X_NUCLEO_IDB0XA1
Dependents: BLE_HeartRate_IDB0XA1
Fork of X_NUCLEO_IDB0XA1 by
BlueNRGGattServer.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 /** 00017 ****************************************************************************** 00018 * @file BlueNRGGattServer.cpp 00019 * @author STMicroelectronics 00020 * @brief Header file for BLE_API GattServer Class 00021 ****************************************************************************** 00022 * @copy 00023 * 00024 * THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS 00025 * WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE 00026 * TIME. AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY 00027 * DIRECT, INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING 00028 * FROM THE CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE 00029 * CODING INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS. 00030 * 00031 * <h2><center>© COPYRIGHT 2013 STMicroelectronics</center></h2> 00032 */ 00033 00034 #ifndef __BLUENRG_GATT_SERVER_H__ 00035 #define __BLUENRG_GATT_SERVER_H__ 00036 00037 #include "mbed.h" 00038 #include "ble/blecommon.h" 00039 #include "btle.h" 00040 #include "ble/GattService.h" 00041 #include "ble/GattServer.h" 00042 #include <vector> 00043 #include <map> 00044 00045 #define BLE_TOTAL_CHARACTERISTICS 10 00046 00047 using namespace std; 00048 00049 class BlueNRGGattServer : public GattServer 00050 { 00051 public: 00052 static BlueNRGGattServer &getInstance() { 00053 static BlueNRGGattServer m_instance; 00054 return m_instance; 00055 } 00056 00057 enum HandleEnum_t { 00058 CHAR_HANDLE = 0, 00059 CHAR_VALUE_HANDLE, 00060 CHAR_DESC_HANDLE 00061 }; 00062 00063 /* Functions that must be implemented from GattServer */ 00064 virtual ble_error_t addService(GattService &); 00065 virtual ble_error_t read(GattAttribute::Handle_t attributeHandle, uint8_t buffer[], uint16_t *lengthP); 00066 virtual ble_error_t read(Gap::Handle_t connectionHandle, GattAttribute::Handle_t attributeHandle, uint8_t buffer[], uint16_t *lengthP); 00067 virtual ble_error_t write(GattAttribute::Handle_t, const uint8_t[], uint16_t, bool localOnly = false); 00068 virtual ble_error_t write(Gap::Handle_t connectionHandle, GattAttribute::Handle_t, const uint8_t[], uint16_t, bool localOnly = false); 00069 virtual ble_error_t initializeGATTDatabase(void); 00070 00071 virtual bool isOnDataReadAvailable() const { 00072 return true; 00073 } 00074 00075 virtual ble_error_t reset(void); 00076 00077 /* BlueNRG Functions */ 00078 void eventCallback(void); 00079 //void hwCallback(void *pckt); 00080 ble_error_t Read_Request_CB(uint16_t attributeHandle); 00081 GattCharacteristic* getCharacteristicFromHandle(uint16_t charHandle); 00082 void HCIDataWrittenEvent(const GattWriteCallbackParams *params); 00083 void HCIDataReadEvent(const GattReadCallbackParams *params); 00084 void HCIEvent(GattServerEvents::gattEvent_e type, uint16_t charHandle); 00085 void HCIDataSentEvent(unsigned count); 00086 00087 private: 00088 static const int MAX_SERVICE_COUNT = 10; 00089 uint8_t serviceCount; 00090 uint8_t characteristicCount; 00091 uint16_t servHandle, charHandle; 00092 00093 std::map<uint16_t, uint16_t> bleCharHandleMap; // 1st argument is characteristic, 2nd argument is service 00094 GattCharacteristic *p_characteristics[BLE_TOTAL_CHARACTERISTICS]; 00095 uint16_t bleCharacteristicHandles[BLE_TOTAL_CHARACTERISTICS]; 00096 00097 BlueNRGGattServer() { 00098 serviceCount = 0; 00099 characteristicCount = 0; 00100 }; 00101 00102 BlueNRGGattServer(BlueNRGGattServer const &); 00103 void operator=(BlueNRGGattServer const &); 00104 00105 static const int CHAR_DESC_TYPE_16_BIT=0x01; 00106 static const int CHAR_DESC_TYPE_128_BIT=0x02; 00107 static const int CHAR_DESC_SECURITY_PERMISSION=0x00; 00108 static const int CHAR_DESC_ACCESS_PERMISSION=0x03; 00109 static const int CHAR_ATTRIBUTE_LEN_IS_FIXED=0x00; 00110 }; 00111 00112 #endif
Generated on Thu Jul 14 2022 01:08:08 by
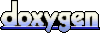