
Simple Pong game on NokiaLCD with PS2
Dependencies: mbed PS2 NokiaLCD
paddle.cpp
00001 #include "mbed.h" 00002 #include "paddle.h" 00003 00004 Paddle::Paddle() { 00005 int x=y=width=height=color=score=0; 00006 lives = 3; 00007 } 00008 00009 Paddle::Paddle(int x, int y, int w, int h, int c, int l, int s) 00010 : x(x), y(y), width(w), height(h), color(c), lives(l), score(s) {} 00011 00012 /* 00013 * Member Function move: 00014 * Description: Colors in the previous paddle black 00015 * and moves paddle to new position. 00016 */ 00017 void Paddle::move(NokiaLCD &lcd, int increment) { 00018 draw(lcd, true); 00019 y += increment; 00020 } 00021 00022 00023 /* 00024 * Member Function moveCPU: 00025 * Description: Colors in the previous paddle black 00026 * and moves paddle to new position. 00027 * inc variable allows paddle to only move every 00028 * other function call. 00029 */ 00030 void Paddle::moveCPU(NokiaLCD &lcd, int _y) { 00031 static int inc = 1; 00032 draw(lcd, true); 00033 if(_y>y+height/2 && y+height<130) y += inc; 00034 else if(_y+5<y+height/2 && y>0) y -= inc; 00035 inc = (inc) ? 0 : 1; 00036 } 00037 00038 void Paddle::draw(NokiaLCD &lcd, bool isBlack) const { 00039 lcd.fill(x, y, width, height, (isBlack) ? 0x000000 : color); 00040 } 00041 00042 bool Paddle::loseLife() { 00043 return --lives; 00044 } 00045 00046 void Paddle::addPoint() { 00047 ++score; 00048 } 00049 00050 int Paddle::size() const { 00051 return width*height; 00052 } 00053 00054 int Paddle::getWidth() const { 00055 return width; 00056 } 00057 00058 int Paddle::getHeight() const { 00059 return height; 00060 } 00061 00062 int Paddle::getX() const { 00063 return x; 00064 } 00065 00066 int Paddle::getY() const { 00067 return y; 00068 } 00069 00070 int Paddle::getLives() const { 00071 return lives; 00072 } 00073 00074 int Paddle::getScore() const { 00075 return score; 00076 } 00077 00078 void Paddle::setLives(int l) { 00079 lives = l; 00080 }
Generated on Tue Jul 12 2022 18:11:13 by
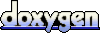