtn) 00066 { 00067 bOldState = bNewState; // bNewState gets the becomes the bOldstate 00068 bNewState = (bool)pin->read(); // update bNewState 00069 bWasPressed = false; // reset the flag was pressed 00070 00071 00072 if(!bBtnNewAction and (bOldState != bNewState)) // if there is a action (changing signal) on the button (btn), then rise the flag bBtnNewAction 00073 bBtnNewAction = true; 00074 00075 00076 if(bBtnNewAction) { // if flag ist true (new action on the button) 00077 00078 if(bOldState == bNewState) { // if current state equals previous state 00079 if(iNumCycle <= NUMCYCLE) { // count the number of cycle unless the max. value is reached 00080 iNumCycle++; 00081 00082 } else if(iNumCycle > NUMCYCLE) { // else if the max. value is reached 00083 iNumCycle = 0; // reset the counter, there is no action any more expected 00084 bBtnNewAction = false; // there is nomore new action. 00085 00086 if(bNewState == PRESSED) { // btn is pressed, then 00087 bIsPressed = true; // set this flag true 00088 00089 if(iNumBtn != NULL) 00090 (*iNumBtn)++; 00091 00092 if(fTime > 0) // if btn has a timer, 00093 timer->attach(callback(this, &Button::setLongPressFlag), fTime); 00094 00095 00096 } else if(bNewState == NOT_PRESSED) { // if btn is not pressed, then 00097 if(bIsPressed == true) // if btn was pressed, then 00098 bWasPressed = true; // set this flag true 00099 00100 bIsPressed = false; // set this flag false 00101 00102 if(fTime > 0) // if btn has a timer, 00103 timer->detach(); 00104 bLongPress = false; 00105 00106 if(iNumBtn != NULL && *iNumBtn > 0) // if there is a pointer to a counter-Variable and the value is greater than 0 00107 (*iNumBtn)--; // decrease iNumBtn by one 00108 } 00109 00110 } 00111 } else { 00112 iNumCycle = 0; 00113 } 00114 } 00115 00116 return; 00117 } 00118 00119 00120 /******************************************************************************/ 00121 // 00122 /******************************************************************************/ 00123 bool Button::isPressed() 00124 { 00125 if(bIsPressed) return true; 00126 else return false; 00127 } 00128 00129 00130 /******************************************************************************/ 00131 // 00132 /******************************************************************************/ 00133 bool Button::isLongPress() 00134 { 00135 if(bLongPress) return true; 00136 else return false; 00137 00138 } 00139 00140 00141 /******************************************************************************/ 00142 // 00143 /******************************************************************************/ 00144 bool Button::wasPressed() 00145 { 00146 if(bWasPressed) return true; 00147 else return false; 00148 00149 } 00150 00151 00152 /******************************************************************************/ 00153 // 00154 /******************************************************************************/ 00155 void Button::setLongPressFlag(){ 00156 bLongPress = true; 00157 timer->detach(); 00158 } 00159 00160
Generated on Wed Jul 13 2022 20:48:54 by
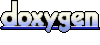