
Demo of the Cmdb Command Interpreter. Replaces the old cmbd demo as it had name clashes on this website.
main.cpp
00001 #include <vector> 00002 00003 #include "mbed.h" 00004 #include "cmdb.h" 00005 00006 DigitalOut myled(LED1); 00007 00008 //We'll be using the Usb Serial port 00009 Serial serial(USBTX, USBRX); //tx, rx 00010 00011 #define CID_TEST (int)1 00012 #define CID_INT (int)2 00013 00014 /** Sample User Command Dispatcher. 00015 * 00016 * @parm cmdb the command interpreter object. 00017 * @parm cid the command id. 00018 */ 00019 void my_dispatcher(Cmdb& cmdb, int cid) { 00020 cmdb.printf("my_dispatcher: cid=%d\r\n", cid); 00021 00022 switch (cid) { 00023 case CID_INT : 00024 cmdb.printf("my_dispatcher: parm 0=%d\r\n",cmdb.INTPARM(0)); 00025 break; 00026 } 00027 } 00028 00029 static const cmd c1 = {"Test",SUBSYSTEM,CID_TEST,"" ,"* Test Subsystem"}; 00030 static const cmd c2 = {"Int" ,CID_TEST ,CID_INT ,"%i","* Int as parameter" ,"dummy"}; 00031 00032 int main() { 00033 // Set the Baudrate. 00034 serial.baud(115200); 00035 00036 // Test the serial connection by 00037 serial.printf("\r\n\r\nCmdb Command Interpreter Demo Version %0.2f.\r\n\r\n", Cmdb::version()); 00038 00039 //Create a Command Table Vector. 00040 std::vector<cmd> cmds; 00041 00042 //Add some of our own first... 00043 cmds.push_back(c1); //Test Subsystem is handled by Cmdb internally. 00044 cmds.push_back(c2); //The Int Command is handled by our 'my_dispatcher' method. 00045 00046 //Add some predefined... 00047 cmds.push_back(COMMANDS); //Handled by Cmdb internally. 00048 cmds.push_back(BOOT); //Handled by Cmdb internally. 00049 00050 cmds.push_back(ECHO); //Handled by Cmdb internally. 00051 cmds.push_back(BOLD); //Handled by Cmdb internally. 00052 cmds.push_back(CLS); //Handled by Cmdb internally. 00053 00054 cmds.push_back(MACRO); //Handled by Cmdb internally. 00055 cmds.push_back(RUN); //Handled by Cmdb internally. 00056 cmds.push_back(MACROS); //Handled by Cmdb internally. 00057 00058 //Add some predefined and mandatory... 00059 cmds.push_back(IDLE); //Handled by Cmdb internally. 00060 cmds.push_back(HELP); //Handled by Cmdb internally. 00061 00062 //Create and initialize the Command Interpreter. 00063 Cmdb cmdb(serial, cmds, &my_dispatcher); 00064 00065 cmdb.printf("%d=%d\r\n",cmds[0].subs,cmds[0].cid); 00066 cmdb.printf("%d=%d\r\n",cmds[1].subs,cmds[1].cid); 00067 00068 while (1) { 00069 //Check for input... 00070 if (cmdb.hasnext()==true) { 00071 00072 //Supply input to Command Interpreter 00073 if (cmdb.scan(cmdb.next())) { 00074 00075 //Flash led when a command has been parsed and dispatched. 00076 myled = 1; 00077 wait(0.2); 00078 00079 //cmdb.print("Command Parsed and Dispatched\r\n"); 00080 00081 myled = 0; 00082 wait(0.2); 00083 } 00084 } 00085 00086 //For Macro Support we basically do the same but take characters from the macro buffer. 00087 //Example Macro: Test|Int_42|Idle 00088 while (cmdb.macro_hasnext()) { 00089 //Get and process next character. 00090 cmdb.scan(cmdb.macro_next()); 00091 00092 //After the last character we need to add a cr to force execution. 00093 if (!cmdb.macro_peek()) { 00094 cmdb.scan(cr); 00095 } 00096 } 00097 } 00098 }
Generated on Mon Jul 18 2022 10:38:06 by
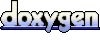