
NRF52_esb
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut myled(LED1); 00004 00005 #include <stdbool.h> 00006 #include <stdint.h> 00007 #include <string.h> 00008 //#include "nrf.h" 00009 #include "micro_esb.h" 00010 #include "uesb_error_codes.h" 00011 #include "nrf_delay.h" 00012 //#include "nrf_gpio.h" 00013 00014 00015 00016 static uesb_payload_t tx_payload, rx_payload; 00017 00018 void uesb_event_handler() 00019 { 00020 static uint32_t rf_interrupts; 00021 static uint32_t tx_attempts; 00022 00023 uesb_get_clear_interrupts(&rf_interrupts); 00024 00025 if(rf_interrupts & UESB_INT_TX_SUCCESS_MSK) 00026 { 00027 } 00028 00029 if(rf_interrupts & UESB_INT_TX_FAILED_MSK) 00030 { 00031 uesb_flush_tx(); 00032 } 00033 00034 if(rf_interrupts & UESB_INT_RX_DR_MSK) 00035 { 00036 uesb_read_rx_payload(&rx_payload); 00037 NRF_GPIO->OUTCLR = 0xFUL << 8; 00038 NRF_GPIO->OUTSET = (uint32_t)((rx_payload.data[2] & 0x0F) << 8); 00039 } 00040 00041 uesb_get_tx_attempts(&tx_attempts); 00042 NRF_GPIO->OUTCLR = 0xFUL << 12; 00043 NRF_GPIO->OUTSET = (tx_attempts & 0x0F) << 12; 00044 } 00045 00046 int main(void) 00047 { 00048 uint8_t rx_addr_p0[] = {0x12, 0x34, 0x56, 0x78, 0x9A}; 00049 uint8_t rx_addr_p1[] = {0xBC, 0xDE, 0xF0, 0x12, 0x23}; 00050 uint8_t rx_addr_p2 = 0x66; 00051 00052 //nrf_gpio_range_cfg_output(8, 15); 00053 00054 NRF_CLOCK->EVENTS_HFCLKSTARTED = 0; 00055 NRF_CLOCK->TASKS_HFCLKSTART = 1; 00056 while(NRF_CLOCK->EVENTS_HFCLKSTARTED == 0); 00057 00058 uesb_config_t uesb_config = UESB_DEFAULT_CONFIG; 00059 uesb_config.rf_channel = 5; 00060 uesb_config.crc = UESB_CRC_16BIT; 00061 uesb_config.retransmit_count = 6; 00062 uesb_config.retransmit_delay = 500; 00063 uesb_config.dynamic_ack_enabled = 0; 00064 uesb_config.protocol = UESB_PROTOCOL_ESB_DPL; 00065 uesb_config.bitrate = UESB_BITRATE_2MBPS; 00066 uesb_config.event_handler = uesb_event_handler; 00067 00068 uesb_init(&uesb_config); 00069 00070 nrf_esb_set_base_address_0(rx_addr_p0); 00071 nrf_esb_set_base_address_1(rx_addr_p1); 00072 //nrf_esb_set_base_address_2(&rx_addr_p2); 00073 00074 tx_payload.length = 8; 00075 tx_payload.pipe = 0; 00076 tx_payload.data[0] = 0x01; 00077 tx_payload.data[1] = 0x00; 00078 tx_payload.data[2] = 0x00; 00079 tx_payload.data[3] = 0x00; 00080 tx_payload.data[4] = 0x11; 00081 00082 while (true) 00083 { 00084 if(uesb_write_tx_payload(&tx_payload) == UESB_SUCCESS) 00085 { 00086 tx_payload.data[1]++; 00087 } 00088 nrf_delay_us(10000); 00089 } 00090 } 00091
Generated on Sun Jul 17 2022 00:47:52 by
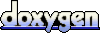