updates
Dependencies: BLE_API mbed-dev-bin nRF51822
Fork of microbit-dal-eddystone by
MicroBitBLEManager.cpp
00001 /* 00002 The MIT License (MIT) 00003 00004 Copyright (c) 2016 British Broadcasting Corporation. 00005 This software is provided by Lancaster University by arrangement with the BBC. 00006 00007 Permission is hereby granted, free of charge, to any person obtaining a 00008 copy of this software and associated documentation files (the "Software"), 00009 to deal in the Software without restriction, including without limitation 00010 the rights to use, copy, modify, merge, publish, distribute, sublicense, 00011 and/or sell copies of the Software, and to permit persons to whom the 00012 Software is furnished to do so, subject to the following conditions: 00013 00014 The above copyright notice and this permission notice shall be included in 00015 all copies or substantial portions of the Software. 00016 00017 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL 00020 THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING 00022 FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER 00023 DEALINGS IN THE SOFTWARE. 00024 */ 00025 00026 00027 00028 #include "MicroBitConfig.h" 00029 #include "MicroBitBLEManager.h" 00030 #include "MicroBitEddystone.h" 00031 #include "MicroBitStorage.h" 00032 #include "MicroBitFiber.h" 00033 #include "MicroBitSystemTimer.h" 00034 00035 /* The underlying Nordic libraries that support BLE do not compile cleanly with the stringent GCC settings we employ. 00036 * If we're compiling under GCC, then we suppress any warnings generated from this code (but not the rest of the DAL) 00037 * The ARM cc compiler is more tolerant. We don't test __GNUC__ here to detect GCC as ARMCC also typically sets this 00038 * as a compatability option, but does not support the options used... 00039 */ 00040 #if !defined(__arm) 00041 #pragma GCC diagnostic ignored "-Wunused-function" 00042 #pragma GCC diagnostic push 00043 #pragma GCC diagnostic ignored "-Wunused-parameter" 00044 #endif 00045 00046 #include "ble.h" 00047 00048 extern "C" { 00049 #include "device_manager.h" 00050 uint32_t btle_set_gatt_table_size(uint32_t size); 00051 } 00052 00053 /* 00054 * Return to our predefined compiler settings. 00055 */ 00056 #if !defined(__arm) 00057 #pragma GCC diagnostic pop 00058 #endif 00059 00060 #define MICROBIT_PAIRING_FADE_SPEED 4 00061 00062 // Some Black Magic to compare the definition of our security mode in MicroBitConfig with a given parameter. 00063 // Required as the MicroBitConfig option is actually an mbed enum, that is not normally comparable at compile time. 00064 // 00065 00066 #define __CAT(a, ...) a##__VA_ARGS__ 00067 00068 00069 const char *MICROBIT_BLE_MANUFACTURER = NULL; 00070 const char *MICROBIT_BLE_MODEL = "BBC micro:bit"; 00071 const char *MICROBIT_BLE_HARDWARE_VERSION = NULL; 00072 const char *MICROBIT_BLE_FIRMWARE_VERSION = MICROBIT_DAL_VERSION; 00073 const char *MICROBIT_BLE_SOFTWARE_VERSION = NULL; 00074 const int8_t MICROBIT_BLE_POWER_LEVEL[] = {-30, -20, -16, -12, -8, -4, 0, 4}; 00075 00076 /* 00077 * Many of the mbed interfaces we need to use only support callbacks to plain C functions, rather than C++ methods. 00078 * So, we maintain a pointer to the MicroBitBLEManager that's in use. Ths way, we can still access resources on the micro:bit 00079 * whilst keeping the code modular. 00080 */ 00081 MicroBitBLEManager *MicroBitBLEManager::manager = NULL; // Singleton reference to the BLE manager. many mbed BLE API callbacks still do not support member funcions yet. :-( 00082 00083 00084 00085 /** 00086 * Constructor. 00087 * Configure and manage the micro:bit's Bluetooth Low Energy (BLE) stack. 00088 * @param _storage an instance of MicroBitStorage used to persist sys attribute information. (This is required for compatability with iOS). 00089 * @note The BLE stack *cannot* be brought up in a static context (the software simply hangs or corrupts itself). 00090 * Hence, the init() member function should be used to initialise the BLE stack. 00091 */ 00092 MicroBitBLEManager::MicroBitBLEManager(MicroBitStorage &_storage) : storage(&_storage) 00093 { 00094 manager = this; 00095 this->ble = NULL; 00096 this->pairingStatus = 0; 00097 } 00098 00099 /** 00100 * Constructor. 00101 * Configure and manage the micro:bit's Bluetooth Low Energy (BLE) stack. 00102 * @note The BLE stack *cannot* be brought up in a static context (the software simply hangs or corrupts itself). 00103 * Hence, the init() member function should be used to initialise the BLE stack. 00104 */ 00105 MicroBitBLEManager::MicroBitBLEManager() : storage(NULL) 00106 { 00107 manager = this; 00108 this->ble = NULL; 00109 this->pairingStatus = 0; 00110 } 00111 00112 /** 00113 * When called, the micro:bit will begin advertising for a predefined period, 00114 * MICROBIT_BLE_ADVERTISING_TIMEOUT seconds to bonded devices. 00115 */ 00116 MicroBitBLEManager *MicroBitBLEManager::getInstance() 00117 { 00118 if (manager == 0) 00119 { 00120 manager = new MicroBitBLEManager; 00121 } 00122 return manager; 00123 } 00124 00125 /** 00126 * When called, the micro:bit will begin advertising for a predefined period, 00127 * MICROBIT_BLE_ADVERTISING_TIMEOUT seconds to bonded devices. 00128 */ 00129 void MicroBitBLEManager::advertise() 00130 { 00131 if (ble) 00132 ble->gap().startAdvertising(); 00133 } 00134 00135 /** 00136 * Post constructor initialisation method as the BLE stack cannot be brought 00137 * up in a static context. 00138 * @param deviceName The name used when advertising 00139 * @param serialNumber The serial number exposed by the device information service 00140 * @param messageBus An instance of an EventModel, used during pairing. 00141 * @param enableBonding If true, the security manager enabled bonding. 00142 * @code 00143 * bleManager.init(uBit.getName(), uBit.getSerial(), uBit.messageBus, true); 00144 * @endcode 00145 */ 00146 void MicroBitBLEManager::init(ManagedString deviceName, ManagedString serialNumber, EventModel &messageBus, bool enableBonding) 00147 { 00148 ManagedString BLEName("BBC micro:bit"); 00149 this->deviceName = deviceName; 00150 00151 //wlw #if !(CONFIG_ENABLED(MICROBIT_BLE_WHITELIST)) 00152 ManagedString namePrefix(" ["); 00153 ManagedString namePostfix("]"); 00154 BLEName = BLEName + namePrefix + deviceName + namePostfix; 00155 //wlw #endif 00156 00157 // Start the BLE stack. 00158 #if CONFIG_ENABLED(MICROBIT_HEAP_REUSE_SD) 00159 btle_set_gatt_table_size(MICROBIT_SD_GATT_TABLE_SIZE); 00160 #endif 00161 00162 ble = new BLEDevice(); 00163 ble->init(); 00164 00165 00166 // Configure the stack to hold onto the CPU during critical timing events. 00167 // mbed-classic performs __disable_irq() calls in its timers that can cause 00168 // MIC failures on secure BLE channels... 00169 ble_common_opt_radio_cpu_mutex_t opt; 00170 opt.enable = 1; 00171 sd_ble_opt_set(BLE_COMMON_OPT_RADIO_CPU_MUTEX, (const ble_opt_t *)&opt); 00172 00173 00174 00175 // Configure the radio at our default power level 00176 setTransmitPower(MICROBIT_BLE_DEFAULT_TX_POWER); 00177 00178 // Bring up core BLE services. 00179 #if CONFIG_ENABLED(MICROBIT_BLE_DFU_SERVICE) 00180 new MicroBitDFUService(*ble); 00181 #endif 00182 00183 #if CONFIG_ENABLED(MICROBIT_BLE_DEVICE_INFORMATION_SERVICE) 00184 DeviceInformationService ble_device_information_service(*ble, MICROBIT_BLE_MANUFACTURER, MICROBIT_BLE_MODEL, serialNumber.toCharArray(), MICROBIT_BLE_HARDWARE_VERSION, MICROBIT_BLE_FIRMWARE_VERSION, MICROBIT_BLE_SOFTWARE_VERSION); 00185 #else 00186 (void)serialNumber; 00187 #endif 00188 00189 #if CONFIG_ENABLED(MICROBIT_BLE_EVENT_SERVICE) 00190 new MicroBitEventService(*ble, messageBus); 00191 #else 00192 (void)messageBus; 00193 #endif 00194 00195 00196 // Setup advertising. 00197 #if CONFIG_ENABLED(MICROBIT_BLE_WHITELIST) 00198 ble->accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED); 00199 #else 00200 ble->accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00201 #endif 00202 00203 ble->accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)BLEName.toCharArray(), BLEName.length()); 00204 ble->setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00205 ble->setAdvertisingInterval(200); 00206 00207 #if (MICROBIT_BLE_ADVERTISING_TIMEOUT > 0) 00208 ble->gap().setAdvertisingTimeout(MICROBIT_BLE_ADVERTISING_TIMEOUT); 00209 #endif 00210 00211 } 00212 00213 00214 /** 00215 * Change the output power level of the transmitter to the given value. 00216 * @param power a value in the range 0..7, where 0 is the lowest power and 7 is the highest. 00217 * @return MICROBIT_OK on success, or MICROBIT_INVALID_PARAMETER if the value is out of range. 00218 * @code 00219 * // maximum transmission power. 00220 * bleManager.setTransmitPower(7); 00221 * @endcode 00222 */ 00223 int MicroBitBLEManager::setTransmitPower(int power) 00224 { 00225 if (power < 0 || power >= MICROBIT_BLE_POWER_LEVELS) 00226 return MICROBIT_INVALID_PARAMETER; 00227 00228 if (ble->gap().setTxPower(MICROBIT_BLE_POWER_LEVEL[power]) != NRF_SUCCESS) 00229 return MICROBIT_NOT_SUPPORTED; 00230 00231 return MICROBIT_OK; 00232 } 00233 00234 00235 00236 00237 /** 00238 * Periodic callback in thread context. 00239 * We use this here purely to safely issue a disconnect operation after a pairing operation is complete. 00240 */ 00241 void MicroBitBLEManager::idleTick() 00242 { 00243 00244 } 00245 00246 00247 /** 00248 * Stops any currently running BLE advertisements 00249 */ 00250 void MicroBitBLEManager::stopAdvertising() 00251 { 00252 ble->gap().stopAdvertising(); 00253 } 00254 00255 #if CONFIG_ENABLED(MICROBIT_BLE_EDDYSTONE_URL) 00256 /** 00257 * Set the content of Eddystone URL frames 00258 * @param url The url to broadcast 00259 * @param calibratedPower the transmission range of the beacon (Defaults to: 0xF0 ~10m). 00260 * @param connectable true to keep bluetooth connectable for other services, false otherwise. (Defaults to true) 00261 * @param interval the rate at which the micro:bit will advertise url frames. (Defaults to MICROBIT_BLE_EDDYSTONE_ADV_INTERVAL) 00262 * @note The calibratedPower value ranges from -100 to +20 to a resolution of 1. The calibrated power should be binary encoded. 00263 * More information can be found at https://github.com/google/eddystone/tree/master/eddystone-uid#tx-power 00264 */ 00265 int MicroBitBLEManager::advertiseEddystoneUrl(const char* url, int8_t calibratedPower, bool connectable, uint16_t interval) 00266 { 00267 ble->gap().stopAdvertising(); 00268 ble->clearAdvertisingPayload(); 00269 00270 ble->setAdvertisingType(connectable ? GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED : GapAdvertisingParams::ADV_NON_CONNECTABLE_UNDIRECTED); 00271 ble->setAdvertisingInterval(interval); 00272 00273 ble->accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00274 00275 int ret = MicroBitEddystone::getInstance()->setURL(ble, url, calibratedPower); 00276 00277 #if (MICROBIT_BLE_ADVERTISING_TIMEOUT > 0) 00278 ble->gap().setAdvertisingTimeout(MICROBIT_BLE_ADVERTISING_TIMEOUT); 00279 #endif 00280 ble->gap().startAdvertising(); 00281 00282 return ret; 00283 } 00284 00285 /** 00286 * Set the content of Eddystone URL frames, but accepts a ManagedString as a url. 00287 * @param url The url to broadcast 00288 * @param calibratedPower the transmission range of the beacon (Defaults to: 0xF0 ~10m). 00289 * @param connectable true to keep bluetooth connectable for other services, false otherwise. (Defaults to true) 00290 * @param interval the rate at which the micro:bit will advertise url frames. (Defaults to MICROBIT_BLE_EDDYSTONE_ADV_INTERVAL) 00291 * @note The calibratedPower value ranges from -100 to +20 to a resolution of 1. The calibrated power should be binary encoded. 00292 * More information can be found at https://github.com/google/eddystone/tree/master/eddystone-uid#tx-power 00293 */ 00294 int MicroBitBLEManager::advertiseEddystoneUrl(ManagedString url, int8_t calibratedPower, bool connectable, uint16_t interval) 00295 { 00296 return advertiseEddystoneUrl((char *)url.toCharArray(), calibratedPower, connectable, interval); 00297 } 00298 #endif 00299 00300 00301 void MicroBitBLEManager::pairingMode(MicroBitDisplay &display, MicroBitButton &authorisationButton) 00302 { 00303 00304 00305 } 00306 00307
Generated on Tue Jul 12 2022 21:39:18 by
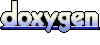