
Client TCP example with scket. Starting point for TLS example with wolfSSL, client-tls
Dependencies: EthernetInterface mbed-rtos mbed
client-tcp.cpp
00001 /* client-tcp.c 00002 * 00003 * Copyright (C) 2006-2015 wolfSSL Inc. 00004 * 00005 * This file is part of wolfSSL. (formerly known as CyaSSL) 00006 * 00007 * wolfSSL is free software; you can redistribute it and/or modify 00008 * it under the terms of the GNU General Public License as published by 00009 * the Free Software Foundation; either version 2 of the License, or 00010 * (at your option) any later version. 00011 * 00012 * wolfSSL is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 * GNU General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU General Public License 00018 * along with this program; if not, write to the Free Software 00019 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA 00020 */ 00021 00022 #include "mbed.h" 00023 #include "EthernetInterface.h" 00024 #include <stdio.h> 00025 #include <stdlib.h> 00026 #include <string.h> 00027 00028 #define MAXDATASIZE (1024*4) 00029 00030 static int getline(char *prompt, char *buff, int size) 00031 { 00032 int sz ; 00033 00034 printf("%s", prompt) ; 00035 for(sz = 0 ; (sz < size) && ((*buff = getchar()) != '\r'); sz++, buff++) { 00036 putchar(*buff) ; 00037 if(*buff == '\\') { 00038 if(++sz >= size)break ; 00039 *buff = getchar() ; 00040 putchar(*buff) ; 00041 switch(*buff) { 00042 case 'n' : 00043 *buff = '\n' ; 00044 break ; 00045 case 'r' : 00046 *buff = '\r' ; 00047 break ; 00048 case 't' : 00049 *buff = '\t' ; 00050 break ; 00051 case '\\': 00052 *buff = '\\' ; 00053 break ; 00054 default: 00055 buff[1] = buff[0] ; 00056 buff[0] = '\\' ; 00057 buff++ ; 00058 } 00059 } else if(*buff == '\b') { 00060 if(sz >= 2) { 00061 buff-=2 ; 00062 sz-=2; 00063 } 00064 } 00065 } ; 00066 putchar('\n') ; 00067 *buff = '\0' ; 00068 return sz ; 00069 } 00070 00071 /* 00072 * clients initial contact with server. Socket to connect to: sock 00073 */ 00074 int ClientGreet(TCPSocketConnection *socket) 00075 { 00076 /* data to send to the server, data recieved from the server */ 00077 char sendBuff[MAXDATASIZE], rcvBuff[MAXDATASIZE] = {0}; 00078 int ret ; 00079 00080 ret = getline("Message for server: ", sendBuff, MAXDATASIZE); 00081 printf("Send[%d]:\n%s\n", ret, sendBuff) ; 00082 if ((ret = socket->send(sendBuff, strlen(sendBuff))) < 0) { 00083 printf("Send error: %i", ret); 00084 return EXIT_FAILURE; 00085 } 00086 printf("Recieved:\n"); 00087 while(1) { 00088 if ((ret = socket->receive(rcvBuff, sizeof(rcvBuff)-1)) < 0) { 00089 if(ret == 0)break ; 00090 printf("Read error. Error: %i\n", ret); 00091 return EXIT_FAILURE; 00092 } 00093 rcvBuff[ret] = '\0' ; 00094 printf("%s", rcvBuff); 00095 if((rcvBuff[ret-3] == '\n')&& 00096 (rcvBuff[ret-2] == '\n')&& 00097 (rcvBuff[ret-1] == '\n'))break ; 00098 } 00099 return ret; 00100 } 00101 00102 /* 00103 * command line argumentCount and argumentValues 00104 */ 00105 void net_main(const void *av) 00106 { 00107 char server_addr[40] ; 00108 char server_port[10] ; 00109 00110 EthernetInterface eth; 00111 TCPSocketConnection socket; 00112 00113 eth.init(); //Use DHCP 00114 while(1) { 00115 if(eth.connect() == 0)break ; 00116 printf("Retry\n") ; 00117 } 00118 printf("Client Addr: %s\n", eth.getIPAddress()); 00119 00120 getline("Server Addr: ", server_addr, sizeof(server_addr)) ; 00121 getline("Server Port: ", server_port, sizeof(server_port)) ; 00122 00123 while (socket.connect(server_addr, atoi(server_port)) < 0) { 00124 printf("Unable to connect to (%s) on port (%s)\n", server_addr, server_port); 00125 wait(1.0); 00126 } 00127 printf("TCP Connected\n") ; 00128 00129 ClientGreet(&socket); 00130 return ; 00131 } 00132 00133 int main(void) 00134 { 00135 #define STACK_SIZE 24000 00136 Thread t(net_main, NULL, osPriorityNormal, STACK_SIZE); 00137 while(1)wait(1.0) ; 00138 }
Generated on Mon Jul 18 2022 23:40:12 by
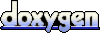